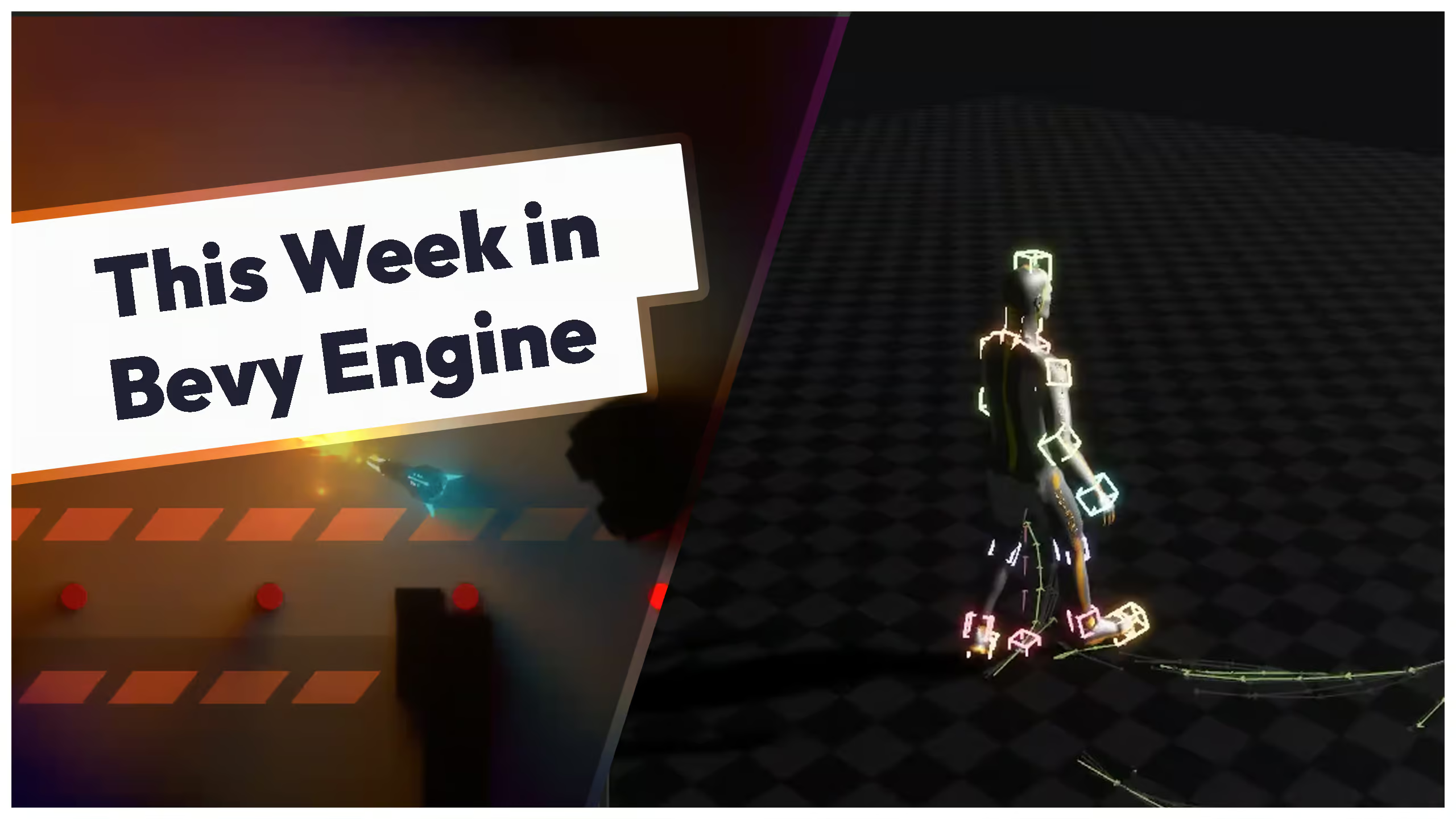
Fallible Systems, Bindless, and Immutable Components
2024-12-09
Now that Bevy 0.15 is out, the PRs that were stacking up behind the release candidate are merging at a fast pace, making this week a very exciting week. Changes that stack up like this and don't make it into a release candidate tend to be interesting features and we really do have an interesting batch.
The Bevy Remote Protocol really struck a chord and has already inspired game debugging prototypes for emacs, neovim, egui, and more.
Half of Pounce Light, the makers of Tiny Glade, gave a talk on their rendering technology at the Graphics Programming Conference titled "Rendering Tiny Glades With Entirely Too Much Ray Marching".
Fallible Systems
Systems in Bevy have historically generally returned unit: ()
and as a consequence, typical error handling used in other areas, like using ?
to return errors, hasn't seen as wide of a use at the system level as it could. #16589 details some of the alternative options currently available as well as introducing systems that can return Result
.
app.add_systems(my_system)
fn my_system(window: Query<&Window>) -> Result {
let window = query.get_single()?;
// ... do something to the window here
Ok(())
}
This is an early step towards some big plans for the future of error handling in Bevy.
Rendering
Multidraw for opaque meshes
#16427 adds support for multidraw, which is a feature that allows multiple meshes to be drawn in a single drawcall.
There are a number of restrictions for when this happens currently, such as only being implemented for opaque 3D meshes, so be sure to read the PR which has a great set of explanations for how you can take advantage of it and where the feature is headed in the future.
Bindless in AsBindGroup
#16368 adds the infrastructure required to support bindless resources in AsBindGroup. The #[bindless(N)]
attribute specifies that, if bindless arrays are supported on the current platform, each resource becomes a binding array of N instances of that resource. A new example shader_material_bindless
shows off the approach.
// `#[bindless(4)]` indicates that we want Bevy to group materials into bind
// groups of at most 4 materials each.
#[derive(Asset, TypePath, AsBindGroup, Debug, Clone)]
#[bindless(4)]
struct BindlessMaterial {
// This will be exposed to the shader as a binding array of 4 *storage*
// buffers (as bindless uniforms don't exist).
#[uniform(0)]
color: LinearRgba,
// This will be exposed to the shader as a binding array of 4 textures and a
// binding array of 4 samplers.
#[texture(1)]
#[sampler(2)]
color_texture: Option<Handle<Image>>,
}
Immutable Components
With the advent of Observers and Hooks in Bevy 0.14 a question was quickly asked about a potential OnMutation
event. Implementing this event contains certain challenges around performance but being able to use hooks and observers to guarantee that values are always up to date is still useful.
#16372 introduces immutable components which through preventing exclusive references enable fully capturing all values, since changes to values can only happen via removal (OnRemove) or replacement (OnInsert).
#[derive(Component)]
#[component(immutable)]
struct Foo {
// ...
}
Box shadows
Bevy 0.15 brought box shadows to UI, and now we have support for multiple box shadows. This is a technique used on the web to create better looking shadows.
StateScoped Entities derive
Bevy supports scoping entities to specific states. Previously to enable that you had to call App::enable_state_scoped_entities::<Scene>()
when building the application. As of #16180, this is now available as a derive when deriving States
and SubStates
.
#[derive(Clone, Eq, PartialEq, Debug, Hash, Default, States)]
#[states(scoped_entities)]
enum MyStates {
#[default]
AssetLoading,
Next,
}
bevy_input_focus
Keyboard focus is a critical component to user interface design, and #15611 introduces a new crate, bevy_input_focus
, which includes a resource to tracking which entity has focus, methods for getting and setting keyboard focus, and a system for dispatching keyboard input elements to the focused entity.
Entity Cloning
#16132 adds an initial implementation of entity cloning, which is a new clone_entity
command creates a clone of an entity with all components that can be cloned.
Performance optimizations are coming in followup PRs.
Misc
Commands.run_schedule
builds onWorld::try_run_schedule
to enable running schedules- Gizmos can now be used in a retained manner, for increased performance.
no_std
bevy_color, bevy_reflect, and bevy_math all got no_std support added!
Alice's Merge Train is a maintainer-level view into active PRs, both those that are merging and those that need work.
Alice also recently started a dogfooding game project currently called "Mischief in Miniature".
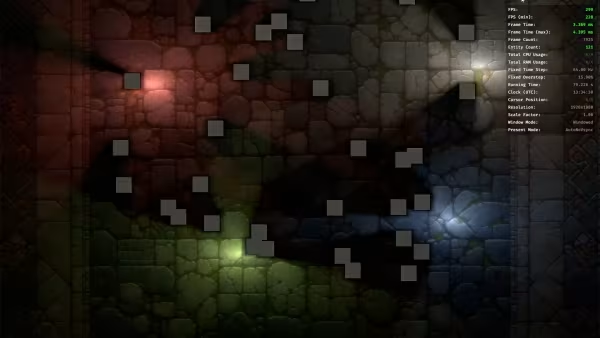
Showcase
Bevy work from the #showcase channel in Discord and around the internet. Use hashtag #bevyengine.
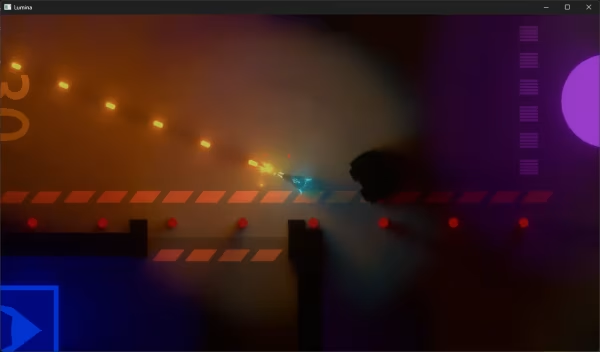
Lumina
showcase
Lumina is a fast paced online multiplayer game that takes advantage of Radiance Cascades for 2d illumination.
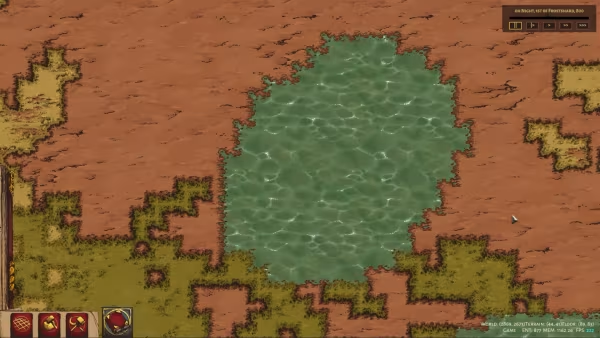
Water and Tile texturing
showcase
New water and a new approach to texturing tiles for Architect of Ruin.
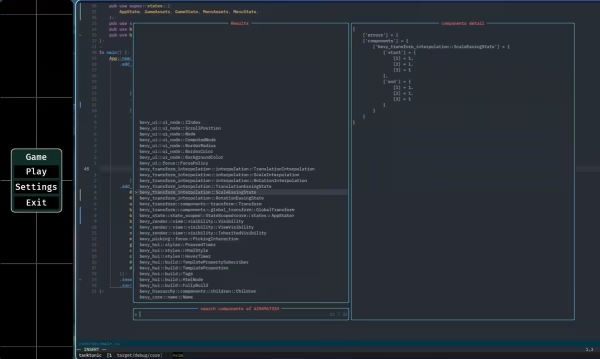
bevy remote protocol in neovim
showcase
A bevy remote protocol inspector prototype, this time in neovim.
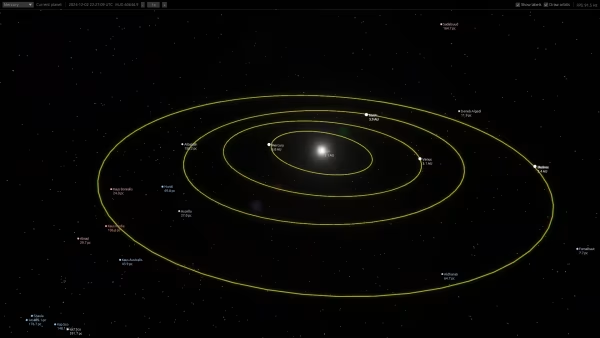
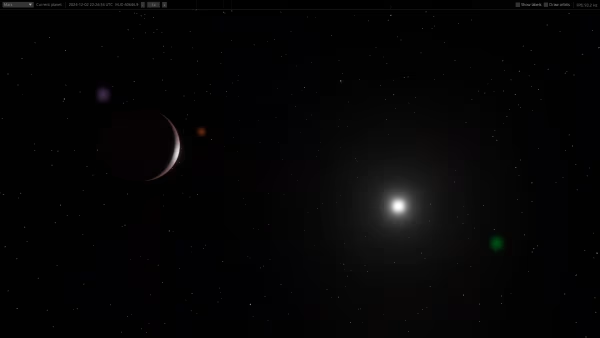
bevy spacesim
showcase
bevy_spacesim is an open-source solar system simulation that has been upgraded to Bevy 0.15 and now takes advantage of 0.15 features like chromatic aberration. The new version also takes advantage of multi-threading in the orbit update system which means you can now fast-forward 1000x to see the planets wizz by
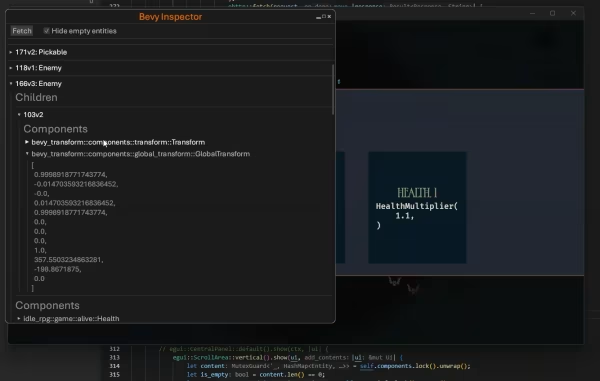
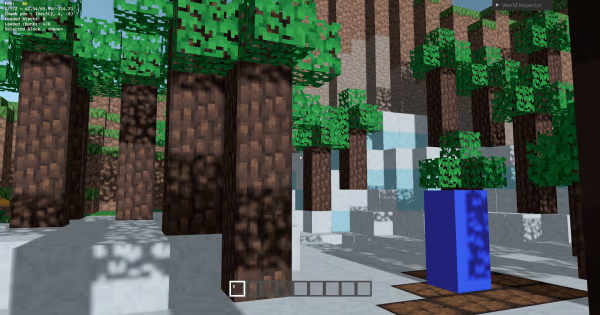
Recreating Minecraft in Bevy
showcase
A project focused on recreating Minecraft using Bevy.
The game is currently fully functional with
- Biomes
- Multiplayer
- Block breaking and placing mechanics
- An inventory system with a working hotbar
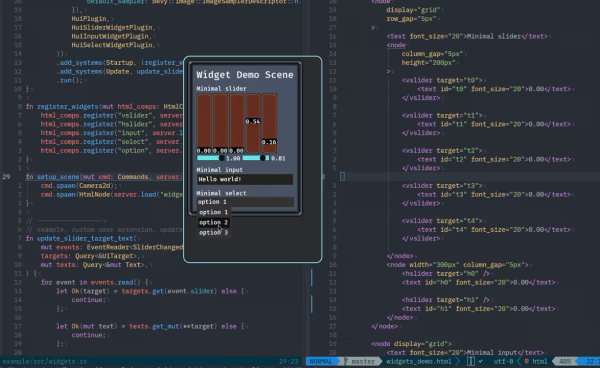
bevy_hui widgets
showcase
a prototype bevy_hui optional widget subcrate. The Idea is to provide just the bevy logic to make a widget work, for as long as your Template uses the defined hierarchy.
<node on_spawn="init_slider" tag:axis="x" >
<button position="absolute">
<slot />
</button>
</node>
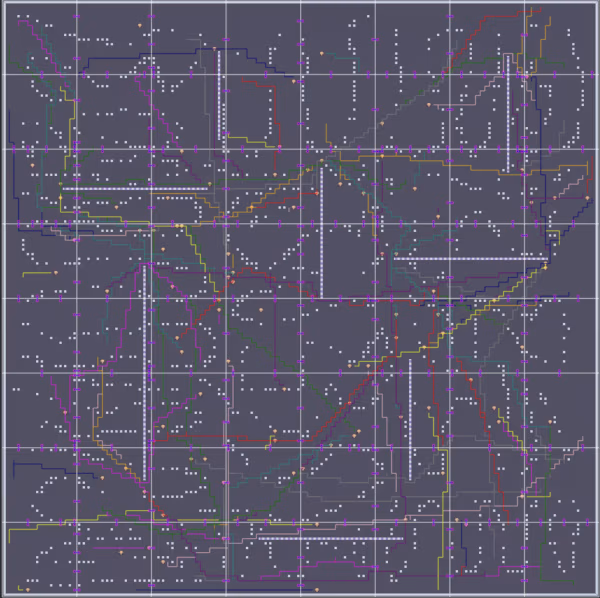
Hierarchical Pathfinding Demo
showcase
This is an in-progress hierarchical pathfinding crate called bevy_northstar
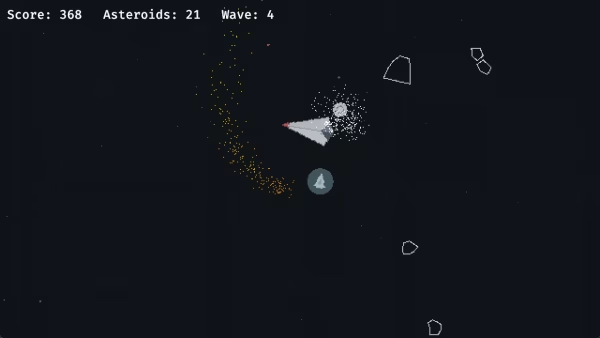
Rusteroids
showcase
Rusteroids is a minimalist, pixel art, Asteroids-like space shooter and is the author's first attempt as Rust and Bevy.
Source is available on GitHub
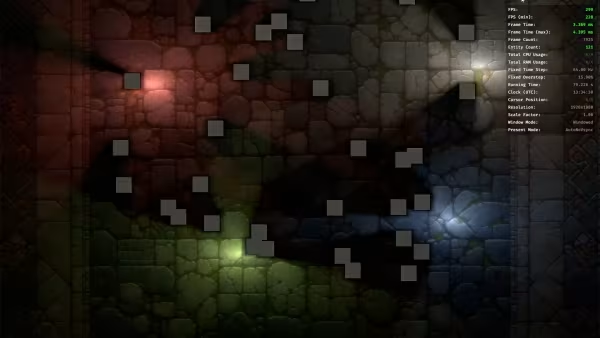
Crates
New releases to crates.io and substantial updates to existing projects
iyes_progress 0.13.0
crate_release
iyes_progress'a goal is to help you implement loading screens where you might have all kinds of things happening (beyond just loading assets), such as custom systems to set up your map/game/multiplayer/whatever. Your systems can report their progress every time they run and iyes_progress will transition your app state for you when everything is ready. It can also give you the cumulative values so you can create a progress bar or other UI indicator.
0.13 is a major overhaul/rewrite of the crate. There is a migration guide in the repo.
Bevy Replicon 0.29.0
crate_release
Bevy Replicon is a crate for server-authoritative networking. 0.29 brings API improvements intended to better support rollback-style networking crates. A number of dependent crates have also updated as a result.
bevy_ios_iap 0.5.0
crate_release
bevy in-app-purchase now supports subscriptions in 0.5 and exposes native APIs via observers.
leptos-bevy-canvas
crate_release
"Embed an idiomatic Bevy app inside your Leptos app."
This crate provides a BevyCanvas
component for embedding Bevy applications and provides tools to help Bevy and Leptos communicate.
The crate is compatible with Bevy 0.15 as well as Leptos' recent 0.7 release.
bevy_heavy
crate_release
bevy_heavy is a crate for computing mass properties (mass, angular inertia, and center of mass) for the 2D and 3D geometric primitives in Bevy's math crate, bevy_math. This is crucial for things like accurate physics simulations.
bevy_transform_interpolation
crate_release
Gameplay systems and physics simulations often use a fixed timestep for consistent, frame rate independent behavior. However, this can lead to visual stutter when the timestep doesn't match the display refresh rate. bevy_transform_interpolation is a drop-in Transform interpolation solution for fixed timesteps in Bevy 0.15
bevy_ios_gamecenter 0.3.0
crate_release
Bevy Plugin and Swift Package to provide access to iOS native Gamecenter from inside Bevy. 0.3 brings a new observer-based API
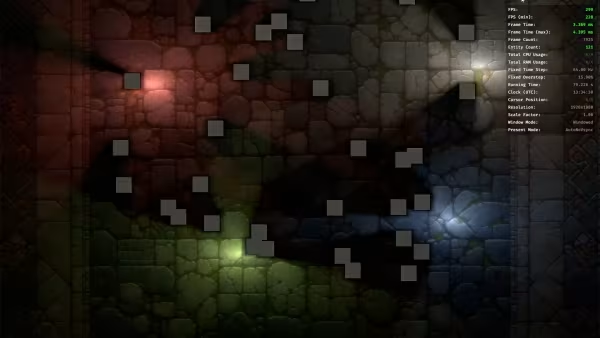
Educational
Tutorials, deep dives, and other information on developing Bevy applications, games, and plugins
How to make a triple AAA game in rust? Playlist
educational
This tutorial series covers how to make a networked game with functional physics. There are a number of videos already added to the playlist and seemingly more to come.
Refactor `FunctionSystem` to use a single `Option` authored by ItsDoot
Updated comment: ZIndex::Local(0) -> ZIndex(0). authored by 772
Multiple box shadow support authored by ickshonpe
Add .contains_aabb for Frustum authored by Soulghost
Getting `QueryState` from immutable `World` reference authored by vil-mo
make example external_source_external_thread deterministic authored by mockersf
enable_state_scoped_entities() as a derive attribute authored by mockersf
Update example link authored by RCoder01
Add `Commands::run_schedule` authored by bushrat011899
Update Contributor's Guide link in README.md authored by james-j-obrien
Remove `petgraph` from `bevy_ecs` authored by bushrat011899
Upgrade to Taffy 0.6 authored by nicoburns
Update rodio requirement from 0.19 to 0.20 authored by mnmaita
Move required components doc to type doc authored by SpecificProtagonist
Add a bindless mode to `AsBindGroup`. authored by pcwalton
Use disqualified for B0001 authored by SpecificProtagonist
switch bevy_mesh to use wgpu-types instead of wgpu authored by atlv24
Make `StateTransitionSteps` public authored by Phoqinu
Rename `UiBoxShadowSamples` to `BoxShadowSamples`. authored by ickshonpe
Remove flush_and_reserve_invalid_assuming_no_entities authored by SpecificProtagonist
Remove the `min` and `max` fields from `LayoutContext`. authored by ickshonpe
Use `IntoIterator` instead of `Into<Vec<..>>` in cubic splines interfaces authored by mweatherley
Move `all_tuples` to a new crate authored by BenjaminBrienen
Entity cloning authored by eugineerd
Native unclipped depth on supported platforms authored by JMS55
Compute better smooth normals for cheaper, maybe authored by shanecelis
bevy_winit(emit raw winit events) authored by HugoPeters1024
Add `no_std` Support to `bevy_math` authored by bushrat011899
implement the full set of sorts on QueryManyIter authored by Victoronz
switch bevy_image to use wgpu-types wherever possible instead of wgpu authored by atlv24
Make visibility range (HLOD) dithering work when prepasses are enabled. authored by pcwalton
Add missing registration for `TextEntity` authored by coreh
BrpQueryRow has field deserialization fix authored by Leinnan
Retained `Gizmo`s authored by tim-blackbird
Improve profiling instructions authored by JMS55
Cluster light probes using conservative spherical bounds. authored by pcwalton
Add Immutable `Component` Support authored by bushrat011899
Add "bevy_input_focus" crate. authored by viridia
Expose `SystemMeta`'s access field as part of public API authored by vil-mo
Turn `apply_deferred` into a ZST System authored by ItsDoot
Window picking authored by NthTensor
Flush commands after every mutation in `WorldEntityMut` authored by nakedible
Add `no_std` support to `bevy_reflect` authored by bushrat011899
Add optional transparency passthrough for sprite backend with bevy_picking authored by vandie
Retain `RenderMeshInstance` and `MeshInputUniform` data from frame to frame. authored by pcwalton
Add `no_std` support to `bevy_color` authored by bushrat011899
picking: disable raycast backface culling for Mesh2d authored by globin
Fallible systems authored by NthTensor
Do not attempt to send screenshots to Pixel Eagle without a token authored by LikeLakers2
Fix the `texture_binding_array`, `specialized_mesh_pipeline`, and `custom_shader_instancing` examples after the bindless change. authored by pcwalton
Move `clone_entity` commands to `EntityCommands` authored by JaySpruce
Fix mehses -> meshes typo authored by Jondolf
Skip all jobs on the `Weekly beta compile test` workflow when running on a fork authored by LikeLakers2
Deny `derive_more` `error` feature and replace it with `thiserror` authored by bushrat011899
Use multidraw for opaque meshes when GPU culling is in use. authored by pcwalton
Run observers before hooks for `on_replace` and `on_remove` authored by nakedible
bevy_input_focus improvements (follow-up PR) authored by viridia
Add `no_std` support to `bevy_tasks` authored by bushrat011899
Fix error in volumetric fog shader authored by rparrett
add missing type registration for `Monitor` authored by jakobhellermann
Update breakout to use Required Components authored by Mclilzee
Want to contribute to Bevy?
Here you can find two types of potential contribution: Pull Requests that might need review and Issues that might need to be worked on.
Pull Requests Opened this week
Added benchmark for large schedule authored by Trashtalk217
`ScrollPosition` scale factor fix authored by ickshonpe
Remove the coordinate rounding from `extract_text_sections`. The coor… authored by ickshonpe
Move UI `Transform` to a field on `Node` authored by ickshonpe
add line height to `TextFont` authored by Cyborus04
add index_range for TextureAtlas authored by useyourfeelings
Add alpha mode implementation to shader_material_2d authored by Leinnan
Update nixos link in linux_dependencies.md authored by TomMD
Batch skinned meshes on platforms where storage buffers are available. authored by pcwalton
Forward decals (port of bevy_contact_projective_decals) authored by JMS55
Moving `PlaybackMode` to `AudioPlayer` and defaulting to `PlaybackMode::Remove` authored by seivan
Set panic as default fallible system param behavior authored by MiniaczQ
Use a read-only depth buffer for transparent/transmissive passes authored by JMS55
Update winit_runner to use spawn_app for wasm32 target authored by xuwaters
Unify ExclusiveSystemParams with normal SystemParams authored by ItsDoot
Remove the need for `collect_inner()` on `QuerySortedManyIter` authored by chescock
Descriptive error message for circular required components recursion authored by SpecificProtagonist
Make `StandardMaterial` bindless. authored by pcwalton
`OverflowClipBox::MarginBox` authored by ickshonpe
Improve child_builder add_child documentation slightly authored by VictorElHajj
Added `with_component` to `EntityWorldMut`, `DeferredWorld`, and `World` authored by bushrat011899
Fix scaling for UI debug overlay authored by ickshonpe
fix tiny copy-paste mistake in `bevy_text::font_atlas_set` authored by onkoe
Make `Parent` and `Children` components immutable authored by tim-blackbird
Implement bindless lightmaps. authored by pcwalton
Use multidraw for shadows when GPU culling is in use. authored by pcwalton
Turn on GPU culling automatically if the target platform supports it. authored by pcwalton
Fix atan2 docs authored by dbeckwith
Add some basic tests for easing functions authored by scottmcm
Link to required components docs in component type docs authored by Jondolf
More complete documentation of valid query transmutes authored by chescock
Cache systems by `S` instead of `S::System` authored by benfrankel
Draw the UI debug overlay using the UI renderer authored by ickshonpe
Allow holes in the `MeshInputUniform` buffer. authored by pcwalton
Rename `EntityCommands::clone` to `clone_and_spawn` authored by JaySpruce
Physical Shadow Softness Parameter authored by ecoskey
Rename `RayCastSettings` to `MeshRayCastSettings` authored by Jondolf
Remove COPY_DST from AsBindGroup uniform buffers authored by JMS55
Issues Opened this week
[MacOs] 0.15 panic: wgpu error: Validation Error authored by extrawurst
Crash on macOS, related to ExtendedMaterial and custom vertex buffer layout authored by splashdust
Warning `Too many textures in mesh pipeline view layout` when using SSAO and TAA together authored by lomirus
Mesh Over-Allocates memory, causing program to crash authored by Asempere123123
Node fields as components authored by tropical32
Add feature to disallow a Type of Component to coexist on an entity authored by pemattern
`NextState<T>` event gets silently dropped under specific conditions authored by Phoqinu
Systems as entities authored by andriyDev
Upgrade typos job in CI and fix the typos detected authored by alice-i-cecile
Improve wording for link to contributing guide authored by alice-i-cecile
Main branch fails to compile on Rust beta. authored by github-actions[bot]
`GlobalTransform::forward` sometimes panics in debug builds authored by MatrixDev
iOS screen rotation broken in 0.15 authored by extrawurst
Mesh picking example doesn't work authored by spectria-limina
Make `StateTransitionSteps` public for better ergonomics of State Scoped Resources authored by Phoqinu
Add a method to get a component `Ptr` to `ThinColumn` authored by Shatur
`Taffy` crashes authored by Phoqinu
iOS app panics on will_terminate authored by extrawurst
example `shadow_biases` has many shadow artefacts authored by mockersf
bevy_mobile_example crashes during runtime authored by Valetoriy
Require release notes and migration guides in the Bevy repo as part of PRs authored by alice-i-cecile
Skybox rotation issue authored by DiSaber
Misaligned text inside UI authored by musjj
Bevy UI freezes application after window resize authored by MatrixDev
Reintroduce 0.14.2 behavior for splines inner elements authored by ThomasCartier
Use Cargo workspace features for internal dependency versions authored by viridia
The safety invariant of `SystemParam::validate_param` is not documented. authored by spectria-limina
Prefix benchmark names with module path authored by BD103
Cycles in required components produce inscrutable error messages authored by rparrett
Docs for RenderSet are at least slightly inaccurate authored by rparrett
Directional lights cascade shadows not working when using multiple render layers authored by doup
The reflection of bevy_render::sync_world::TemporaryRenderEntity doesn't show up as a Component authored by anlumo
Wrong links in `PointerHits` doc authored by Selene-Amanita
Support generating or redirecting rays for picking authored by Selene-Amanita
`DebugUiPlugin` doesn't respect scale factor properly authored by JeanMertz
`EaseFunction::ExponentialIn` jumps at the beginning authored by scottmcm
High-degree easing functions shouldn't be jerky authored by scottmcm
Forward rendering seems broken authored by JMS55
`SystemRunner` param - run systems inside other systems authored by vil-mo
Accept raw vertex buffer data when creating a Mesh authored by ThomasVille
create_surfaces is unsound authored by spectria-limina
Migration Guide 0.14 -> 0.15 missing stuff authored by Bytekeeper
out of bounds panic in `bevy_pbr` `remove_mesh_input_uniform` when running `testbed_3d` authored by jakobhellermann
Screenshot does not contains the `bevy_egui` GUI in 0.15 anymore authored by thmxv
panic in collect_meshes_for_gpu_building: index out of bounds authored by mockersf
Multiple `AnimationTarget` per entity authored by musjj
Using `TextWriter` with an invalid span index produces an inscrutable panic authored by rparrett
Render Volumetric Fog at Lower Resolution authored by ambiso
Split screen example crashes when minimizing the window authored by d28n