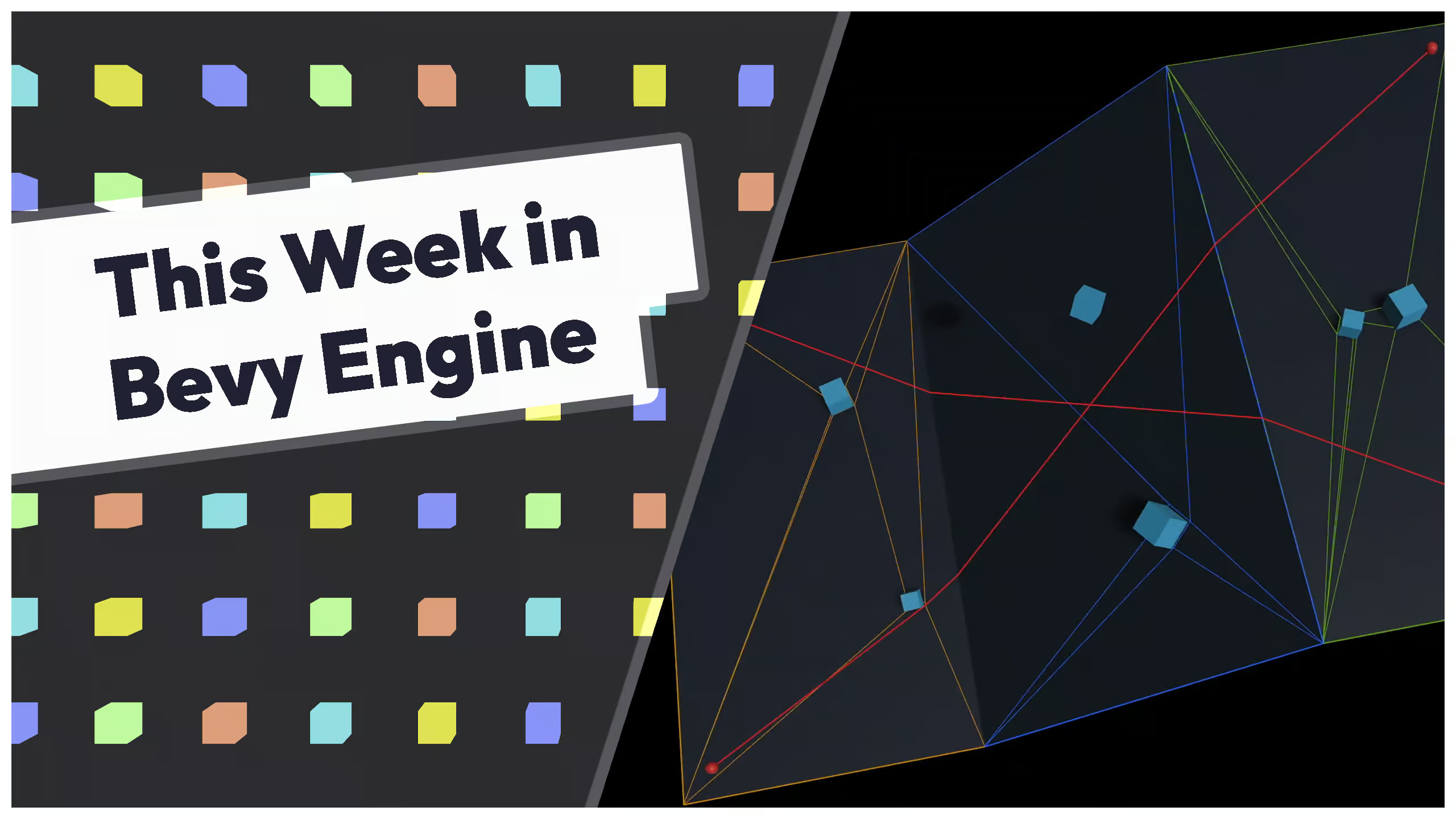
0.14.2, Compute Shaders, and Simulations
2024-09-09
0.14.2 is out! Including fixed for UI elements, gizmos, hue mixing, and more. See the full list over on GitHub.
Animations also get a boost this week with Animation Masks, a major part of a highly desired feature. We also see progress in the curves and picking working groups as well as some 0.14.2 UI fixes! And while we won't cover performance this week in depth, don't forget about #14673 which opportunistically used dense iteration for a 3.5x speedup in hybrid parallel iteration.
There are a number of compute shader showcases this week, including shallow water, particle life, and Nannou's new compute abstractions.
Animation Masks
The lack of masking bones is one of the most commonly requested missing features in the AnimationGraph
implementation in 0.14. As of #15013 support for mask groups has been implemented, which allows toggling animation effects on and off for specific groups of bones.
Note that additive blending of animations is not included in this PR, although it is intended to come next. Additive blending is the feature that would allow for a humanoid walking animation that affected the legs, and a punching animation that affected the upper body, to blend together and apply at 100% for their respectively masked affected parts.
You can check out the new animation_masks example to see it in action.
ShaderStorageBuffer
#14663 adds a fantastic new feature to allow creating a storage buffer without needing to access the RenderDevice
. This is useful for sharing the same buffers between compute and material shaders and fixes a cowpath where previously it was easier to do it in a less optimal way.
Check out the new shader/storage_buffer
example which shows how to take advantage of the new ShaderStorageBuffer
asset.
System Ordering Ambiguities
Remove all existing system order ambiguities in DefaultPlugins.
Systems run in parallel, but their order isn't always deterministic. The nondeterministic_system_order example already shows this, #13950 adds ambiguity_detection tests in CI, and #15031 removes all system order ambiguities from DefaultPlugins
, resulting in fewer bugs and cleaner diagnostic output
UI Fixes
A number of UI fixes were merged, especially around slicing. Some of these are in 0.14.2.
- Resolve UI outlines using the correct target's viewport size
- UI texture atlas slice shader
- UI outlines radius
- UI texture slice texture flipping reimplementation
bevy_mod_picking the upstreamening
Ignore clicks on uinodes outside of rounded corners
Curve Crew
#14976 introduces new color interpolation techniques using the new Curve
functionality to power a ColorCurve
. This allows interpolating between multiple colors and using the fancy new curve apis.
let gradient = ColorGradient::new([
basic::RED,
basic::LIME,
basic::BLUE
]).expect("non-empty");
let curve = gradient.to_curve();
let brighter_curve = curve.map(|c| c.mix(&basic::WHITE, 0.5));
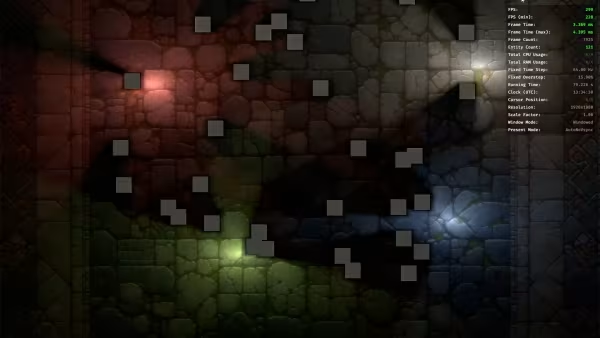
Showcase
Bevy work from the #showcase channel in Discord and around the internet. Use hashtag #bevyengine.
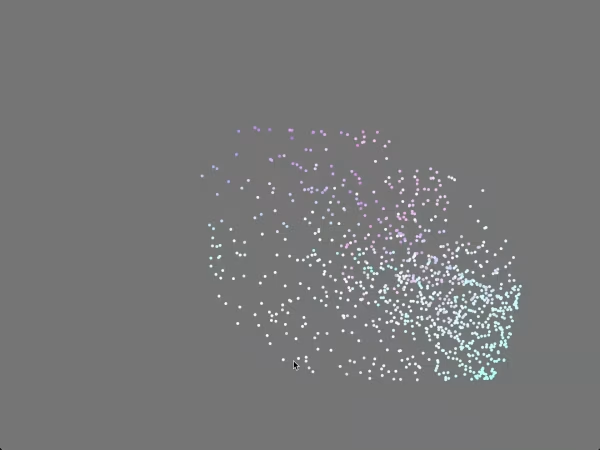
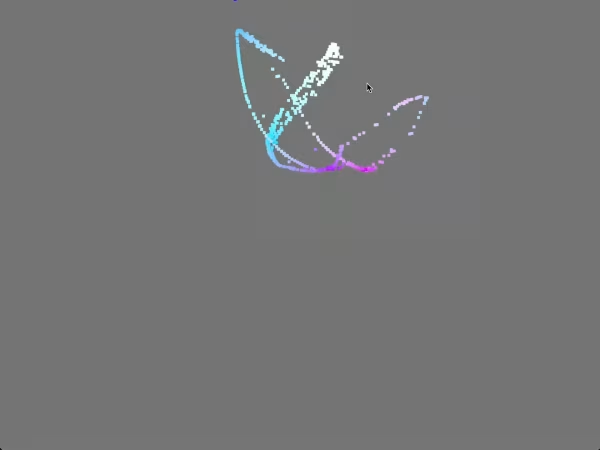
Bevy + Nannou Compute Shaders
showcase
This demo shows off newly added support for compute shaders to Nannou. This is a particle system written in a 200 line sketch.
The sketch is really interesting and includes a compute shader abstraction that is somewhat similar to Bevy's Material.
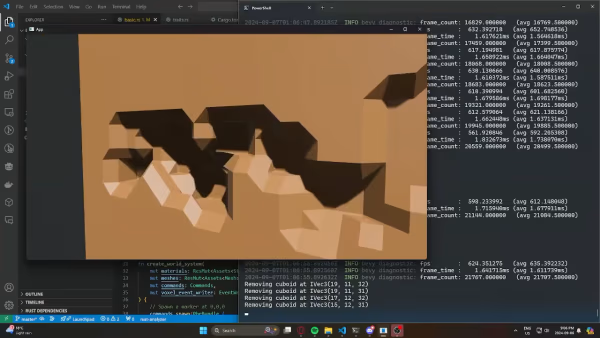
Marching cubes
showcase
This implementation of marching cubes is implemented on the CPU and swaps out the mesh and collision entirely. It's usually ~1-2ms to recompute the mesh of this size (32x32x32)
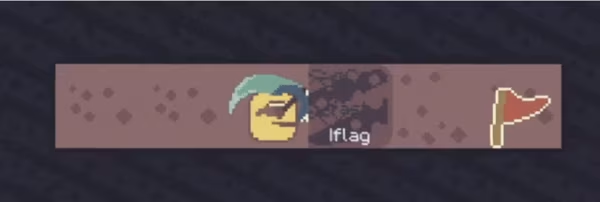
typing+mining prototype
showcase
early progress on a new type-to-mine game. Type words to mine blocks and make it to the flag.
The camera shake is powered by bevy_camera_shake
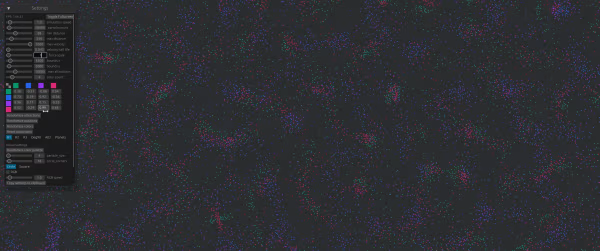
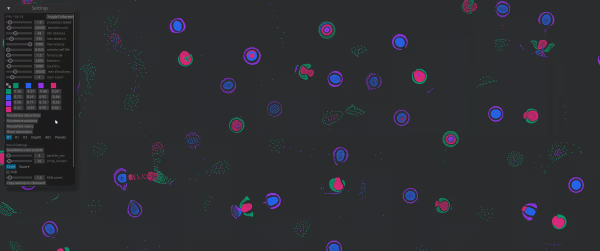
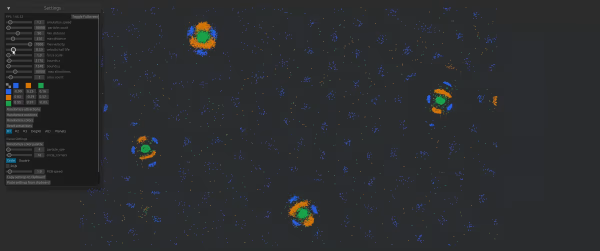
Particle Life
showcase
Particle Life is a color based particle simulation: a color may either attract, repel or be neutral towards each of the other colors. However, this relation is asymmetric, so for example blue may attract red, but red may be neutral or repel blue. 60fps with 300k particles, 20k bounds and 300 max distance.
Source is available on GitHub and a video is available on YouTube
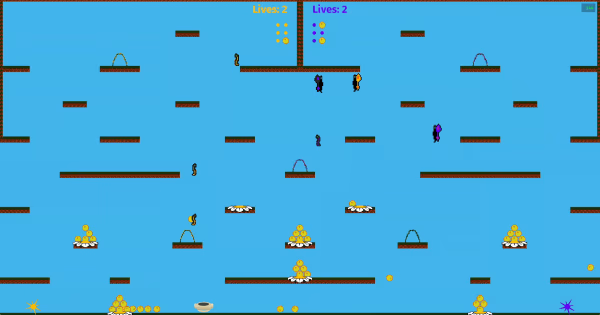
Killer Queen
showcase
A fast-paced, 4-10 player, local multiplayer, arcade-like 2d platformer inspired by the addictive arcade game Killer Queen
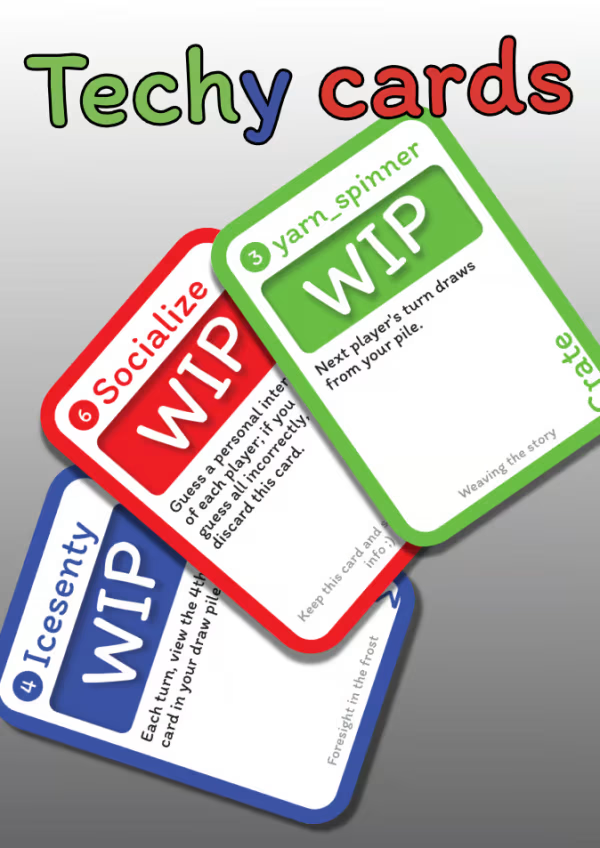
Bevy-themed Physical Card Game
showcase
A Bevy-themed physical card game!
The goal of the game is to combine 3 types of cards in a short session, to get the most points:
- Members: "relevant" actors from bevy ecosystem
- Crates: bevy-relevant dependencies
- Techniques: whatever makes a project work
Development of the project will be open sourced, and physical packs might happen one day.
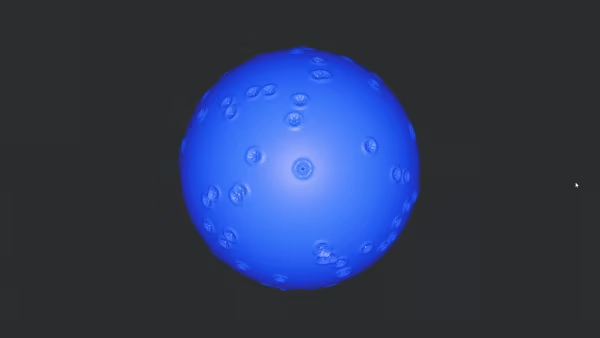
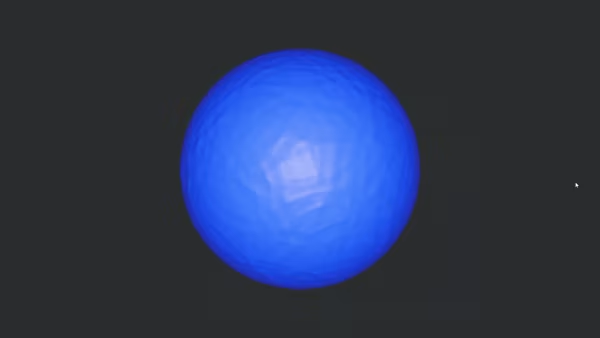
Shallow Water Simulation
showcase
A port of spherical shallow water simulation to compute shaders with Bevy. High resolution working just fine at 60fps
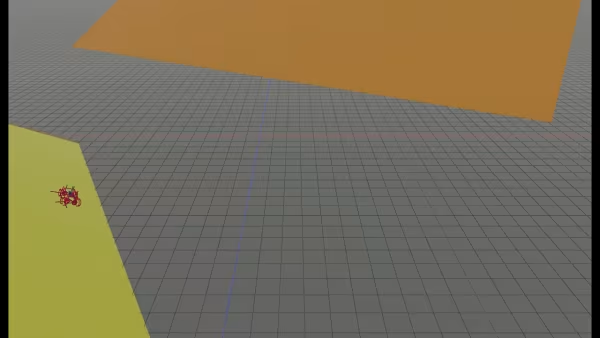
Vehicle Joints
showcase
Progress on a vehicle physics implementation using Rapier. The code is originally from the vehicle_joints3
example from the rapier repository.
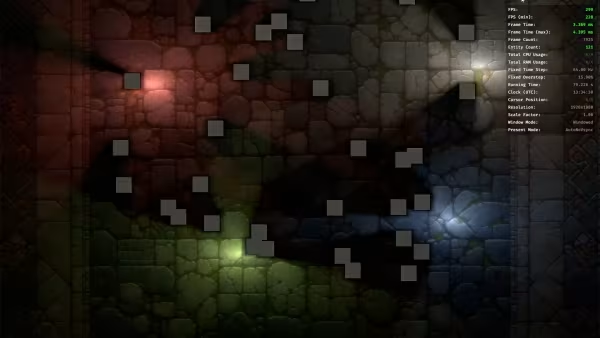
Crates
New releases to crates.io and substantial updates to existing projects
bevy_simplenet v0.13.0
crate_release
bevy_simplenet
is a networking crate based on websockets. Supports native and WASM clients.
- Added
AuthToken
for client authentication. This was the last big missing feature. - Added ability to construct servers with a pre-defined
axum
router. - Removed
nightly
dependency.
Bevy Replicon 0.28.1
crate_release
bevy_replicon
is a crate for server-authoritative networking.
0.28.1 adds:
- Added the ability to defer replication, which is useful for exchanging messages or downloading assets required by the server before replication starts.
- If there is any spawning, despawning, removal, or insertion, client events wait for replication. However, with this release, it can be disabled per event.
- Entity mapping when a client event is buffering is fixed.
The full changelog is available on GitHub
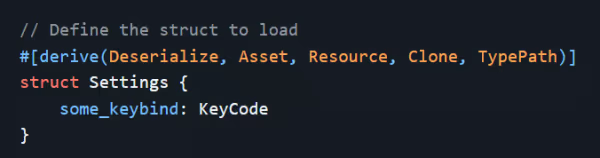
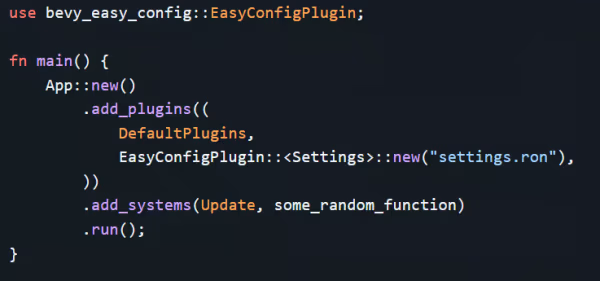
bevy_easy_config v0.2.0
crate_release
bevy_easy_config
allows you to automatically load structs at startup, from the asset folder, by simply deriving the proper traits, and adding it with the bevy easy config plugin. Now the struct is available from anywhere in your code as a resource, and it even hot reloads!
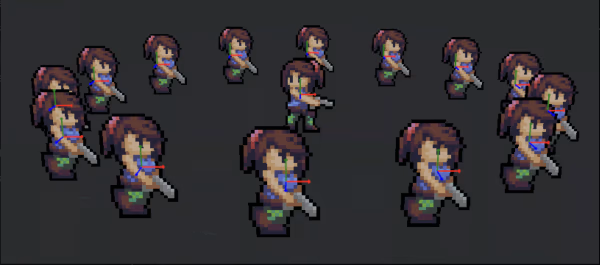
bevy_spritesheet_animation 0.4.2
crate_release
bevy_spritesheet_animation
is a Bevy plugin for animating sprites that are backed by spritesheets
0.4.2 adds:
- 3D sprites are now supported (both static and animated) 🎉
- Sprite playback can now be manually controlled if needed (eg. "go to frame 3", "reset that animation", ...)
- The high-level API has been made simpler and more concise so that it's a breeze to configure animations
- Various optimizations have sped up playback by a factor of about 3
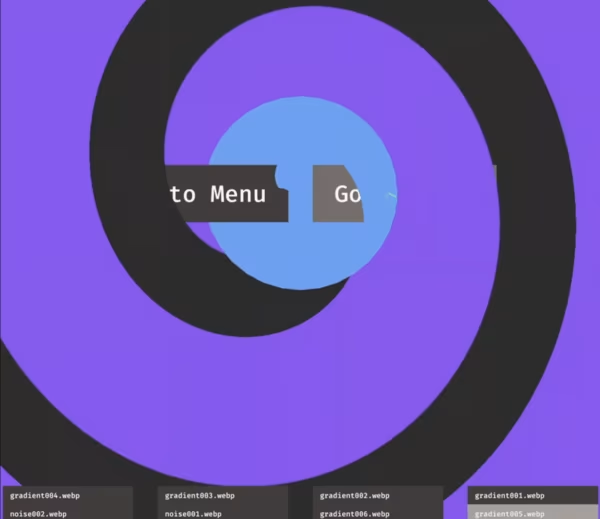
bevy_2d_menu_mask_transition
crate_release
bevy_2d_menu_mask_transition release
is a plugin for creating smooth menu transitions with customizable masks.
Interpolate `WorldQuery` path in docs of generated types authored by no-materials
Optimize UI text measurement authored by UkoeHB
Color gradient curve authored by RobWalt
Cosmetic improvements for `text_input` example authored by rparrett
Fix some examples having different instruction text positions authored by rparrett
Extract borders without border radius authored by ickshonpe
Reflect `SmolStr`'s `De/Serialize` implementation authored by bushrat011899
Use cosmic-text shaping buffer authored by UkoeHB
Don't reallocate scale factors in measure_text_system authored by UkoeHB
Reuse TextLayoutInfo in queue_text authored by UkoeHB
Avoid reallocating spans buffer in TextPipeline authored by UkoeHB
Implement animation masks, allowing fine control of the targets that animations affect. authored by pcwalton
Resolve UI outlines using the correct target's viewport size authored by ickshonpe
Use `#[doc(fake_variadic)]` for `SystemParamBuilder` tuple impls. authored by chescock
Adds `ShaderStorageBuffer` asset authored by tychedelia
Reduce allocations in ui_layout_system authored by UkoeHB
UI texture atlas slice shader authored by ickshonpe
Fix `with_child` not inserting `Parent` component authored by BigWingBeat
Updated `LogPlugin` Documentation with Performance Warning authored by bushrat011899
Bump crate-ci/typos from 1.24.1 to 1.24.3 authored by mnmaita
Ignore clicks on uinodes outside of rounded corners authored by ickshonpe
style: simplify string formatting for readability authored by hamirmahal
Opportunistically use dense iter for archetypal iteration in Par_iter authored by re0312
Remove all existing system order ambiguities in `DefaultPlugins` authored by alice-i-cecile
Return `Result`s from `Camera`'s world/viewport conversion methods authored by chrisjuchem
Utilise new method for 2d circle example. authored by FarmingtonS9
UI outlines radius authored by ickshonpe
Picking event ordering authored by NthTensor
UI texture slice texture flipping reimplementation authored by ickshonpe
Fix `bevy_ui` compile error when `bevy_picking` feature is disabled authored by BigWingBeat
`Node::is_empty` authored by ickshonpe
Revert accidentally added asset docs authored by alice-i-cecile
fix imports in example ui_texture_slice_flip_and_tile authored by mockersf
Want to contribute to Bevy?
Here you can find two types of potential contribution: Pull Requests that might need review and Issues that might need to be worked on.
Pull Requests Opened this week
More triangles/vertices per meshlet authored by JMS55
Trim cosmic-text's shape run cache authored by UkoeHB
Rename rendering components for improved consistency and clarity authored by Jondolf
Reflect auto registration authored by eugineerd
Add method to remove component and all required components for removed component authored by rewin123
Stop using `Handle<Scene>` and `Handle<DynamicScene>` as components authored by tim-blackbird
Add convenience methods for constructing and setting storage buffer data authored by tychedelia
Honor mesh-light interaction mediation for “low” render layers authored by coreh
Use associated type bounds for `iter_many` and friends authored by tim-blackbird
Remove remnant `EntityHash` and related types from `bevy_utils` authored by ItsDoot
Sorts the scene entries by path before serializing. authored by Wiwip
Add module and supporting documentation to `bevy_assets` authored by alice-i-cecile
Add basic docs to AssetMode authored by alice-i-cecile
Add basic docs explaining what asset processing is and where to look authored by alice-i-cecile
Remove deprecated `SpriteSheetBundle` and `AtlasImageBundle` authored by benfrankel
remove cfg-check in ci tool authored by mockersf
Simplify `pick_rounded_rect` authored by CrazyboyQCD
Fix `AsBindGroup` sampler validation. authored by tychedelia
Add `many_entities_mut` function variants to `DeferredWorld` authored by ItsDoot
Add `observer` to `Trigger` authored by bushrat011899
Meshlet screenspace-derived tangents authored by JMS55
bevy_reflect: Add crate level `functions` feature docs authored by nixpulvis
Add state scoped events authored by UkoeHB
Add set_state extension method to Commands authored by UkoeHB
Fix error link authored by rparrett
Unify crate-level preludes authored by BD103
Register reflect type `CursorIcon` authored by eero-lehtinen
chore(readme): enhance warning authored by realguse
Remove OrthographicProjection.scale (adopted) authored by richchurcher
bevy_reflect: Function Overloading (Generic & Variadic Functions) authored by MrGVSV
Split OrthographicProjection::default into 2d & 3d (Adopted) authored by Azorlogh
Issues Opened this week
Incorrect Time resource for fixed systems run with World::run_schedule(FixedMain) authored by nick-e
Camera's near plane clips into FogVolume when positioned near its edge authored by jirisvd
Crash on Camera Initialization authored by WilliamLin062
Window resizing glitches on MacOS authored by UkoeHB
Move sprite 9 patches to a shader to avoid thin lines authored by alice-i-cecile
Replace "system order ambiguity" terminology to make it more clear it's a race condition authored by ItsDoot
Expose a way to manually run startup schedules authored by torsteingrindvik
bug: cannot create an unfocused window on Mac for dev authored by swarnimarun
A UI node size of zero shouldn't be used as a proxy to indicate that a node has `Display::None` set authored by ickshonpe
Add `many_entities_mut` function variants to `DeferredWorld` authored by ItsDoot
Swap most uses of allow in lints to expect authored by alice-i-cecile
FogVolume planes are rendered incorrectly when looked at from certain angles authored by jirisvd
The doc for `DynamicStorageBuffer` doesn't really explain the difference with `StorageBuffer` authored by djeedai
Segmentation fault on loading 3d examples authored by Will-Carson
Expose Trigger::ObserverTrigger::observer authored by alexparlett
Deprecate `LoadAndSave` asset processor authored by alice-i-cecile
StateScoped should support OnRemove observers authored by UkoeHB
Separate Changed<T> and Added<T>, readding `Mutated` query filter authored by mdeering24
Document which GLTF extensions are (un)supported authored by torsteingrindvik
Materials with slow loading textures never update the bind group after loading authored by JMS55
game_menu example doesnt change button colour with mouse interaction authored by Liam19
DX12 backend panics when window is resized authored by akimakinai