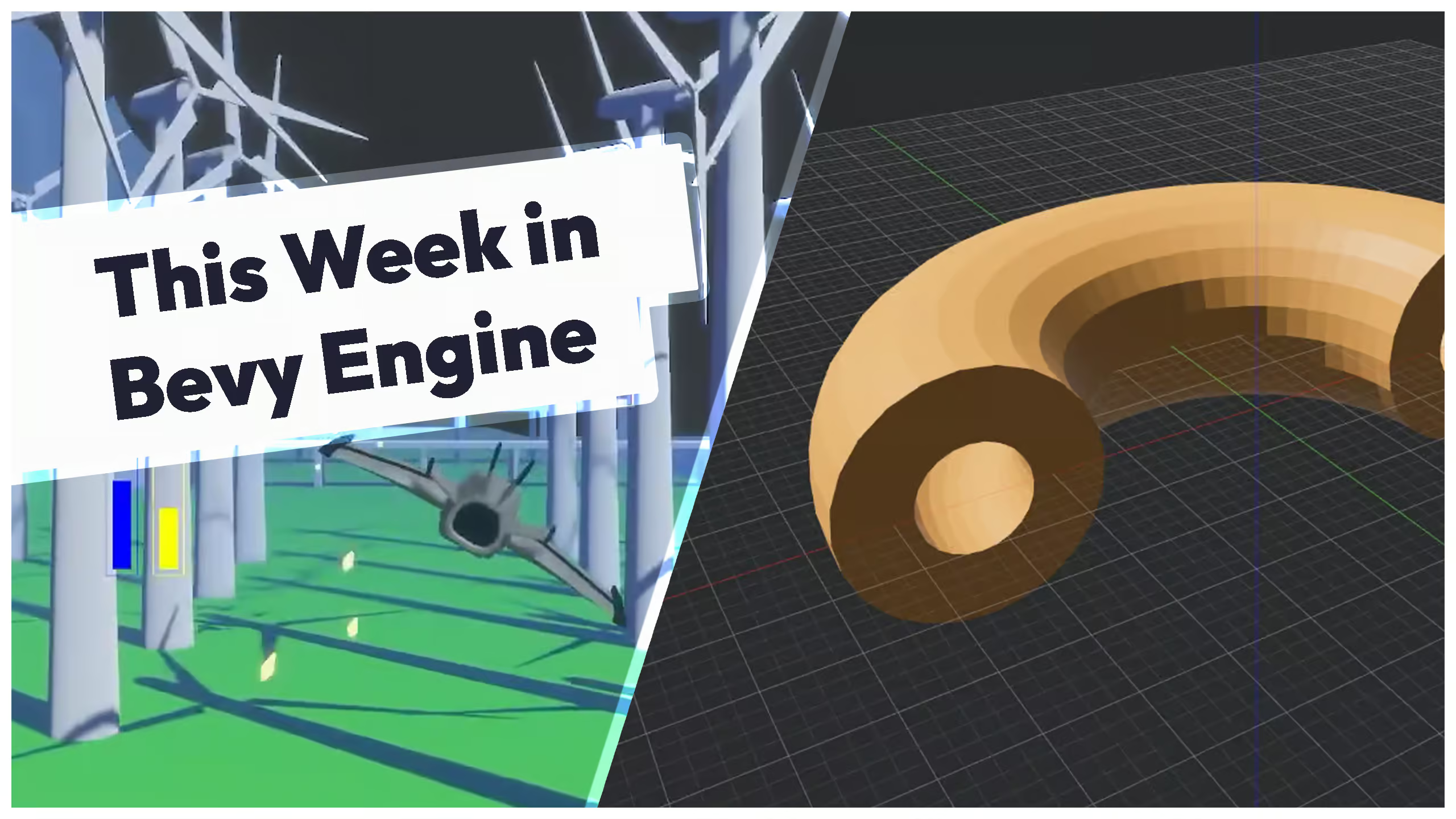
WESL, Recursive run_system, and Transform Propagation Optimization
2025-03-17
This week in Bevy we see experimental WESL support land! This is an exciting, very early look into some community driven extensions to WGSL.
The transform propagation system gets a nice optimization for static scenes, run_system becomes recursively callable, and the Bevy Remote Protocol takes steps towards OpenRPC support.
We also see a visual history of the development of Settletopia, 3d g-force hud, and Curveball (a curve generating tool for Neverball level developers).
Experimental WESL support
WESL is a community-driven portable superset of WGSL and the pre-MVP 0.1.x has been released! It intends to add features like imports, conditional compilation, packaging, and more to WGSL, which aligns with how Bevy uses WGSL generally.
#17953 introduces Bevy support for WESL as a shader source, which enables loading .wesl
shaders.
This is experimental support, so don't expect to mix shaders via naga-oil yet but use this as a chance to check out the new shader_material_wesl
example and some of the new WESL language syntax for imports, etc.
children! in updated examples
A large number of the Bevy examples now take advantage of the new children!
macro that came with the recent improved spawning apis.
computed_states
now uses children!custom_transitions
,sub_states
gamepad_viewer
render_primitives
breakout
states
text_input
andvirtual_time
tests for helpers
Bevy's examples contain some "helper" code, especially when the functionality isn't directly related to the example's purpose but it is still useful to have, like camera controllers in non-camera examples.
#18288 adds a new examples/helpers
directory for "library" style examples. Currently this includes a camera_controller
and ui widgets.
Bevy Remote Protocol: OpenRPC
A first step towards OpenRPC support for the Bevy Remote Protocol. #18068 introduces an rpc.discover
endpoint that follows the spec.
Spawning related entities is a recent addition to the Bevy spawn APIs. For example Children::spawn(...)
. #18259 introduces a more direct way to spawn a Vec
of Bundles
by implementing SpawnableList
. Previously this was achievable through the use of SpawnIter
which required iterating the Vec
.
Children::spawn(vec![
(
Name::new("first element"),
Transform::from_xyz(0., 10., 0.),
),
(
Name::new("second element"),
Transform::from_xyz(10., 10., 0.),
),
]);
Recursive run_system
As of #18076, run_system
will requeue the system's command queue enabling the ability to recursively call run_system
.
#[derive(Resource)]
pub struct Test {
id: SystemId,
counter: u32,
}
let mut world = World::new();
let id = world.register_system(|mut commands: Commands, mut test: ResMut<Test>| {
print!("{:?} ", test.counter);
test.counter -= 1;
if test.counter > 0 {
commands.run_system(test.id);
}
});
world.insert_resource(Test { id, counter: 5 });
world.run_system(id).unwrap();
Saturation trait for bevy_color
#18202 introduces a new set of saturation APIs encapsulated in a Saturation
trait.
fn next_golden(&mut self) -> Color {
self.current
.rotate_hue((1.0 / GOLDEN_RATIO) * 360.0)
.with_saturation(self.rand_saturation())
.with_luminance(self.rand_luminance())
.into()
}
Decoupled naga for wgpu testing
The ability to test upcoming wgpu features in Bevy is an important capability that is made easier this week through #18099, which decouples some of the rendering internals allowing the testing of newer wgpu versions.
Transform propagation optimization
Another speed boost in Transform propagation lands this week with #18093 optimizing the algorithm to mark static subtrees. This means scenes with largely static Transforms see a big speedup.
System Config Genericization
Following a great writeup, #17962 reduces duplication between IntoSystemConfigs
and IntoSystemSetConfigs
, potentially setting the groundwork for issue #14195: "Support .run_if() on observers"
Alice's Merge Train is a maintainer-level view into active PRs and how the Bevy sausage is made.
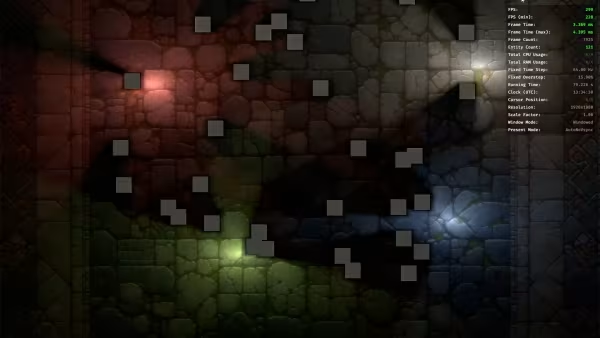
Showcase
Bevy work from the #showcase channel in Discord and around the internet. Use hashtag #bevyengine.
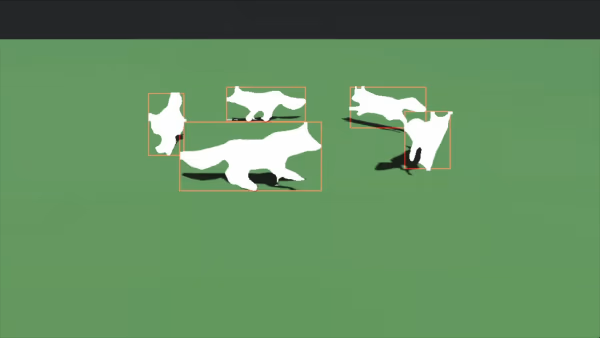
vulkan-cuda interop
showcase
This demo is running as a node in the render graph using an image segmentation model running in pytorch via pyo3. the main render texture is exported from vulkan via wgpu-hal to cuda and then exposed as a cuda array to torch in a python script.
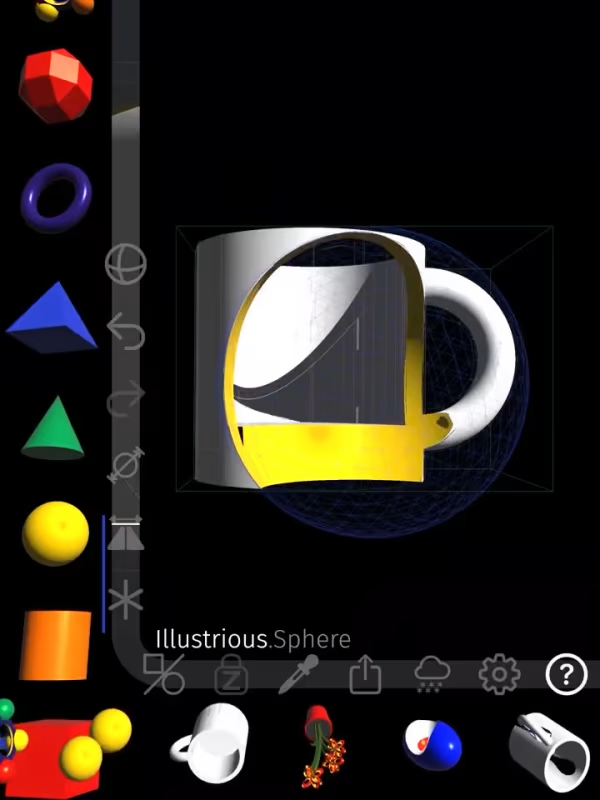
Hybrid raytracing shader
showcase
Using shadybug for development, this hybrid raytracing shader is ready for GDC and working on an iPad.
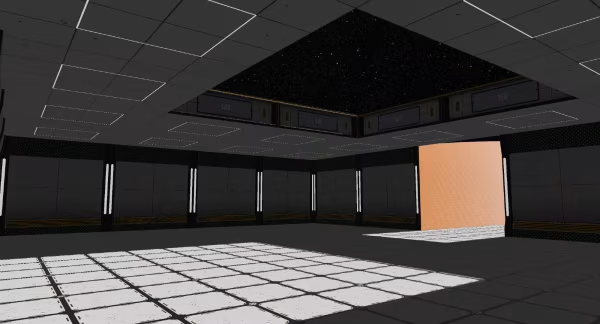
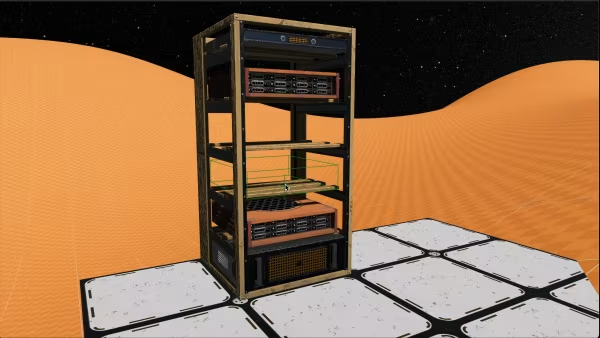
Computronium
showcase
Computronium is an automation game like Factorio or Satisfactory with an emphasis on computer hardware and networks.
This week:
- Walls can now be placed in the world and snap to foundations
- Servers can now be added to and removed from rack slots
- A new sprint ability was added
- The first music track was composed
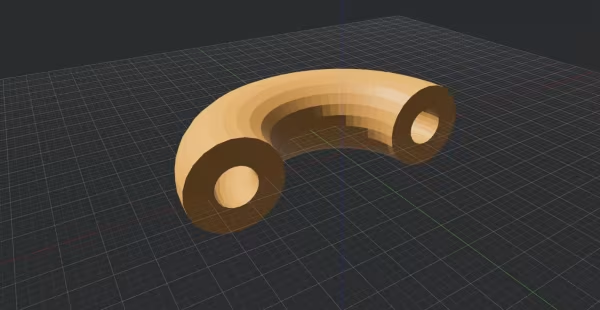
Curveball
showcase
Curveball is a curve generating tool for Neverball level developers. You can use it on the web and check out the git repo
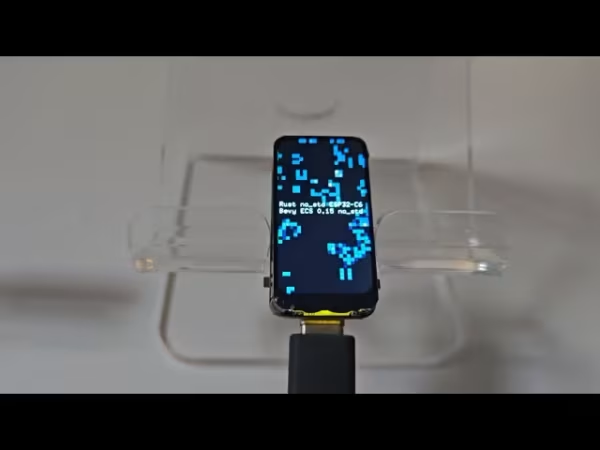
Conways Game of Life: esp32-c6
showcase
Conway's Game of Life Bare Metal demo using Bevy no_std + esp-hal 1.0.0. It runs on 1.47 inch ESP32-C6 Waveshare display, with decent framerate, including DMA and framebuffer
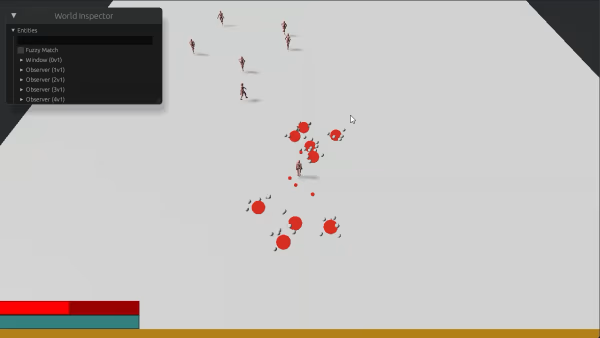
Composable Ability System
showcase
A composable ability system. Items are entities with "affixes" which can be equipped by another entity. The item can then "pass on" effects to the equipping entity.
(
affix_type: "Test",
tags: [],
level_requirement: 1,
stats: {
"MeditateLevels": 1.0,
},
grants: {
"IncreasedLife": 25.0,
},
requires: ([]),
weight: 0.0,
children: [
(
stats: {
"ExplosionEffect": {
"radius": "root.AddedLife / 10",
"damage": {
"min": 10.0,
"max": 50.0,
},
},
"MeditateIntervalCue": 1.0,
"TargetThis": 1.0
},
requires: ([]),
),
],
)
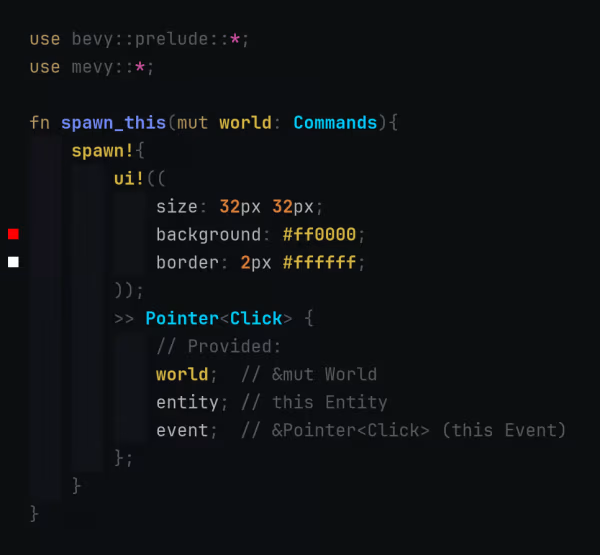
Mevy Macros
showcase
New macro additions to mevy, a collection of bevy macros
>>
observes an event and queues a closure command..
means a short syntax for 'on event' World mutation- using
>
will only provide EntityCommands and the Event
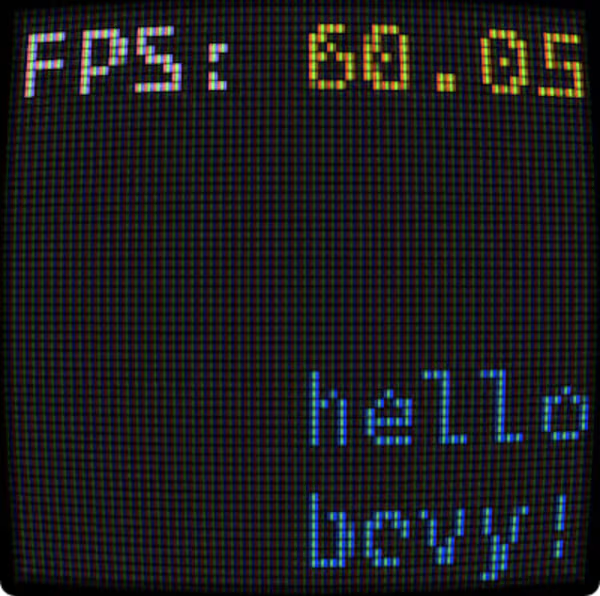
bevy_old_tv_shader 2d camera support
showcase
2d camera support for an old tv style shader that can optionally apply the bevy_old_tv_shader effect to UI and text as well
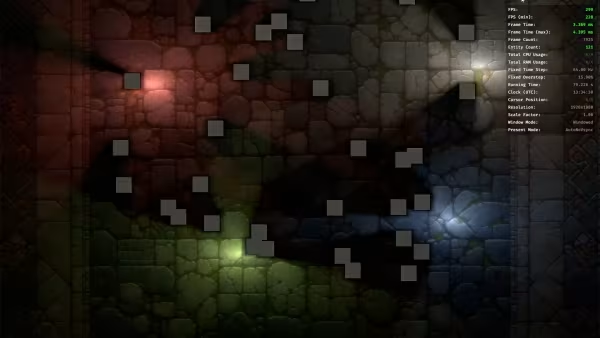
Crates
New releases to crates.io and substantial updates to existing projects
bevy_radix_sort
crate_release
bevy_radix_sort is a low-level GPU-based radix sort implementation that's optimized for sorting u32 key/value pairs.
If you're interested in sorting algorithms like this one, check out the state of the art in GPU sorting including a wgsl implementation of OneSweep
bevy_ecs_tiled v0.6.0
crate_release
bevy_ecs_tiled is a Bevy plugin for working with 2D tilemaps created with the Tiled map editor. It relies upon the official Tiled Rust bindings to parse and load Tiled map files and the bevy_ecs_tilemap crate to perform rendering. bevy_ecs_tiled aims to provide a simple and ergonomic workflow by using Tiled as an editor when working on Bevy 2D games.
0.6 brings:
- Support for Tiled world
- Aggregate physics colliders from tiles to improve global performances
- Better support for infinite maps
- Rework loading events to use both entity-scoped observers and regular events
bevy_save
crate_release
bevy_save enables you to easily save and load your entire application state, including resources.
Bevy Replicon 0.31.0
crate_release
bevy_replicon is a crate for server-authoritative networking.
0.31 brings
- Connected clients are now represented as entities.
- Switch from bincode to postcard
As a result of preparing for a talk on the crate at Bevy Meetup #9, the quickstart guide was rewritten as well. The quickstart guide now contains details on how to write a messaging backend or implement client-side prediction.
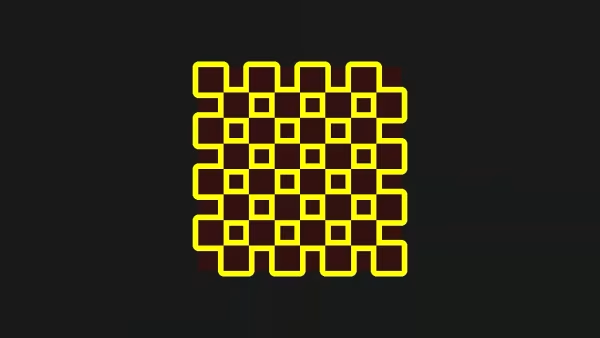
bevy_mod_outline 0.9.1
crate_release
bevy_mod_outline is a mesh outlining crate that supports both the vertex extrusion and jump-flood methods
0.9.1 brings:
- New double-sided outlining modes for non-manifold geometry.
- Support for outlining shapes defined using the alpha channel of a texture
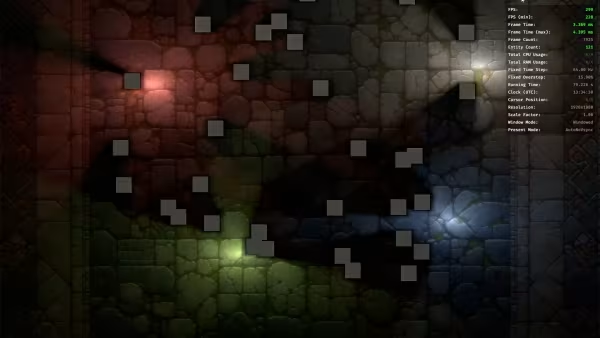
Devlogs
vlog style updates from long-term projects
Programming isn't enough
devlog
A devlog look into a year-long development effort for a digital, competitive card game. The devlog topic is how focusing on programming isn't enough for a commercial game.
The Development History of Settletopia
devlog
A largely visual history of the development of Settletopia, which can now be wishlisted on Steam
Transform Propagation Optimization: Static Subtree Marking authored by aevyrie
Add support for experimental WESL shader source authored by tychedelia
Remove lifetime from `QueryEntityError` authored by bushrat011899
Partially fix panics when setting `WGPU_SETTINGS_PRIO=webgl2` authored by greeble-dev
Ignore unmaintained security advisory about paste for now authored by alice-i-cecile
Improve `Segment2d`/`Segment3d` API and docs authored by Jondolf
action shouldn't comment on a pr if last comment is from action authored by mockersf
Decoupled naga for wgpu testing authored by JMS55
`UiTargetCamera` doc comment correction authored by ickshonpe
Fix CI comment workflow after 18196 authored by mockersf
bevy_reflect: bump petgraph dependency authored by flokli
Address `Entities::len` inconsistency authored by ElliottjPierce
Add Saturation trait to bevy_color authored by boondocklabs
docs: Fix incorrect cycle_modes function documentation authored by Emmet-v15
Add more to the 2d testbed for text authored by rparrett
Expand the `RelationshipSourceCollection` to return more information authored by Brezak
Fix mikkitspace typos authored by BenjaminBrienen
(Adoped) Remove panics and optimise mesh picking authored by BD103
`extract_text_shadows` camera query fix authored by ickshonpe
Parallelize prepare_assets::<T> systems authored by brianreavis
Replace winit's synthetic events by our own key tracking authored by Azorlogh
Backtrace: std and threadsafe bevy_error_panic_hook authored by SpecificProtagonist
don't use bevy_pbr for base bevy_gizmos plugin authored by rambip
Respect viewport position in coordinate conversion functions authored by Novakasa
Improve component registration performance authored by ElliottjPierce
`many-cameras` option for `many_buttons` authored by ickshonpe
Fix unsound lifetimes in `Query::join` and `Query::join_filtered` authored by chescock
Use register_dynamic for merging authored by ElliottjPierce
Reimplement bindless storage buffers. authored by pcwalton
Recursive run_system authored by SpecificProtagonist
Queued component registration authored by ElliottjPierce
Make buffer binding arrays emit bind group layout entries and bindless resource descriptors again. authored by pcwalton
Rework WindowMode::Fullscreen API authored by jf908
make various entity wrapper type modules public authored by Victoronz
Fix non-crate typos authored by BenjaminBrienen
Remove superfluous spaces in `Transform` documentation authored by Brezak
bevy_reflect: Reflection-based cloning authored by MrGVSV
Add `print_stdout` and `print_stderr` lints (#17446) authored by sirius94
Sync up the Derive of DragEntry to match the other events authored by Peepo-Juice
Implement SpawnableList for Vec<Bundle> authored by krunchington
Update text_input and virtual_time examples to use Improved Spawning API authored by krunchington
Update state/states example to use children! macro authored by krunchington
Internalize BevyManifest logic. Switch to RwLock authored by cart
Add derive Default to Disabled authored by NiseVoid
Upgrade to cosmic-text 0.13 authored by rparrett
Remove the entity index from the UI phase's sort key authored by ickshonpe
Switch to `ImDocument` in `BevyManifest` authored by bushrat011899
Update render_primitives example for children! macro authored by krunchington
Update breakout example's stepping plugin to use children authored by krunchington
Implement Serialize/Deserialize/PartialEq for bounding primitives authored by mweatherley
Generic system config authored by newclarityex
RPC Discover endpoint with basic informations authored by Leinnan
Update computed_states example to use children macro authored by krunchington
Update custom_transitions and sub_states examples to use children macro authored by krunchington
Small cleanup for ECS error handling authored by alice-i-cecile
Add `examples/helpers/*` as library examples authored by bushrat011899
Update ui_test requirement from 0.23.0 to 0.29.1 authored by mnmaita
implement `Bounded2d` for `ConvexPolygon` authored by lynn-lumen
Return an error when direct-nested-loading a subasset authored by andriyDev
Update gamepad_viewer to use children macro authored by krunchington
Fix shadow_biases example authored by JMS55
bevy_reflect: Deprecate `PartialReflect::clone_value` authored by MrGVSV
add Entity default to the entity set wrappers authored by Victoronz
Use 4-byte LightmapSlabIndex for batching instead of 16-byte AssetId<Image> authored by superdump
Fix MainTransparentPass2dNode attachment ordering authored by JMS55
Add missing system ordering constraint to prepare_lights authored by JMS55
Want to contribute to Bevy?
Here you can find two types of potential contribution: Pull Requests that might need review and Issues that might need to be worked on.
Pull Requests Opened this week
Provide a safe abstraction for split access to entities and commands authored by ItsDoot
bevy_reflect: Add `ReflectClone` type data authored by MrGVSV
Re-introduce "Replace Ambient Lights with Environment Map Lights (#17482)" authored by SparkyPotato
move utilities from bevy_reflect/derive to bevy_macro_utils. authored by Bleachfuel
Fix surface validation errors in WSL2 environments during window resizing authored by tyler274
Use `ThinColumn` in `ComponentSparseSet` authored by ecoskey
Incorporate viewport position into clamping in `camera_system` authored by mweatherley
Let `FilteredEntity(Ref|Mut)` receive access when nested. authored by chescock
Restore separate methods for `World::get_many_entities` authored by chescock
FilteredResource returns a Result instead of a simple Option authored by andristarr
Remote entity reservation v2 authored by ElliottjPierce
Bone attachments authored by hukasu
public function activeanimation update authored by MushineLament
Generalize `DiGraph`/`UnGraph` 📊 authored by ItsDoot
Update shader_prepass, testbed_2d, and first_person_view_model examples to use children! macro authored by krunchington
Add process cpu/memory usage to SystemInformationDiagnosticsPlugin authored by tjlaxs
Update accesskit and accesskit_winit requirements authored by mnmaita
Update getrandom requirement from 0.2.0 to 0.3.1 authored by mnmaita
Update log_layers_ecs example for children macro authored by krunchington
Fix nested immediate asset loading. authored by andriyDev
Shrink from_reflect_with_fallback authored by SpecificProtagonist
Fixed Reflect derive macro on empty enums (#18277) authored by Wuketuke
Create new `NonSendMarker` authored by joshua-holmes
bevy_reflect: Add clone registrations project-wide authored by MrGVSV
update superlinter authored by mockersf
Update sprite_slice, spatial_audio_3d, spatial_audio_2d examples to use children macro authored by krunchington
Update text2d example to use children macro authored by krunchington
implement get_many_unique authored by Victoronz
Prefer `core::time::Duration` over `std::time::Duration` authored by mnmaita
Derive Macro for MapEntites (#17611) authored by Wuketuke
Required components accept const values (#16720) authored by Wuketuke
Add `no_std` Library Example authored by bushrat011899
Record bloom render commands in parallel authored by JMS55
Update testbed_ui to use Improved Spawning API authored by krunchington
Revert "don't use bevy_pbr for base bevy_gizmos plugin" authored by mockersf
Move non-generic parts of the PrepassPipeline to internal field authored by IceSentry
Remove system set indirection on `TransformPlugin` authored by hukasu
Add bevy_anti_aliasing authored by IceSentry
Issues Opened this week
`World::register_required_components` can make bundles outdated authored by urben1680
check-examples-on-wasm CI job keeps timing out stochastically authored by alice-i-cecile
Bevy only able to use software rendering in Fedora 41 authored by lmariscal
Text with an invalid span is silently not rendered authored by benfrankel
Add `Assets::map_unchanged` or `get_mut_untracked` or similar authored by Waridley
Can't take transparent screenshots authored by amytimed
`EntityHashSet` can't be used as a relationship target collection because it doesn't implement `VisitEntitiesMut` authored by Brezak
Migrate examples to the improved Spawning API (to `children! from `with_children`) authored by krunchington
Screenshots use previous camera position authored by amytimed
`mesh_picking` not updating when mesh data changes, bug or intended? authored by ncorbin786
Transform propagation ignoring earlier branches authored by ickshonpe
Ability to trace bevy systems when running `wasm32-unknown-unknown` builds in the browser authored by mitchmindtree
Mac M1+WASM: `many_buttons` extreme memory usage authored by Azorlogh
Use `Name` component in warnings and errors regarding entities authored by morgenthum
Add label and CI task to catch and document direct dependency changes authored by bushrat011899
Add additional benchmarks to `picking::ray_mesh_intersection` authored by BD103
world_to_ndc returns inf that triggers panic in bevy_ui layout authored by tbillington
Using mimalloc with bevy reveals unsoundness. authored by moonheart08
Gltf assets do not reload correctly authored by thepackett
Add a lax version of `RelationshipTarget` as a super trait. authored by mintlu8
Allow readonly queries and system parameters to be initialized with only `&World`. authored by ElliottjPierce
Revisit Queued Component Registration code quality after Component as Entities authored by ElliottjPierce
Reflect derive is broken on main for empty enums authored by eugineerd
`RelationshipInsertHookMode` for `remove` and `take` authored by Brezak
Nested subasset loads tries to return the root nested asset. authored by andriyDev
(Android) rotation mobile example black screen and then crash authored by insertokname
Ergonomics of re-using run conditions authored by cBournhonesque
Being able to visualize the exact schedule order being used authored by cBournhonesque
Consider using add_command_buffer_generation_task() for MainTransparentPass3dNode and MainTransmissivePass3dNode authored by JMS55
Consider using add_command_buffer_generation_task() for bloom authored by JMS55
1-frame rendering flashes when changing the scene authored by JMS55
Example 3d_viewport_to_world: gizmo is displayed in the wrong position authored by ElDiddi