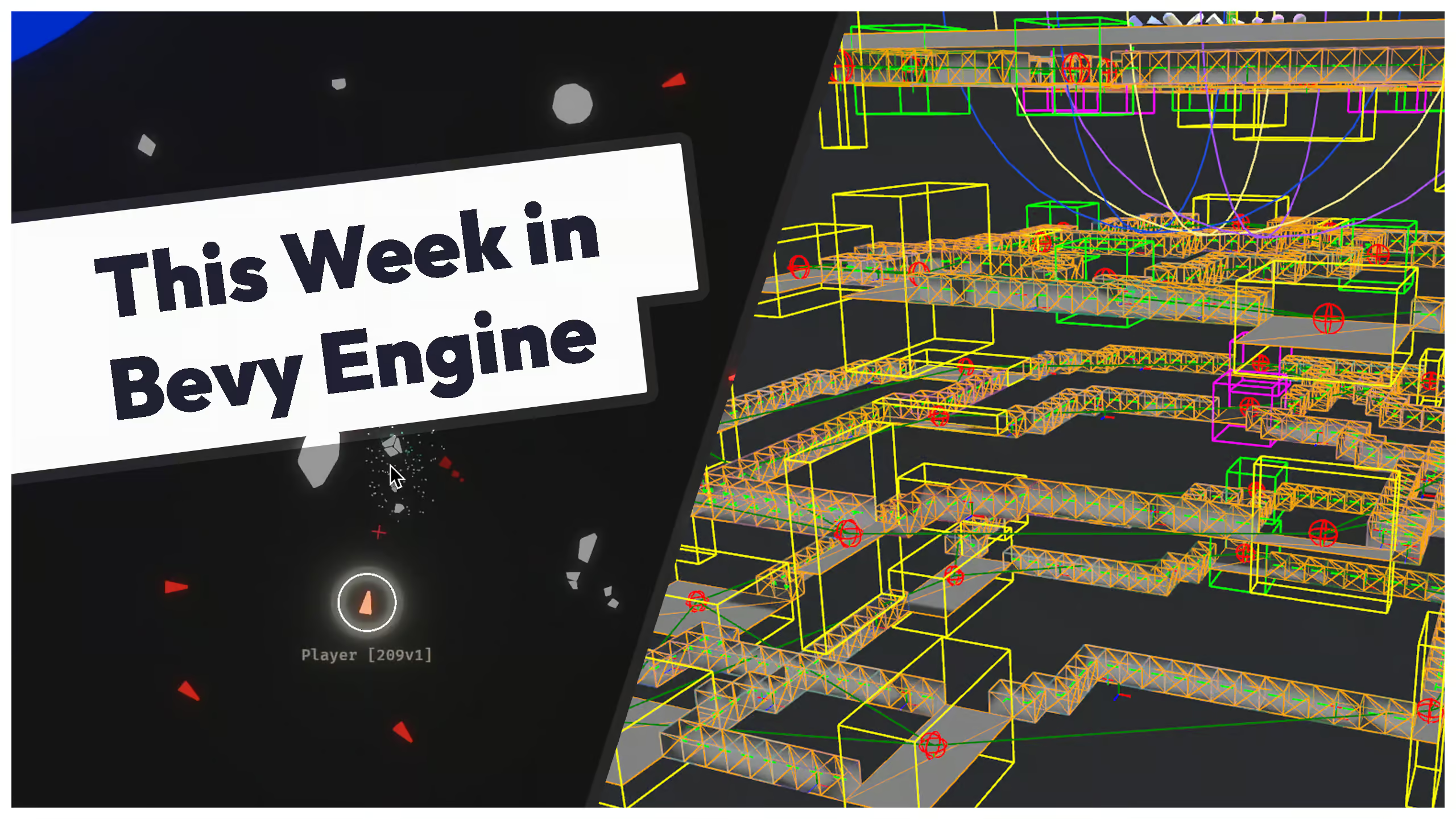
BundleEffects, Fallible Observers, and Planetary meshes
2025-02-17
This week Bevy has upgraded to wgpu 24 and enabled unchecked shaders for added performance.
The next generation scene/ui system is also making headway with improved spawn apis and Bundle effects.
In the showcases this week we see a number of landscape and planetary terrain lod systems, with and without voxels.
Bevy's Next Generation Scene/UI System
Progress towards bsn continues with
#17521: Improved Spawn APIs and Bundle Effects. Previously, it was common to spawn hierarchies of entities using the with_children
or with_child
apis.
commands
.spawn(Foo)
.with_children(|p| {
p.spawn(Bar).with_children(|p| {
p.spawn(Baz);
});
p.spawn(Bar).with_children(|p| {
p.spawn(Baz);
});
});
A new BundleEffect
trait has been added which in addition to other types (such as Spawn
and SpawnRelated
) results in the following next-generation macro-based hierarchy spawning experience.
world.spawn((
Foo,
children![
(
Bar,
children![Baz],
),
(
Bar,
children![Baz],
),
]
))
The order of events is
- The Bundle is inserted on the entity
- Relevant Hooks are run for the insert
- Relevant Observers are run
- The BundleEffect is run
Notably, this extends to any arbitrary Relationship, not just Children
. All together this new functionality enables spawning hierarchies, lists, and relationships with or without macro syntax.
world.spawn((
Foo,
related!(Children[
(
Bar,
related!(Children[Baz]),
),
(
Bar,
related!(Children[Baz]),
),
])
))
and hierarchies can now be returned from functions.
fn button(text: &str, color: Color) -> impl Bundle {
(
Node {
width: Val::Px(300.),
height: Val::Px(100.),
..default()
},
BackgroundColor(color),
children![
Text::new(text),
]
)
}
fn ui() -> impl Bundle {
(
Node {
width: Val::Percent(100.0),
height: Val::Percent(100.0),
..default(),
},
children![
button("hello", BLUE),
button("world", RED),
]
)
}
fn system(mut commands: Commands) {
commands.spawn(ui());
}
Fallible Observers
Building on top of fallible systems, Observers also receive the ability to fail in #17731. This means Observer functions can now take advantage of the ?
operator.
fn fallible_observer(
trigger: Trigger<Pointer<Move>>,
mut world: DeferredWorld,
mut step: Local<f32>,
) -> Result {
let mut transform = world
.get_mut::<Transform>(trigger.target)
.ok_or("No transform found.")?;
*step = if transform.translation.x > 3. {
-0.1
} else if transform.translation.x < -3. || *step == 0. {
0.1
} else {
*step
};
transform.translation.x += *step;
Ok(())
}
Fallible Systems
#17753 introduces configurable error handling for fallible systems at the global, Schedule, and individual system levels.
app.set_system_error_handler(bevy::ecs::result::warn);
MeshTag
Because of mesh preprocessing, @builtin(instance_index)
is not stable and can't be used top reference external data. #17648 introduces a user supplied mesh index that can be used for referencing external data when drawing instanced meshes. The shader/automatic_instancing.rs
example has been updated to show its usage.
Trait Tags
As of #17758 (which will affect Bevy 0.16's docs when it is released), Bevy's docs will have stylized tags for types that implement notable traits, such as Component
, Resource
, and more.
For example, the new MeshTag
now has a Component
tag, making it easier to identify as a Component
.
New Examples
Alice's Merge Train is a maintainer-level view into active PRs, both those that are merging and those that need work.
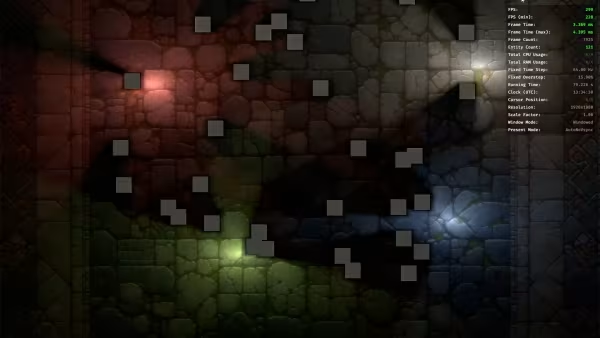
Showcase
Bevy work from the #showcase channel in Discord and around the internet. Use hashtag #bevyengine.
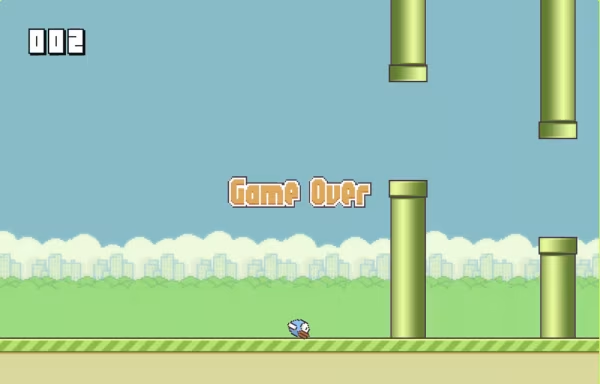
Flappy
showcase
Flappy is a Bevy 0.15 Flappy Bird implementation that is deployed on the web to play and open source for educational purposes.
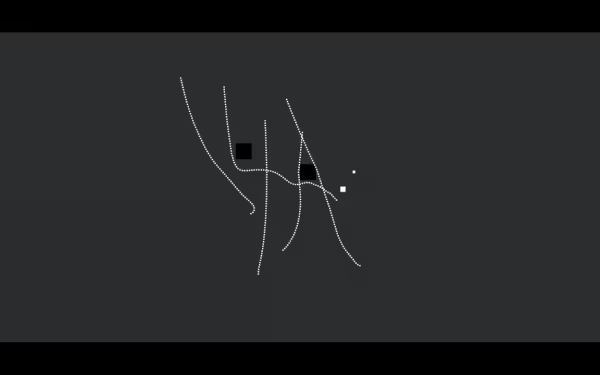
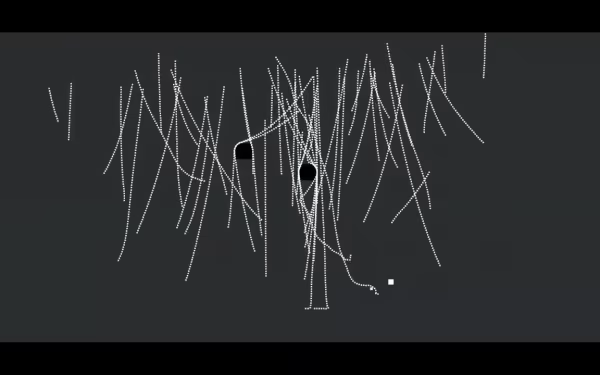
Verlet integration web slinging
showcase
Verlet Integration is a numerical method used to integrate Newton's equations of motion. This demo uses it to create a web slinging effect.
If you want to learn more about Verlet in game applications: Math for Game Programmers: Building a Better Jump covers some.
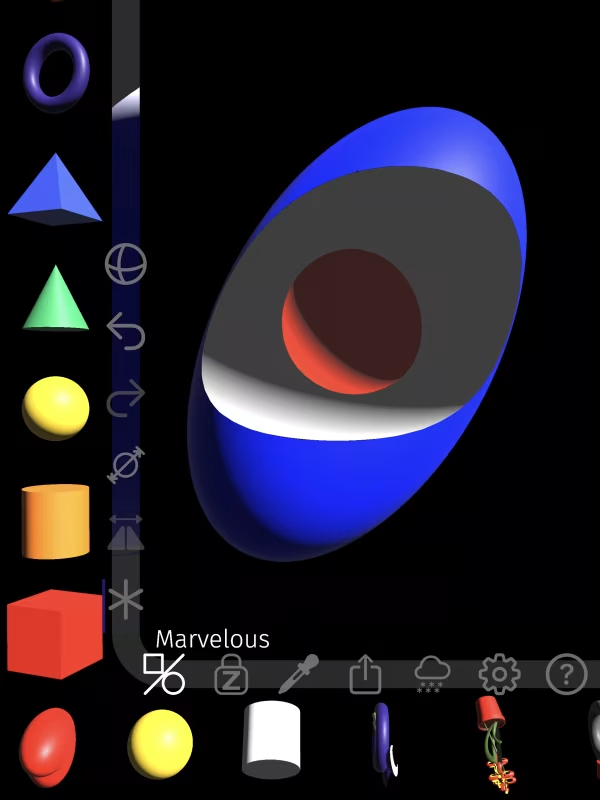
Noumenal’s main shader
showcase
Noumenal’s main shader, now with hybrid ray tracing. The shader computes everything from primitive surface data stored in storage buffers and screen coordinates.
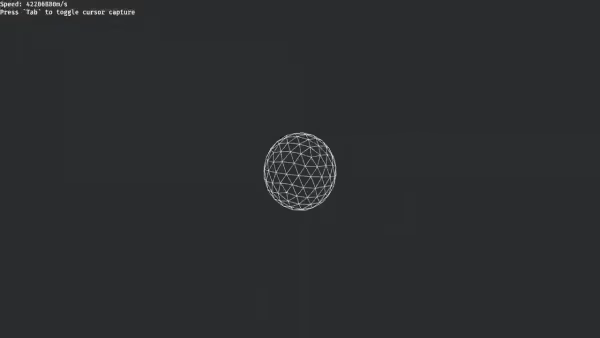
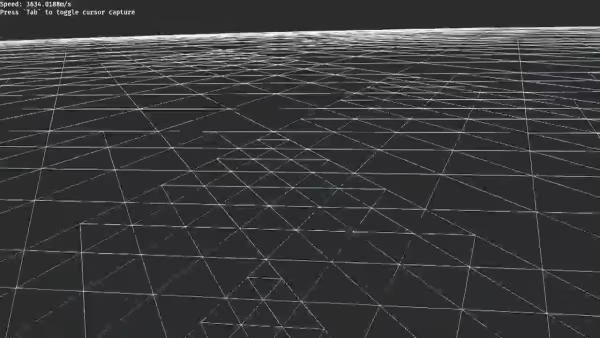
Earth-sized Planet
showcase
An earth-sized planet subdivided down to sub-meter polygons. Meshes subdivide themselves as the player gets closer, and each mesh knows its latitude/longitude as well as barycentric coordinates relative to the 20 top-level icosahedral faces, which it will use to assign itself UVs and heights. The demo makes use of big_space
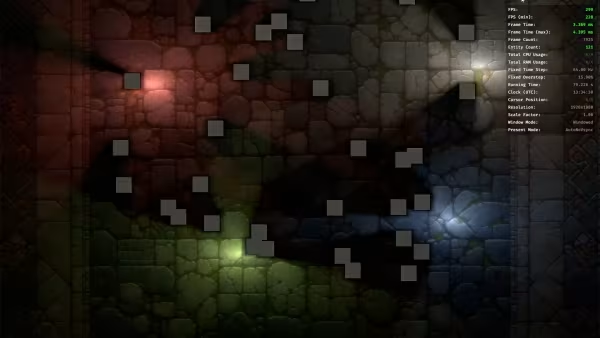
Crates
New releases to crates.io and substantial updates to existing projects
bevy_picking_bvh_backend
crate_release
bevy_picking_bvh_backend is an optimization to mesh picking using a BVH tree (Bounding Volume Hierarchy).
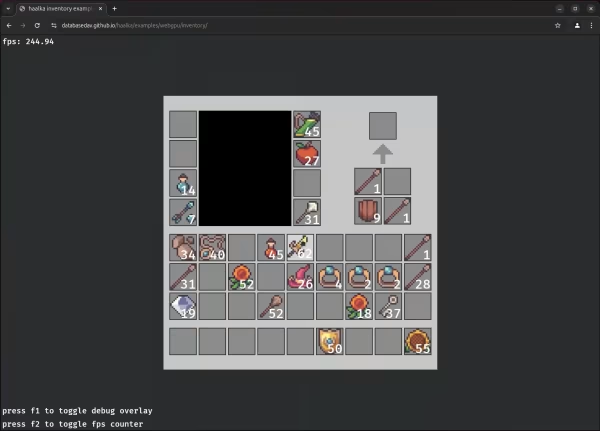
haalka 0.3.0
crate_release
haalka is an ergonomic reactivity library powered by the incredible FRP signals of futures-signals. It is a port of the web UI libraries MoonZoon and Dominator and offers the same signal semantics as a thin layer on top of bevy_ui. While haalka is primarily targeted at UI and provides high level UI abstractions as such, its core abstraction can be used to manage signals-powered reactivity for any entity, not just bevy_ui nodes.
bevy_webserver
crate_release
bevy_webserver is a web server integration that allows you to easily append a webserver to Bevy. It supports creating standalone webapps or appending a webserver to an existing bevy app/game.
bevy_easy_database
crate_release
bevy_easy_database is a persistent storage solution for that automatically serializes and persists your components to disk using fjall as the underlying database.
It supports automatic persistence, hot-reloading of component data, and selective persistence using marker components.
bevy_tailwind v0.2.3
crate_release
bevy_tailwind brings tailwind's classes to Bevy, generating Nodes from a tw
macro.
tw!("p-1", {
"pl-2": true,
"px-3 pl-4": true,
"p-5": true
});
Move specialize_* to QueueMeshes. authored by tychedelia
Fix shadow retention by keying off the `RetainedViewEntity`, not the light's render world entity. authored by pcwalton
Set late indirect parameter offsets every frame again. authored by pcwalton
main_transparent_pass_2d render node command encoding parallelization authored by PPakalns
Cleanup publish process authored by mockersf
deps: bump notify-debouncer-full to remove unmaintained crate authored by SilentSpaceTraveller
Add entity disabling example authored by alice-i-cecile
Upgrade to wgpu v24 authored by JMS55
Harden proc macro path resolution and add integration tests. authored by raldone01
Improved Spawn APIs and Bundle Effects authored by cart
adds example for local defaults authored by newclarityex
many_components stress test improvements authored by hymm
CosmicBuffer is a public type but not not used or accessible in any public API authored by fschlee
Remove `labeled_assets` from `LoadedAsset` and `ErasedLoadedAsset` authored by andriyDev
Add a custom render phase example authored by IceSentry
Encapsulate `cfg(feature = "track_location")` in a type. authored by chescock
Store UI render target info locally per node authored by ickshonpe
Support decibels in bevy_audio::Volume authored by mgi388
Add scroll functionality to bevy_picking authored by colepoirier
`Query::get_many` should not check for duplicates authored by chescock
make bevy math publishable authored by mockersf
Derive Reflect on Skybox authored by jf908
Allowed creating uninitialized images (for use as storage textures) authored by Lege19
Fix documentation of `Entities::get` authored by urben1680
UI clipping update function comments fix authored by ickshonpe
run example in CI on windows using static dxc authored by mockersf
Expand `EasingCurve` documentation authored by greeble-dev
Add user supplied mesh tag authored by tychedelia
Sweep bins after queuing so as to only sweep them once. authored by pcwalton
Silence deprecation warning in `Bundle` derive macro (#17369) authored by Person-93
Add links to the types on the documentation of `GltfAssetLabel` authored by hukasu
Allow users to register their own disabling components / default query filters authored by alice-i-cecile
Fix failing proc macros when depending on bevy through dev and normal dependencies. authored by raldone01
Split the labels in many_buttons authored by ickshonpe
feat(ecs): configurable error handling for fallible systems authored by JeanMertz
Expose method to update the internal ticks of Ref and Mut authored by cBournhonesque
Trait tags on docs.rs authored by SpecificProtagonist
feat(ecs): implement fallible observer systems authored by JeanMertz
UI text extraction refactor authored by ickshonpe
Fix validation errors in `Fox.glb` authored by rparrett
Fix error in scene example authored by rparrett
Don't relocate the meshes when mesh slabs grow. authored by pcwalton
Cache `MeshInputUniform` indices in each `RenderBin`. authored by pcwalton
Panic on failure in `scene` example authored by rparrett
Use `target_abi = "sim"` instead of `ios_simulator` feature authored by madsmtm
Use dual-source blending for rendering the sky authored by ecoskey
Add tonemapping switch to bloom 2d example authored by hukasu
Add ways to configure `EasingFunction::Steps` via new `StepConfig` authored by RobWalt
gate get_tag behind ndef MESHLET_MESH_MATERIAL_PASS authored by mockersf
Add relative position reporting to UI picking authored by bytemunch
Deterministic fallible_systems example authored by mockersf
implement iterators that yield UniqueEntitySlice authored by Victoronz
Use unchecked shaders for better performance authored by JMS55
Add separate option to control font size behavior in `many_text2d` authored by rparrett
Minor tidying in `font_atlas_debug` authored by rparrett
Add ComponentId-taking functions to Entity{Ref,Mut}Except to mirror FilteredEntity{Ref,Mut} authored by anlumo
Meshlet texture atomics authored by JMS55
Shorten the 'world lifetime returned from `QueryLens::query()`. authored by chescock
Switch bins from parallel key/value arrays to `IndexMap`s. authored by pcwalton
`ui_material` example webgl2 fix authored by ickshonpe
Build batches across phases in parallel. authored by pcwalton
Fill out some missing docs for bevy_assets authored by alice-i-cecile
Combine `output_index` and `indirect_parameters_index` into one field in `PreprocessWorkItem`. authored by pcwalton
Improve clarity of existing bevy_assets documentation authored by alice-i-cecile
implement par_iter_many and par_iter_many_unique authored by Victoronz
Add `NodeImageMode` to the UI prelude authored by ickshonpe
Update `typos` to 1.29.6 authored by rparrett
Update `HitData` position docs authored by bytemunch
Remove prepasses from the render world when they're removed from the main world. authored by pcwalton
Actually add objects to the scene buffers in sorted render phases. authored by pcwalton
Add `TypeRegistry::register_by_val` authored by NyxAlexandra
Update picking docs to include position space authored by bytemunch
Bump Rust tracy client version authored by mate-h
Want to contribute to Bevy?
Here you can find two types of potential contribution: Pull Requests that might need review and Issues that might need to be worked on.
Pull Requests Opened this week
UI Text picking authored by bytemunch
Assert a minimum thread count for `TaskPoolOptions` authored by jmskov
Add an example demonstrating how to use default query filtering and entity cloning to create a prefab system authored by alice-i-cecile
Split PointerId into distinct id and PointerKind fields authored by alice-i-cecile
Remove BlitPlugin and introduce TextureBlitter authored by IceSentry
Fix unsoundness in `QueryIter::sort_by` authored by chescock
Shader validation enum authored by fjkorf
Trait-based sections on docs.rs authored by SpecificProtagonist
Add core Error to InvalidDirectionError authored by shafferchance
Retain skins from frame to frame. authored by pcwalton
Resolved UI hierarchy authored by ickshonpe
Remove the id from `PointerId::Touch` authored by ickshonpe
Make sprite picking opt-in authored by notmd
Parallel Transform Propagation authored by aevyrie
Compile Time Query checking and System Parameters authored by MalekiRe
docs(example): Example how to insert reflected `Component` onto entities authored by favilo
Rustdoc html postprocessing authored by SpecificProtagonist
Preserve spawned RelationshipTarget order and other improvements authored by cart
Adding More Comments to the Embedded Assets Example authored by Carter0
Split out the `IndirectParametersMetadata` into CPU-populated and GPU-populated buffers. authored by pcwalton
Add `TypePath::crate_version` authored by MarcGuiselin
Add image sampler configuration in GLTF loader authored by axlitEels
Staged Components authored by ElliottjPierce
Correctly resolve third party bevy crates via `bevy_macro_utils` authored by raldone01
Issues Opened this week
Sprite in `bloom_2d` example is not rendered authored by rparrett
Manual Bundle registration authored by urben1680
InvalidDirectionError doesn't implement std::error::Error authored by anlumo
Entities that should be visible are being culled authored by superdump
Alternative to `remove_with_requires` that doesn't remove components that still have other dependants authored by Zoomulator
TargetCamera mangles ui when an unusual ritual is performed. authored by coolcatcoder
XKB configuration is ignored authored by SL-RU
Make checked vs unchecked shaders configurable authored by alice-i-cecile
Entity{Ref,Mut}Except is duplicate functionality to FilteredEntity{Ref,Mut} authored by anlumo
Inject measure funcs directly into UiSurface authored by ickshonpe
AnimationEvaluationError::ComponentNotPresent should take a ComponentId authored by anlumo
Add support for gyroscope and accelerometer authored by Bcompartment
Rendered geometry "breaks" when adding many primitives authored by coljnr9
No top level feature enabling `bevy_reflect/documentation` authored by makspll
`PickingBehavior::IGNORE` not working with `SceneRoot` authored by Morphylus
Add a `Includes` query filter for working with disabling components authored by alice-i-cecile
`StandardMaterial` doesn't support primitive restart authored by Lege19
Node incorrectly sized with image child under specific conditions authored by danielwolbach
Mesh tangent generation is very slow authored by aloucks
Nearest sampling visual artifacts/glitches on Vulkan backend authored by Akryum
Unflushed commands before exclusive system when configured with `*_ignore_deferred` authored by urben1680
Putting System To Sleep / Suspending app for long periods of time causes UUID panic authored by Braymatter
`BevyManifest` incorrectly assumes that all crates starting with `bevy_` are exposed through `::bevy::` authored by raldone01
Make extending `StandardMaterial` wgsl shader easier for common cases authored by MatrixDev
Sprites do not work with images after get_mut authored by Jakkestt
Rename `EventWriter::send()` to `EventWriter::write()` authored by IceSentry
bevy_winit: Window centering is incorrect when the primary monitor's usable space differs from the intended monitor's authored by etorresh
(wasm) sprites rendering invisible if in background authored by mirsella
Dependabot is failing to create PRs authored by mockersf
Cold Specialization Memory Leaks authored by brianreavis
`WGPU_SETTINGS_PRIO=webgl2` causes panics authored by greeble-dev