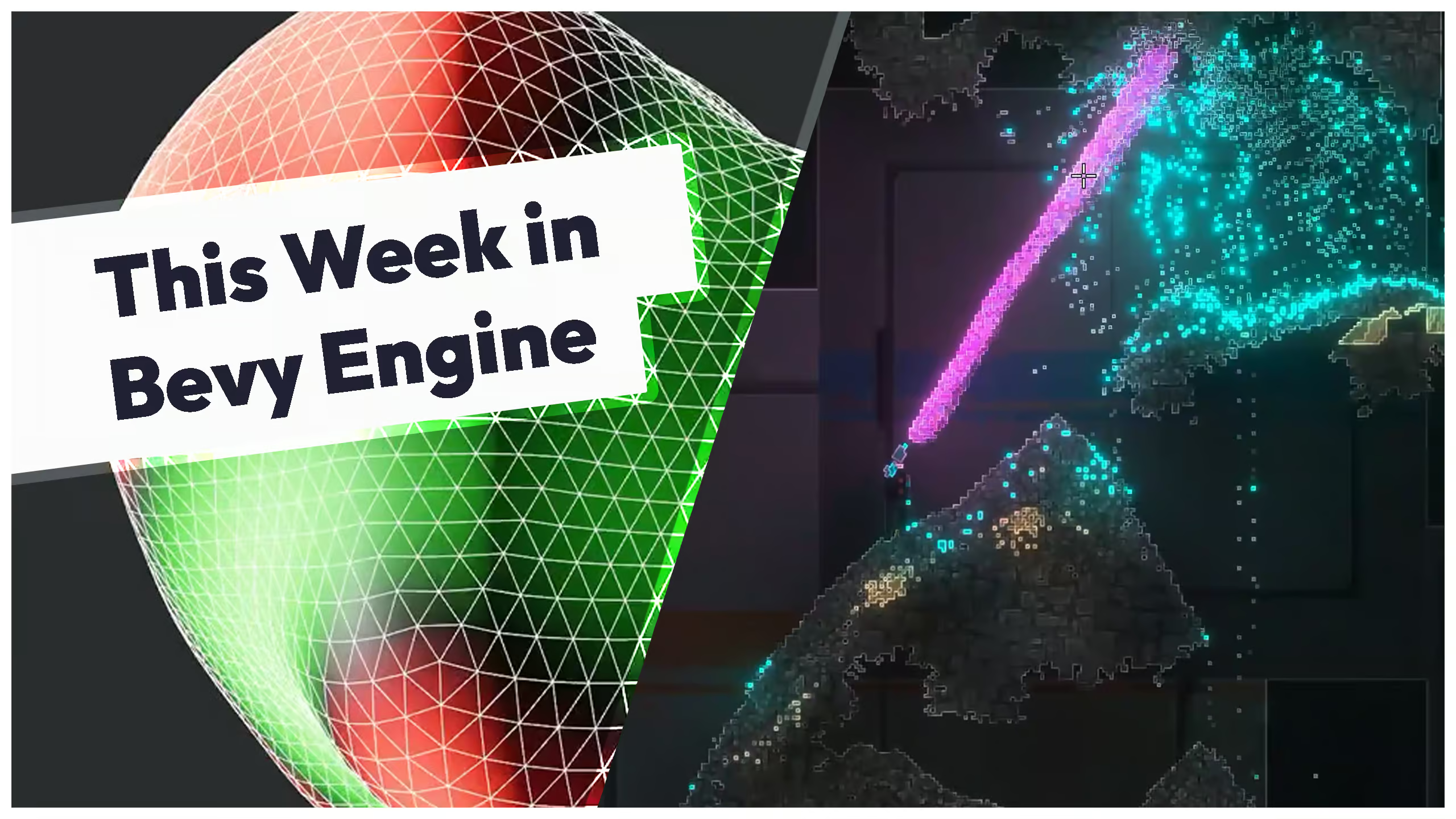
Better Errors, Settletopia, and One-to-One Relationships
2025-03-10
This week Bevy gains a new catch-all error type: BevyError
which gets built into the new Bevy Result
, and more Bevy APIs get Result
return values.
In the showcase we see a few tower defense games and a number of submissions to the Code for a Cause Jam.
Settletopia, the multiplayer open-world fantasy colony sim, also lands its Steam page.
Error Handling
In 0.16 Bevy is moving towards more Result
oriented error handling for systems, observers, and more.
#18144 introduces a brand new catch-all BevyError
which improves error reporting in a similar way to crates like anyhow behave. This has been integrated into Bevy's Result
type alias making the following possible:
fn oh_no() -> Result {
let number: usize = "hi".parse()?;
println!("parsed {number}");
Ok(())
}
#18082 additionally brings a Result
return type to Query::single
and Query::single_mut
. The old get_single
functions are now deprecated.
One-to-One Relationships
One-to-Many Relationships already made it into 0.16, and #18087 brings directed One-to-One Relationships.
#[derive(Component)]
#[relationship(relationship_target = Below)]
pub struct Above(Entity);
#[derive(Component)]
#[relationship_target(relationship = Above)]
pub struct Below(Entity);
One important note is that this is an asymmetrical Relationship. That means one component in the Relationship is the source of truth. That's Above
in the example. Adding Above
to an Entity
causes Below
to be added to the other Entity
automatically, but adding Below
does not cause Above
to be added.
Alice's Merge Train is a maintainer-level view into active PRs and how the Bevy sausage is made.
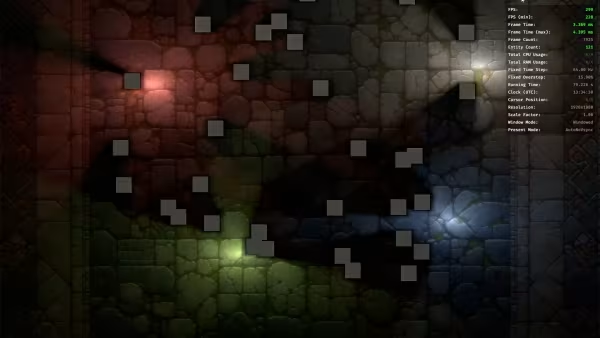
Showcase
Bevy work from the #showcase channel in Discord and around the internet. Use hashtag #bevyengine.
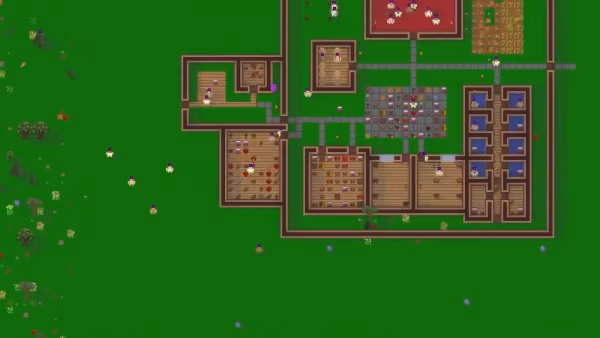
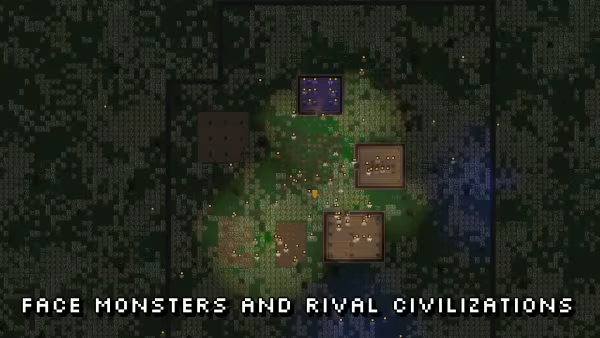
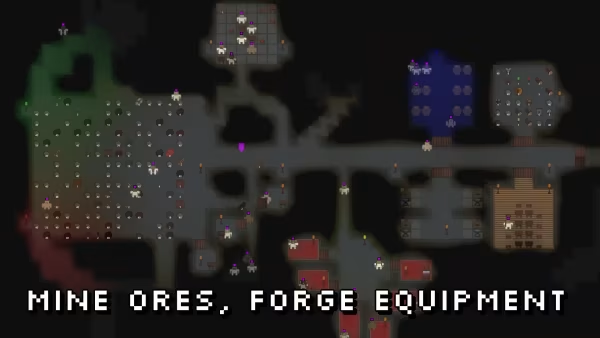
Settletopia
showcase
Settletopia, the multiplayer open-world fantasy colony sim now has a Steam page and a new showcase video! Wishlist now to develop a small settlement into a thriving multi-settlement civilization that spans the world
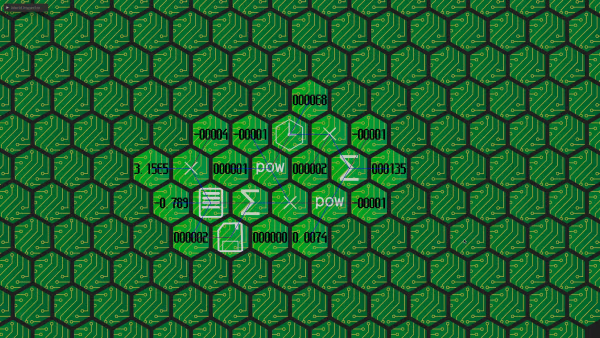
Tower Defense Pi
showcase
The base mechanics for a tower defense style game where you "program" the towers yourself. The numeric calculation bits were programmed first, resulting in the ability to calculate pi.
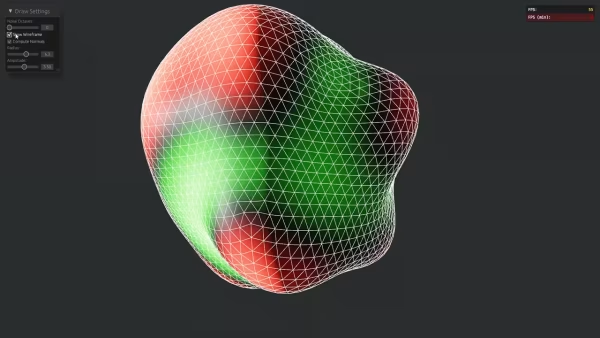
Simplex Noise and Smooth Normals
showcase
Generating planets with simplex noise and smooth normals. It works for any planet radius, noise amplitude and noise octave, and is now almost ready to be dropped into the full-size planet.
bevy_egui, iyes_perf_ui, and bevy_editor_cam are used to build the demo.
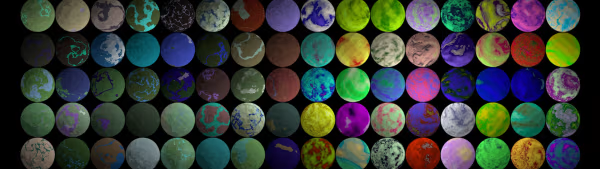
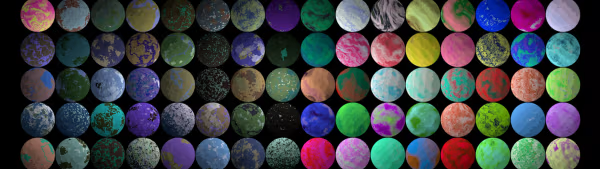
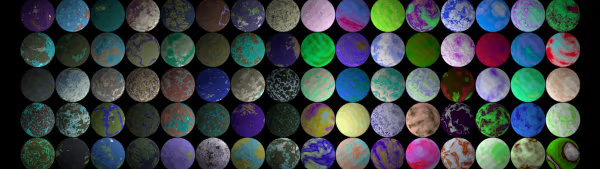
Pixel Planets
showcase
This is a set of Pixel Planets rendered into a video; next up is procedurally generating solar systems.
If you want to generate your own pixel planets, check out Pixel Planet Generator (github) or a Bevy port like this one
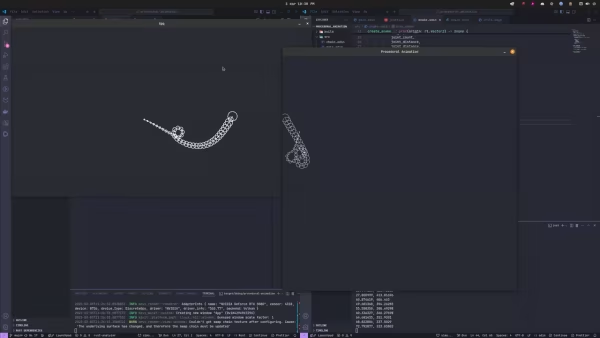
Procedural Snake
showcase
Procedural animation of a snake based on this YouTube video in two languages: Rust/Bevy and Odin/raylib
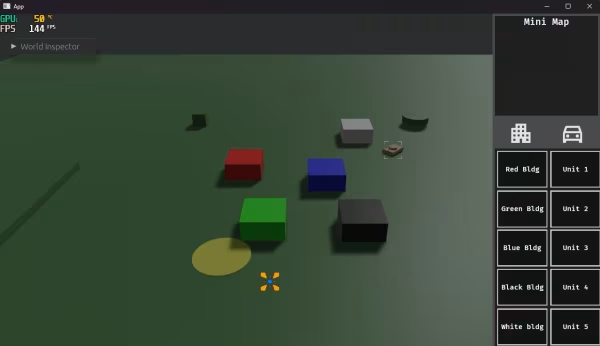
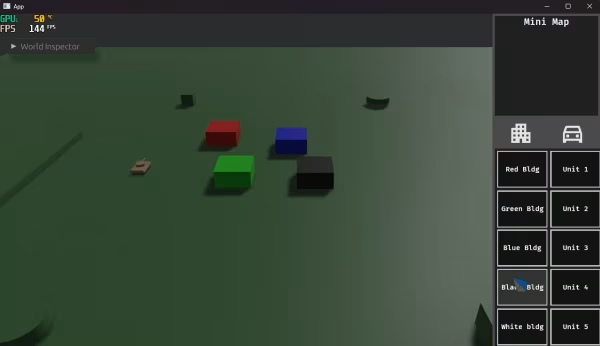
RTS base building
showcase
A look at base building mechanics to an RTS game. The game uses dynamic flowfields that update any time an object is spawned. A preview of the flowfield logic is available here.
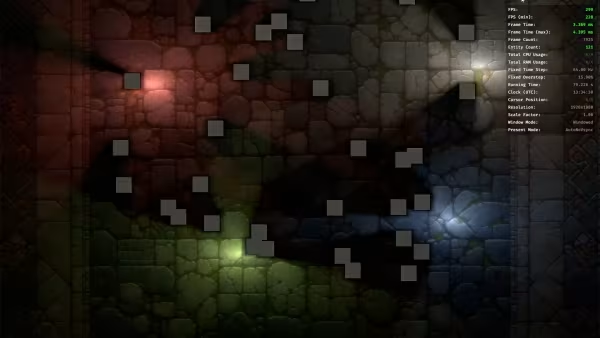
Crates
New releases to crates.io and substantial updates to existing projects
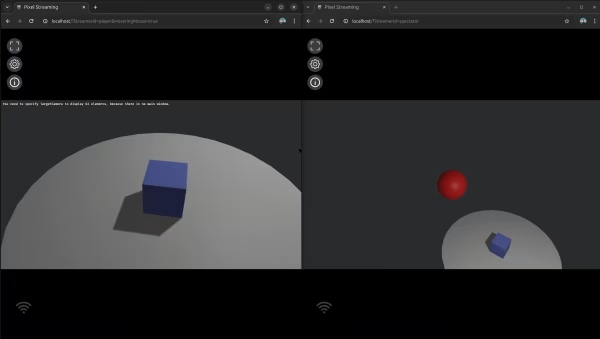
bevy_streaming
crate_release
Stream Bevy's camera to a streaming server (through WebRTC) with ultra-low latency, and play the game through a simple browser or phone: aka "Cloud Gaming"
bevy_mod_async
crate_release
bevy_mod_async is an attempt at a more ergonomic async abstraction for Bevy, built on Bevy's executor. The entire crate is built around TaskContext
, which is the primary API for interacting with the ECS in an asynchronous manner.
commands.spawn_task(|cx| async move {
let some_entity = cx
.with_world(|world| {
world.spawn_empty().id()
})
.await;
let mut events = cx.event_stream::<MyEvent>();
let MyEvent = events.next().await;
cx.sleep(Duration::from_secs(1)).await;
cx.with_world(move |world| {
world.entity_mut(some_entity).despawn();
})
.await;
cx.with_world(|world| {
world.insert_resource(ClearColor(Color::WHITE));
})
.detach();
});
seldom_state
crate_release
seldom_state
is a plugin that adds a StateMachine
component that you can add to your entities. The state machine will change the entity's components based on states, triggers, and transitions that you define. It's useful for AI, animations, rigid player controllers, etc.
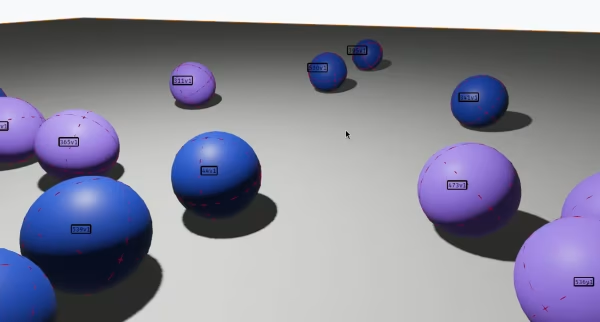
bevy_ui_anchor 0.5
crate_release
bevy_ui_anchor supports anchoring UI elements to specific points or entities in the world.
beet_flow 0.0.5
crate_release
beet_flow is scenes-as-control-flow, use that same scene graph we all know and love to define modular behaviors.
world.spawn((
Name::new("My Behavior"),
Sequence
))
.with_child((
Name::new("Hello"),
ReturnWith(RunResult::Success),
))
.with_child((
Name::new("World"),
ReturnWith(RunResult::Success),
))
.trigger(OnRun::local());
bevy_mod_openxr
crate_release
Bevy support for openxr. xr here stands for eXtended Reality, such as Augmented Reality (AR) or Virtual Reality (VR)
Fix `webgl2` feature gating affecting webgpu in `transmission` example authored by rparrett
Fix label name for Deliberate-Rendering-Change CI authored by alice-i-cecile
Make Query::single (and friends) return a Result authored by alice-i-cecile
Fix incorrect doc about `GamepadAxis::RightZ`/`LeftZ` authored by Zoomulator
Bump `typos` to 1.30.0 authored by rparrett
Make asset watcher work when path contains "../" authored by Threadzless
Fix invisible window creation on Windows authored by aloucks
Add missing bindless imports in `pbr_prepass.wgsl`. authored by pcwalton
Update ruzstd requirement from 0.7.0 to 0.8.0 authored by mnmaita
`Val::resolve` doc comment fix authored by ickshonpe
fix generics for relationships authored by Bleachfuel
Reextract meshes when their material assets change. authored by pcwalton
fix test for .iter().sort() to include data to sort authored by Nathan-Fenner
Fix panic with multiple fog volumes authored by greeble-dev
Emphasize no structural changes in SystemParam::get_param authored by GlennFolker
Fix unsound query transmutes on queries obtained from `Query::as_readonly()` authored by chescock
testbed for UI authored by mockersf
Remove deprecated `component_reads_and_writes` authored by bushrat011899
Relationship(…Target) html trait tag authored by SpecificProtagonist
Cache systems by `S` instead of `S::System` authored by benfrankel
Replace some `!Send` resources with `thread_local!` authored by joshua-holmes
One-to-One Relationships authored by dmyyy
Replace Ambient Lights with Environment Map Lights authored by SparkyPotato
Update ureq requirement from 2.10.1 to 3.0.8 authored by mnmaita
`BorderRadius` comment fix authored by ickshonpe
Revert "Replace Ambient Lights with Environment Map Lights (#17482)" authored by mockersf
Preserve spawned RelationshipTarget order and other improvements authored by cart
Fix Component require() IDE integration authored by cart
Replace internal uses of `insert_or_spawn_batch` authored by JaySpruce
Helper function for getting inverse model matrix in WGSL shaders authored by jakkos-net
Deprecate `insert_or_spawn` function family authored by ElliottjPierce
allow `Call` and `Closure` expressions in hook macro attributes authored by RobWalt
Stop automatically generating meta files for assets while using asset processing. authored by andriyDev
Prevent an additional world update after all windows have closed on Windows authored by aloucks
Update Component docs to point to Relationship trait authored by krunchington
Support for non-browser `wasm` authored by bushrat011899
Fix mesh tangent attribute matching in mesh transform operations authored by aloucks
Add `no_std` support to `bevy` authored by bushrat011899
Improve derive(Event) and simplify macro code authored by Bleachfuel
Deprecated Query::many and many_mut authored by alice-i-cecile
BevyError: Bevy's new catch-all error type authored by cart
Add `TextureAtlasSources::uv_rect` to get `Rect` in UV coords authored by copygirl
rendering regression: mention in pr comment that the solution could be to update the pr authored by mockersf
Ensure `bevy_utils` is included with `std` feature authored by bushrat011899
Add byte information to `PositionedGlyph` authored by bytemunch
Want to contribute to Bevy?
Here you can find two types of potential contribution: Pull Requests that might need review and Issues that might need to be worked on.
Pull Requests Opened this week
Partially fix panics when setting `WGPU_SETTINGS_PRIO=webgl2` authored by greeble-dev
Add `on_insert` hook to `Node` to warn if parent has `Text` authored by bytemunch
Remove `insert_or_spawn` function family authored by ElliottjPierce
UI Node Gradients authored by ickshonpe
wrap EntityIndexMap/Set slices as well authored by Victoronz
Pad out binding arrays to their full length if partially bound binding arrays aren't supported. authored by pcwalton
Make buffer binding arrays emit bind group layout entries and bindless resource descriptors again. authored by pcwalton
Add `LeafNode` to forbid adding `Node` children to certain `Node`s authored by bytemunch
Add a `scale_factor` parameter to `Val::resolve` authored by ickshonpe
Consolidate together Bevy's TaskPools [adopted] authored by hymm
Combine `Query` and `QueryLens` using a type parameter for state authored by chescock
Temporary fix to prevent panics on loading Gltf assets in parallel due to stack overlows authored by john-sharratt
Improve component registration performance authored by ElliottjPierce
bevy_reflect: bump petgraph dependency authored by flokli
Queued component registration authored by ElliottjPierce
Rustdoc: Restore visibility of required components authored by SpecificProtagonist
Create cfg aliases for `bevy_app` authored by hukasu
Warn about uses of `RenderLayers` and `UiTargetCamera` authored by hukasu
Async Plugin Construction authored by SpecificProtagonist
Remote entity reservation authored by ElliottjPierce
Add test for mesh transformations authored by greeble-dev
Add Allows filter to bypass DefaultQueryFilters authored by NiseVoid
Split example file docblock and code when generating web examples markdown authored by doup
Address `Entities::len` inconsistency authored by ElliottjPierce
Add the ability for SubApps to have a custom update function authored by moonheart08
Add Saturation trait to bevy_color authored by boondocklabs
Cleaner macros utilizing syn::Member authored by Bleachfuel
Introduce public `World::register_dynamic_bundle` method authored by urben1680
Issues Opened this week
Allow `DynamicEnum` to not have a set variant authored by villor
Support Many-to-Many relationships authored by ItsDoot
Component trait docs should link to Relationship authored by ItsDoot
Relationship / RelationshipTarget traits should get their own HTML tag authored by alice-i-cecile
StandardMaterial causes long delays on Firefox with WebGL authored by ashivaram23
bevy_remote doesn't work with dynamic components authored by SpecificProtagonist
Render gizmo line widths in logical pixels authored by brianchirls
Add current process cpu/memory usage to SystemInformationDiagnosticsPlugin authored by francisdb
Example `shader_material_bindless` crashes authored by hukasu
DefaultPlugins should mention that `ScheduleRunnerPlugin` is not always included authored by VincentWo
UI specific color enum authored by ickshonpe
Phyisically based unified volumetrics system authored by mate-h
enable partial bindless support on metal authored by mockersf
Cannot lock bevy's patch version authored by jf908
Runtime Predicate Query Filters authored by dmyyy
`animated_fox` example on the website only produces animation events once authored by hukasu
🐢 Create inter-thread executor API authored by joshua-holmes
Errors on blank project: ERROR wgpu_hal::vulkan::instance authored by shwwwa
Gizmos draw below UI authored by torsteingrindvik
Tracking: Take advantage of `ComponentId`'s being discoverable before registration authored by ElliottjPierce
Bevy's default App::update() is limiting for subapp workflows. authored by moonheart08
fov on image rendertarget camera not respected sometimes authored by Fritzoid
why ActiveAnimation update function didn't public? authored by MushineLament