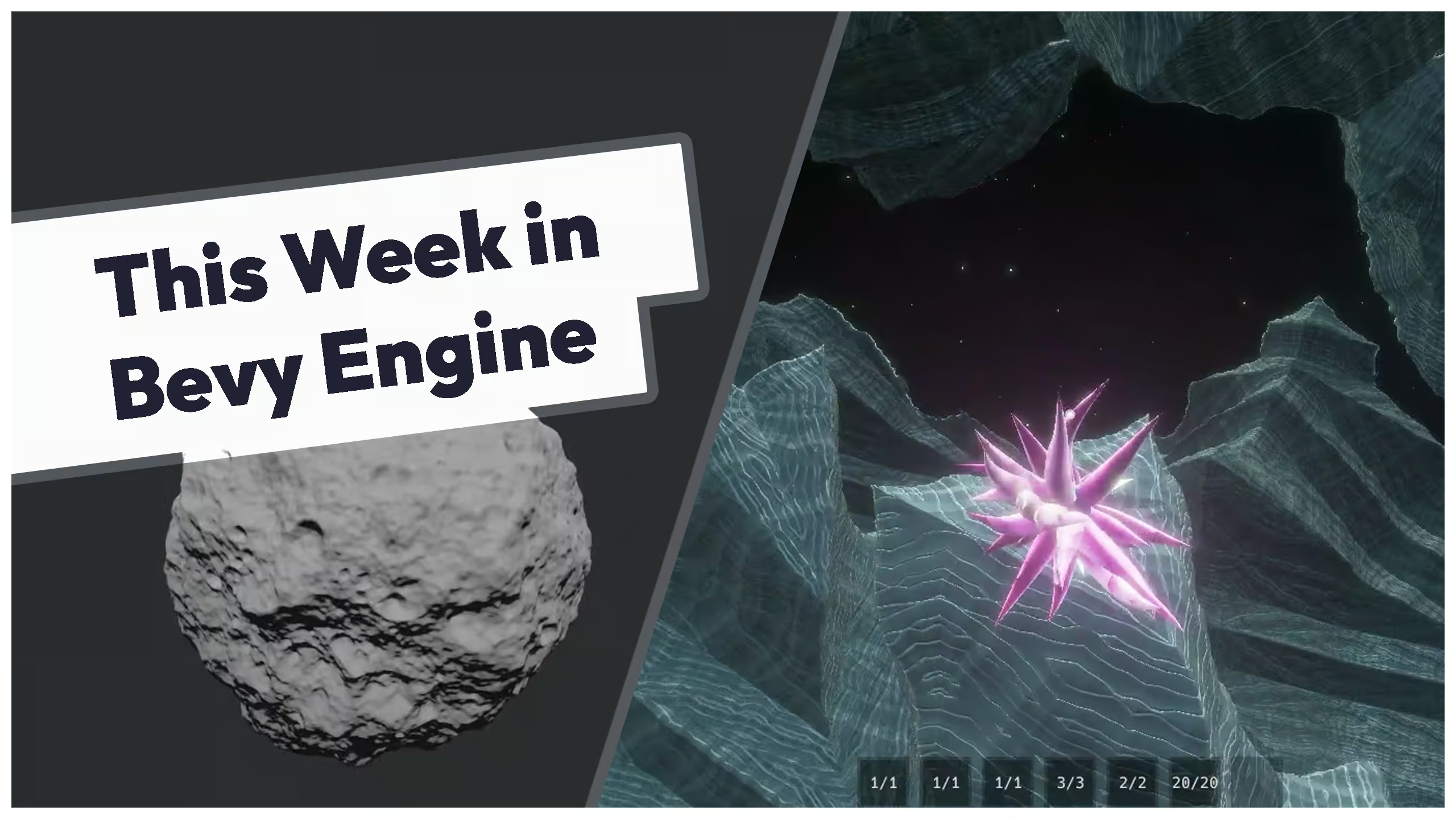
Asteroids, Shadows, and the Entry API
2024-09-23
Welcome back to another week in Bevy!
This week we see some much needed documentation added to Assets v2, the base of generalized animations, and the ability to turn anti-aliasing on and off.
On top of that there's a new compute-shader based asteroid generator, a fragment shader-based raytracing example, and updates on a couple of games.
Assets
bevy_assets
and asset processing both got some brand new, much needed documentation.
Percentage-Closer Soft Shadows (PCSS)
Percentage-closer soft shadows (PDF) are a technique from 2004 that allow shadows to become blurrier farther from the objects that cast them. #13497 introduces PCSS to Bevy including wonderfully documented Pull Request and code behind it.
A new 3d/pcss
example is also introduced.
Reflecting derived traits
#[derive(Component, Default, Reflect)]
-#[reflect(Component)]
+#[reflect(Component, Default)]
pub struct AnimationPlayer {
All components and resources got additional traits that they derive, like Default
, reflected as well in a large number of PRs this week, all by the same author. As a result, the reflection information for all components and resources is much more fleshed out now than it was last week.
You can find out more about why and how in #15187
HeadlessPlugins
There are DefaultPlugins
, which is what most Bevy apps use when using various functionality, and MinimalPlugins
, which are a set of plugins mostly useful for testing. As of
#15260 there is now HeadlessPlugins
, which is aimed at being more functional than MinimalPlugins
and less functional than the DefaultPlugins
and mostly used on servers.
Turns
In #15169 the Rot2
APIs got extended with the ability to specify rotation in terms of Turns.
Ui Anti-Aliasing
As of #15170 anti-aliasing in UI can now be turned off using a new UiAntiAlias
component. This is useful for hard-pixel retro style game UI.
use bevy::{prelude::*, ui::prelude::*};
commands.spawn((Camera2dBundle::default(), UiAntiAlias::Off));
EntityCommands entry API
The "entry API" is an idiomatic Rust convention that starts out with an entry
call and provides a set of additional functions for manipulating the existing or non-existing values.
#15274 introduces a subset of the entry API for modifying or inserting component values.
commands
.entity(player)
.entry::<Level>()
.and_modify(|mut lvl| lvl.0 += 1)
.or_default();
Picking
Picking upstreaming continues a variety of bug fixes.
Generalized Animation
This week #15207 sets the stage for the as-yet-unmerged generalized animation PR. #15207 is a deep PR but has great comments, so could be great to read over for anyone interested in getting deeper into the ECS.
Alice's Merge Train is a maintainer-level view into active PRs, both those that are merging and those that need work.
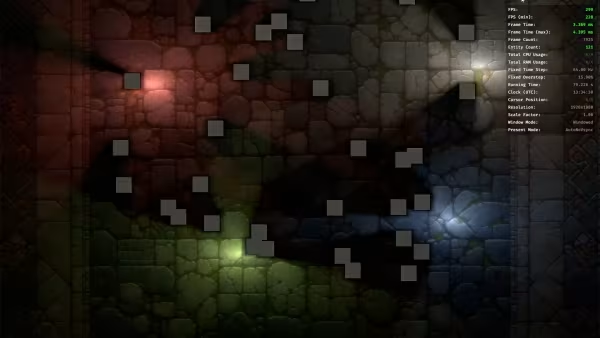
Showcase
Bevy work from the #showcase channel in Discord and around the internet. Use hashtag #bevyengine.
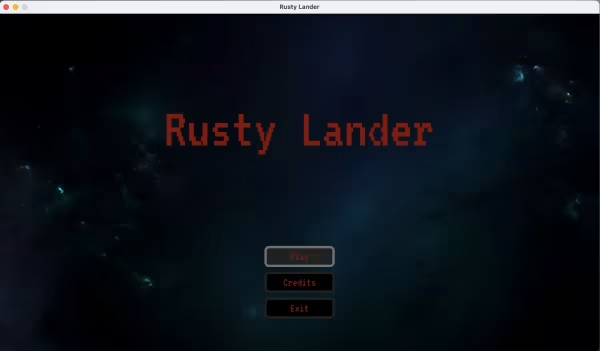
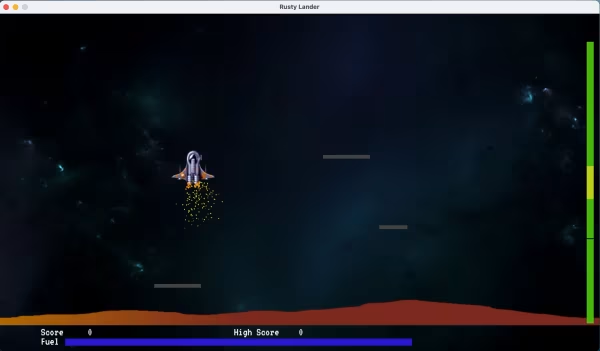
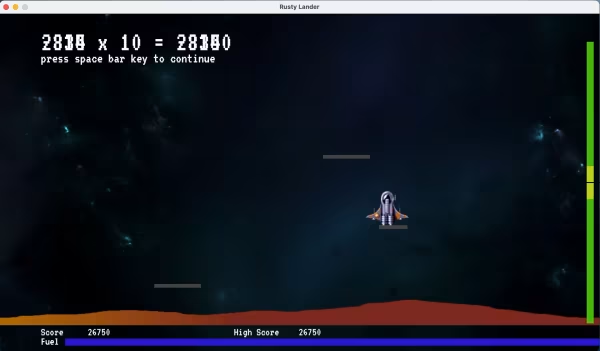
Rusty Lander v0.2
showcase
Rusty Lander got a v0.2 release. The game is still a work in progress. New work includes fonts and colors as well as gameplay and UI:
- add dynamic thrust particle's property effect
- show fuel, score & high score UI texts
- update score after spaceship landing
- add space key pressed after landing system
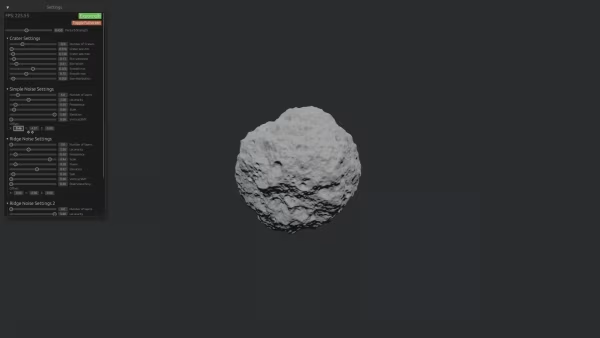
AstroGen
showcase
A procedural asteroid generator in bevy using compute shaders, with source code available!
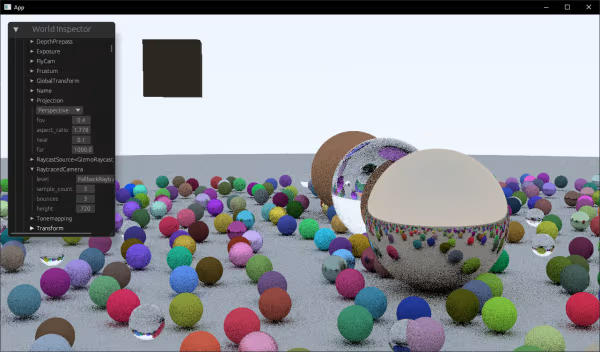
Bevy raytracing in one weekend
showcase
"raytracing in one weekend" done in a single fragment shader pass in bevy.
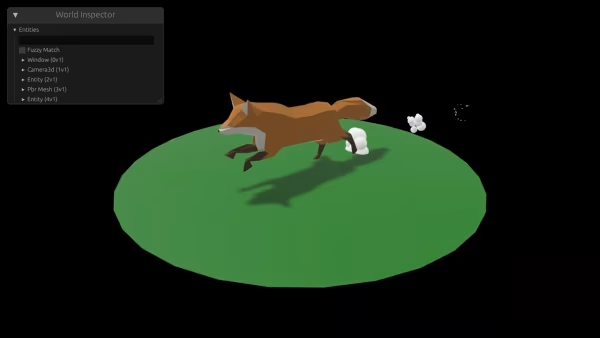
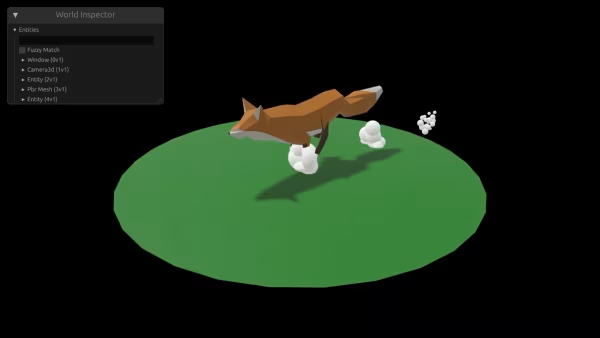
Mesh Particles in bevy_hanabi
showcase
This demo showcases mesh particles (the smoke puffs) in bevy_hanabi. The PR is up in draft form for upstreaming back to bevy_hanabi so hopefully we see it in a release soon.
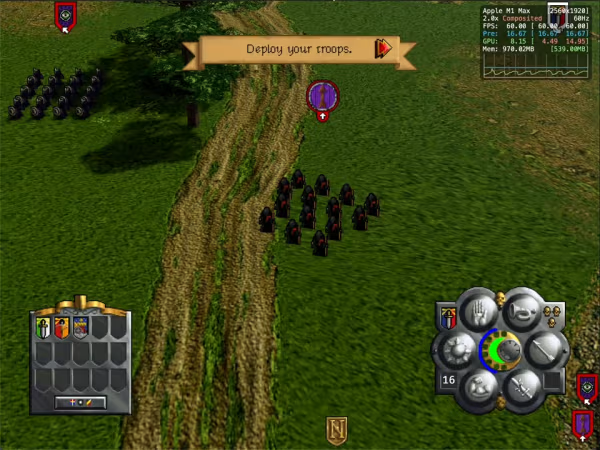
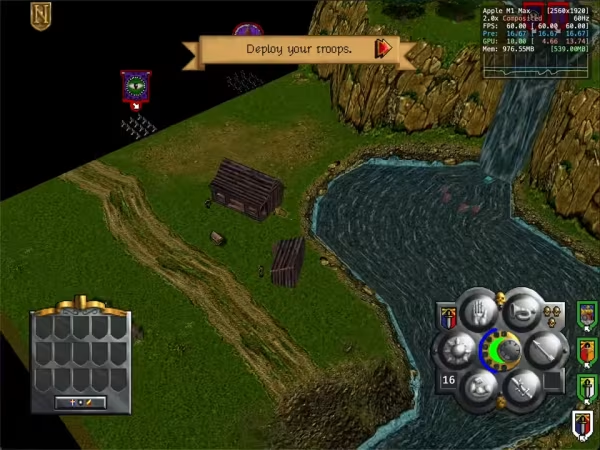
Warhammer: Dark Omen in Bevy
showcase
This is a reproduction of Warhammer: Dark Omen in Bevy. The project has been in development for about 6 months during which the developer has been slowly chipping away at the hard gameplay problems.
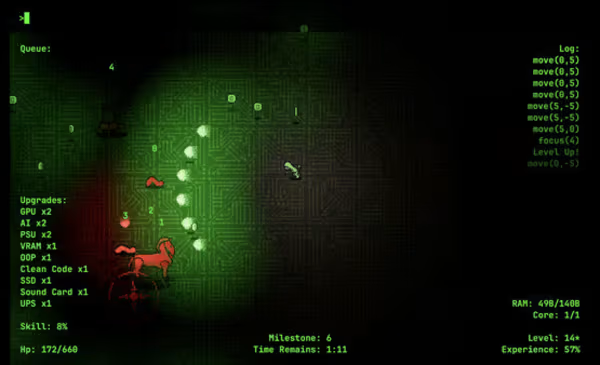
HackeRPG Steam Alpha
showcase
HackeRPG is an arena roguelike where the mission is to stay in an arena full of virtual enemies for 15 minutes. It is now on Steam in alpha.
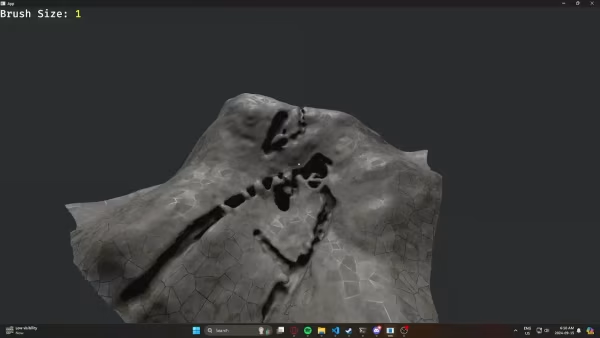
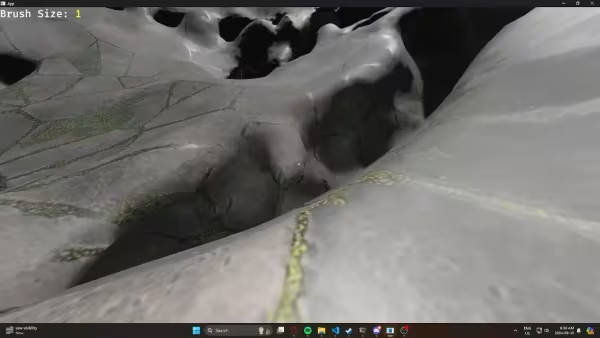
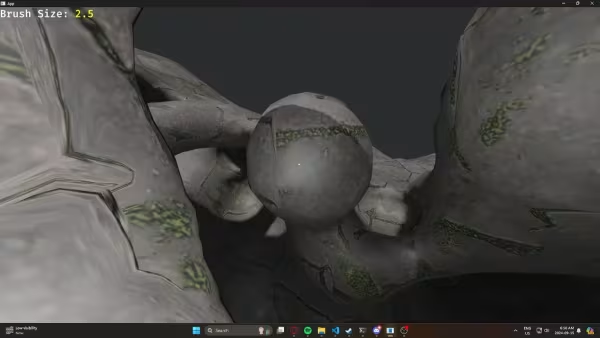
Fast Surface Nets
showcase
The continuation of last week's marching cubes conversion to fast-surface-nets continues. Future work includes wacom-tablet based sculpting, which already has a working octotablet/bevy implementation, and automatic decorating of the mesh.
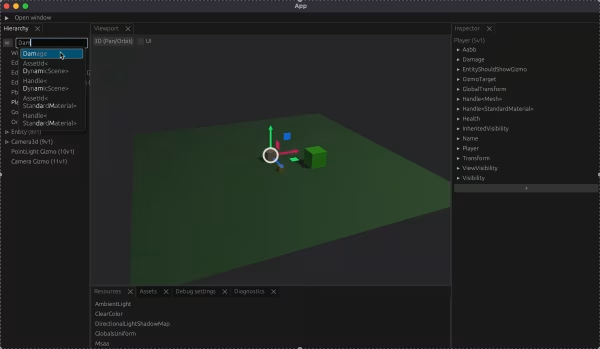
bevy_editor_pls enhancements
showcase
A fork of bevy_editor_pls
that allows the querying of hierarchy with filters:
w
: `Withx
:Without
i
: Entity Index.
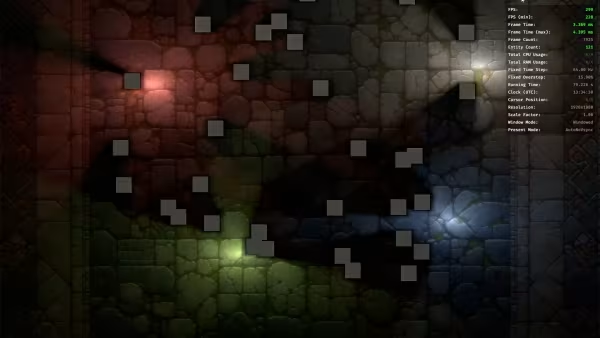
Crates
New releases to crates.io and substantial updates to existing projects
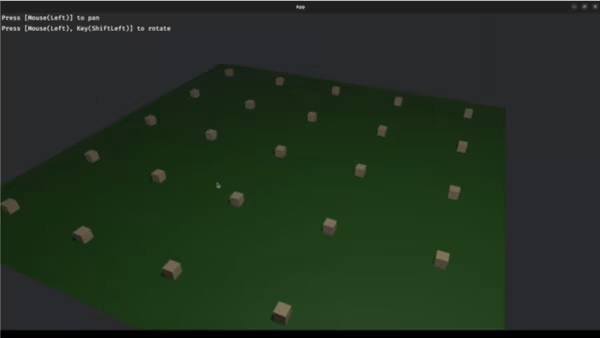
bevy_map_camera
crate_release
A 3D camera controller inspired by Google Maps, f4maps and Change Finder.
Based upon LookTransform, LookAngles and Orbital Camera Controller from smooth-bevy-cameras.
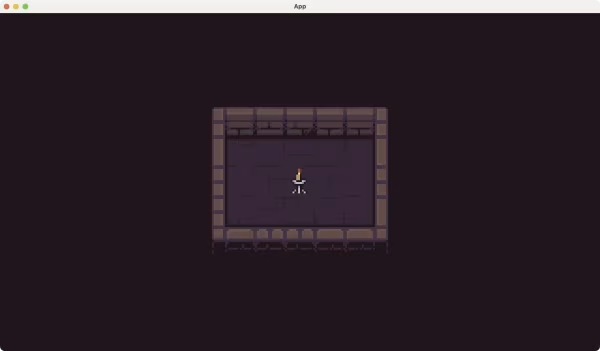
bevy_light_2d 0.4.0
crate_release
bevy_light_2d
is a general purpose 2d lighting plugin.
Its 0.4 release includes:
- Point lights colors are now added to ambient light, instead of multiplied by it
- Fixed point lights rendering despite being despawned
- Fixed shadow sometimes appearing when no occluders were present
Migration guide
Point light intensity needs to be adjusted to account for changes to ambient light. Generally this means point light intensity values need to be lowered. See the relevant changes to the dungeon
example.
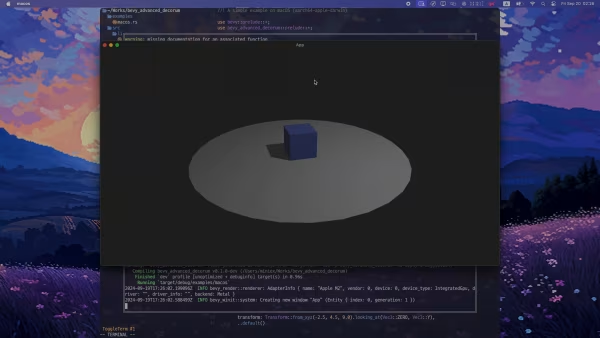
bevy_advanced_decorum
crate_release
bevy_advanced_decorum
is a window decoration plugin. Its first release includes:
- customizable titlebar transparency
- toggle window title visibility
- control over standard window buttons (close, minimize, zoom)
- fine-tuned positioning of window controls
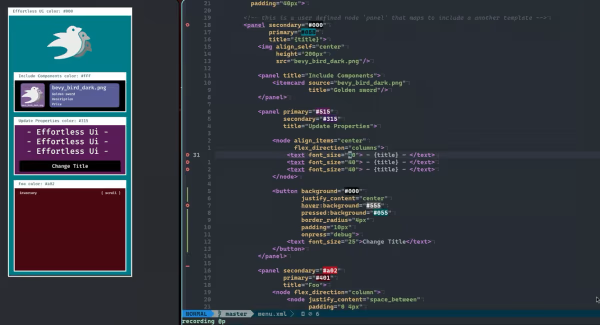
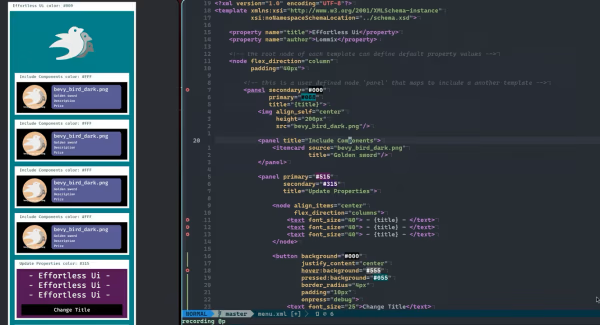
lommix_ui MVP
crate_release
The MVP for a new XML-based UI crate with hot-reloadable workflows.
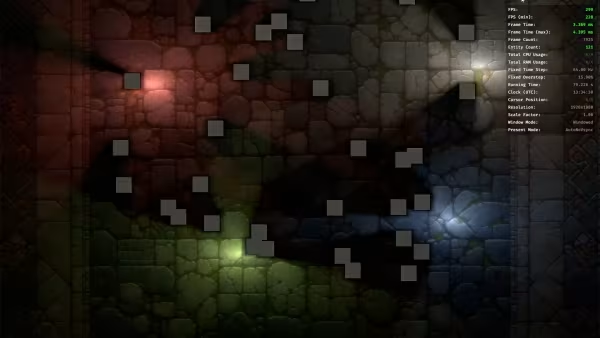
Educational
Tutorials, deep dives, and other information on developing Bevy applications, games, and plugins
retrofit bevy_quickstart with bevy_asset_loader
educational
This post cover's the authors own experience replacing the bevy quickstart's asset loading with bevy_asset_loader.
Apply your Transforms!
educational
Blender will happily let you scale, rotate and perform other operations on your objects.. which can result in unexpected output if you're not sure what's going on. This post covers what that looks like and how to fix it!
Reflect derived traits on all components and resources: bevy_pbr authored by blazepaws
Use `FromReflect` when extracting entities in dynamic scenes authored by yrns
Reflect derived traits on all components and resources: bevy_gizmos authored by blazepaws
Reflect derived traits on all components and resources: bevy_gltf authored by blazepaws
explicitly mention `component` in methods on `DynamicSceneBuilder` authored by atornity
Reflect derived traits on all components and resources: bevy_hierarchy authored by blazepaws
Reflect derived traits on all components and resources: bevy_input authored by blazepaws
Use of deprecated function in example for ButtonInput authored by blazepaws
add `allow_all` and `deny_all` methods to `DynamicSceneBuilder` authored by atornity
Reflect derived traits on all components and resources: bevy_picking authored by blazepaws
Reflect derived traits on all components and resources: bevy_render authored by blazepaws
Reflect derived traits on all components and resources: bevy_sprite authored by blazepaws
Reflect derived traits on all components and resources: bevy_state authored by blazepaws
Reflect derived traits on all components and resources: bevy_text authored by blazepaws
Reflect derived traits on all components and resources: bevy_window authored by blazepaws
Reflect derived traits on all components and resources: bevy_ui authored by blazepaws
Reflect derived traits on all components and resources: bevy_ecs authored by blazepaws
Example for bevy_ecs::event::Events uses deprecated function get_reader authored by blazepaws
Reflect derived traits on all components and resources: bevy_transform authored by blazepaws
Reflect derived traits on all components and resources: bevy_animation authored by blazepaws
Reflect derived traits on all components and resources: bevy_audio authored by blazepaws
Reflect derived traits on all components and resources: bevy_core_pipeline authored by blazepaws
Increase border_rect for TextureSlicer to match image authored by tjlaxs
Substitute trivial fallible conversions with infallible function calls authored by Nilirad
Remove redundent information and optimize dynamic allocations in `Table` authored by Adamkob12
Fix `MeshAllocator` panic authored by eero-lehtinen
Improve type inference in `DynSystemParam::downcast()` by making the type parameter match the return value. authored by chescock
Add missing insert API commands authored by crvarner
Rotation api extension authored by RobWalt
Enable/disable UI anti-aliasing authored by patrickariel
Fixing text sizes for examples authored by tjlaxs
Rename push children to add children authored by nealtaylor98
Note on compiling on FreeBSD authored by adrylain
Don't leak SEND resource, even if thread is panicking. authored by navneetankur
Fix floating point math authored by BenjaminBrienen
Group `IntoSystemConfigs` `impl`s together authored by ItsDoot
Add module and supporting documentation to `bevy_assets` authored by alice-i-cecile
Add basic docs explaining what asset processing is and where to look authored by alice-i-cecile
Use `FloatOrd` for sprite Z comparison and ignore sprites with NaN authored by jnhyatt
Add `DynamicTyped` link to `TypeInfo` docs (#15188) authored by Wcubed
Implement `enabled` flag for fps overlay authored by no-materials
Rename Add to Queue for methods with deferred semantics authored by BenjaminBrienen
Add `EntityRefExcept` and `EntityMutExcept` world queries, in preparation for generalized animation. authored by pcwalton
bevy_reflect: Add `Reflectable` trait authored by MrGVSV
Throw real error messages on all failed attempts to get `StateTransition` schedule authored by icorbrey
Enable `clippy::check-private-items` so that `missing_safety_doc` will apply to private functions as well authored by 13ros27
Allow `bevy_utils` in `no_std` Contexts authored by bushrat011899
Implement percentage-closer soft shadows (PCSS). authored by pcwalton
Fix typo in sprite_picking.rs example authored by mgi388
command based entry api with `EntityCommands::entry` authored by atornity
Remove allocation in `get_short_name` authored by bushrat011899
Fix memory leak in world's command queue authored by hymm
Added `HeadlessPlugins` (#15203) authored by Wcubed
Fix subtle/weird UB in the multi threaded executor authored by SkiFire13
AssetServer LoadState API consistency authored by crvarner
Cleanup legacy code from bevy_sprite authored by s-puig
simplify std::mem references authored by BenjaminBrienen
Add ASCII art to custom mesh example (#15261) authored by Wcubed
Remove int2ptr cast in `bevy_ptr::dangling_with_align` and remove `-Zmiri-permissive-provenance` in CI authored by SkiFire13
Various picking bugfixes authored by NthTensor
Broaden "Check for bevy_internal imports" CI Task authored by bushrat011899
Choose more descriptive field names for `ReserveEntitiesIterator` authored by RobWalt
bevy_reflect: Add `FunctionRegistry::call` authored by MrGVSV
Fix warnings triggered by `elided_named_lifetimes` lint authored by VitalyAnkh
Allow to expect (adopted) authored by richchurcher
`RenderUiSystem::ExtractTextureSlice` authored by ickshonpe
Fix doc link import style to avoid unused_imports authored by Shadowcat650
change return type of `World::resource_ref` to `Ref` authored by atornity
move ShortName to bevy_reflect authored by BenjaminBrienen
Want to contribute to Bevy?
Here you can find two types of potential contribution: Pull Requests that might need review and Issues that might need to be worked on.
Pull Requests Opened this week
bevy_reflect: Replace "value" terminology with "opaque" authored by MrGVSV
Shadow Hierarchies (aka fragments/auxilary nodes) authored by villor
bevy_reflect: Add `ReflectRef`/`ReflectMut`/`ReflectOwned` convenience casting methods authored by MrGVSV
Update ``glam`` to 0.29, ``encase`` to 0.10. authored by targrub
Reduce runtime panics through `SystemParam` validation authored by MiniaczQ
Allow a closure to be used as a required component default authored by fluffiac
Fix transparent_window example on wayland authored by mahkoh
UI Scrolling authored by Piefayth
Added Mesh::ATTRIBUTE_POSITION_2D (VertexFormat::Float32x2) authored by brianreavis
Reduce memory usage in component fetches and change detection filters authored by ItsDoot
Allow animation clips to animate arbitrary properties. authored by pcwalton
Add `core` and `alloc` over `std` Lints authored by bushrat011899
The Cooler 'Retain Rendering World' authored by Trashtalk217
WIP: Add UI `GhostNode` authored by villor
bevy_reflect: Add dynamic type data access and iteration to `TypeRegistration` authored by MrGVSV
Split `TextureAtlasSources` out of `TextureAtlasLayout` and make `TextureAtlasLayout` serializable authored by clarfonthey
`impl_reflect!` for EulerRot instead of treating it as an opaque value authored by aecsocket
Issues Opened this week
`bevy_color`'s operations are not stable across all hardware due to use of trigonometric float methods authored by BenjaminBrienen
Add an option to disable FpsOverlayPlugin authored by Shatur
StateScoped entity could cause "Could not despawn entity" warning authored by Shatur
Add fake variadics to `Reflect` impls authored by janhohenheim
GLTF error message could be more informative authored by viridia
Add example demonstrating how to achieve cross-platform deterministic math authored by alice-i-cecile
Dynamic queries with optional components authored by Occuros
Register asset saver? authored by JMS55
Way to disable renderer for specific window. authored by entropylost
Asset processing cannot be configured from code authored by alice-i-cecile
Panic loading GLB file authored by viridia
I am getting a stack overflow error from loading too many animations at once authored by victor7543
Move methods on `Children` that mutate to `EntityCommands` authored by ItsDoot
WindowResized events don't trigger authored by Pnoenix
Systems should be skipped if their resources cannot be fetched authored by alice-i-cecile
Introduce `QuerySingle<Q, F>` family of system parameters authored by MiniaczQ
Extrudable not implemented for Polygons authored by AnjanaYEAH
UnsafeWorldCell::world_metadata is unsound given its safety contract authored by Victoronz
Filtered lifecycle observers authored by alice-i-cecile
Minimizing window in the `irradiance_volume` example causes a crash authored by cryscan
despawn_recursive causing Tracy error authored by ConnorKnack
Support KHR_texture_transform per texture authored by jf908
bevy_ui: Implement Val::ObjectFit for width and height fields on bevy_ui::ui_node::Style authored by vveisard
Move `bevy_hierarchy` back into `bevy_ecs` authored by alice-i-cecile
`create_handle_internal is too complex authored by alice-i-cecile
Introduce `NonEmptyQuery` system param authored by MiniaczQ
add a no-std platform target to CI authored by BenjaminBrienen
error: linking with `cc` failed: exit status: 1 authored by nixon-voxell
Fix `ObserverSystem` docs authored by ItsDoot
Allow filters to take a `Bundle` instead of a singular `Component`, and make the conjunction behavior configurable authored by ItsDoot
Replace `Trigger`'s default bundle filtering behavior from an OR to an AND authored by ItsDoot
Use `StableInterpolate` in the camera examples authored by alice-i-cecile
`OverflowAxis::Clip` allows UI node contents to spill over the border authored by Piefayth
`ShortName` should work without depending on bevy_reflect authored by BenjaminBrienen
Feature request: `EntityWorldMut::take_by_id`(`s`) authored by urben1680
Asset locking authored by andriyDev
Bevy app with `pbr_transmission_textures` crashes on macOS/Metal after PCSS PR merge authored by coreh