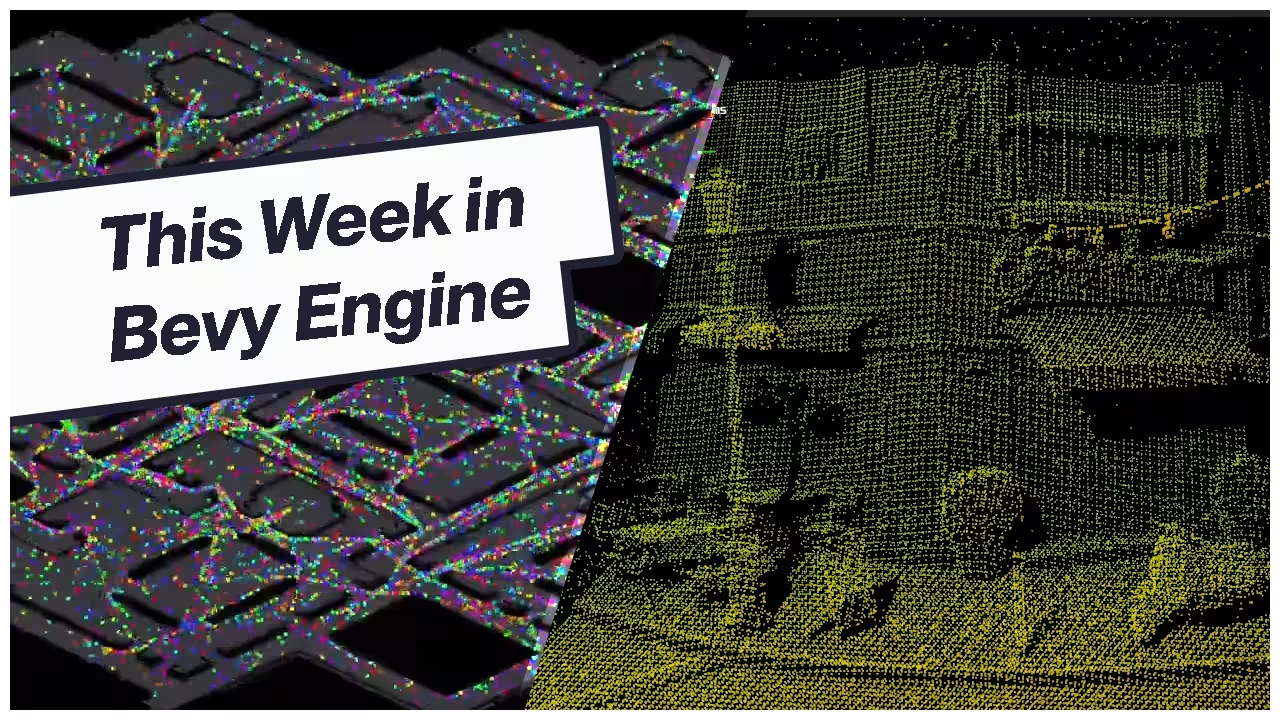
Meshlets, Stable Interpolation, and Generalized ECS Reactivity with Observers!
2024-06-17
Release Candidate 0.14.0-rc.3 is out this week with one last new feature for 0.14 (Observers), an upgrade to wgpu 0.20 (previously 0.19), as well as assorted fixes for rc.2.
With the 0.14 release getting closer fewer major features are getting merged but we still have some great functionality to talk about.
As always you can keep up to date with the release using the 0.14 milestone.
Observers
#10839 (Generalised ECS reactivity with Observers) introduces the idea of Observers to Bevy. Fundamentally Observers seem like an infrastructure-level improvement to the ECS implementation in Bevy. They are absolutely usable in application-level code but personally I'm looking forward to see how they develop and what gets built on top of them over the next few releases.
Observers can use systems with all the dependency injection you'd expect as well as a new Trigger
type. Observers can be "global" or watch specific entities.
#[derive(Event)]
struct Speak {
message: String,
}
world.observe(|trigger: Trigger<Speak>| {
println!("{}", trigger.event().message);
});
// Observers currently require a flush() to
// be registered. In the context of schedules,
// this will generally be done for you.
world.flush();
world.trigger(Speak {
message: "Hello!".into(),
});
Virtual Geometry in Bevy 0.14
The upcoming experimental meshlets feature in Bevy 0.14 is comparable to Unreal Engine's Nanite (PDF) in purpose. The primary author of the feature in Bevy (jms55) wrote an in-depth post titled Virtual Geometry in Bevy 0.14 which is a fantastic read for anyone interested in the functionality meshlets provide. The post is less "how to use meshlets" and more "how meshlets is implemented" and includes numerous code examples and screenshots showing off the functionality.
Custom Primitives
#13795 introduces a new example that shows off custom primitives and extrusions of those primitives. This is an interesting example because it shows off a lot of the primitive and meshing features that have developed over the last few months, including the Bounded, Measured, Meshable, and Extrusion traits.
Extrusion Segments
#13719 adds support for segments to the ExtrusionBuilder
Stable Interpolation
Freya Holmer recently gave a talk titled lerp smoothing is broken which then influenced an implementation of stable interpolation in #13741. The video and the PR are both top-notch documentation resources for what is going on here, so I'll leave you with the short version of StableInterpolate
is:
A type with a natural interpolation that provides strong subdivision guarantees.
2d top-down camera example
#12720 adds a new top-down 2d camera example shows off how to use lerp
to make a camera follow a player's movement.
Alice's Merge Train is a maintainer-level view into active PRs, both those that are merging and those that need work.
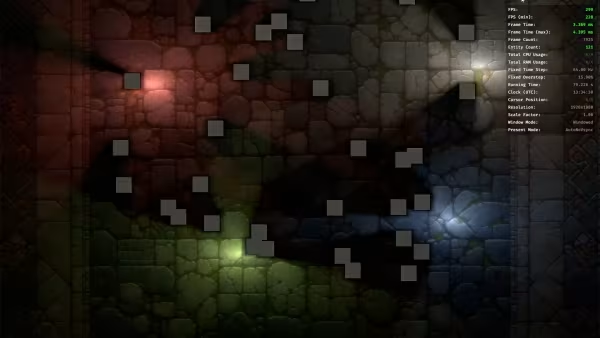
Showcase
Bevy work from the #showcase channel in Discord and around the internet. Use hashtag #bevyengine.
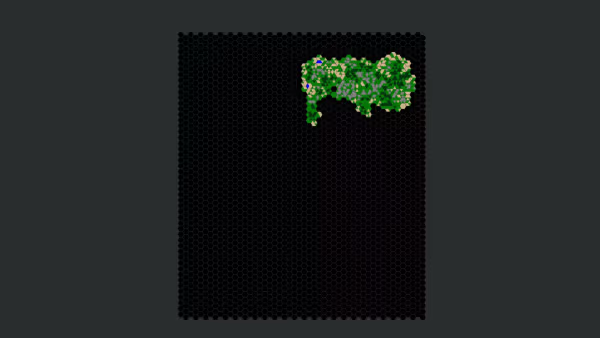
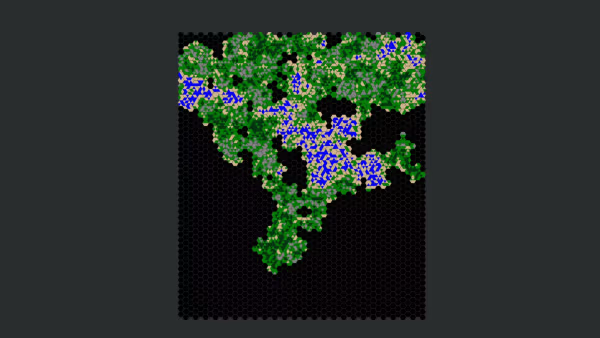
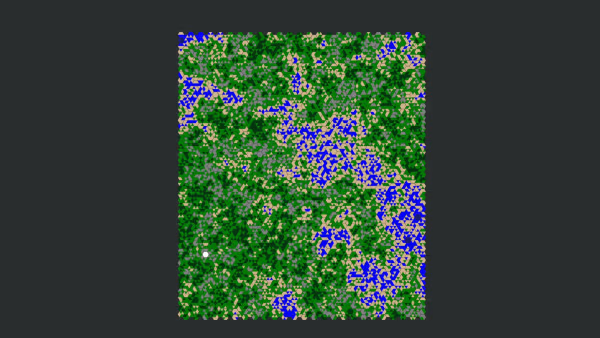
Wave Function Collapse on Hex Grids
showcase
This is a demo of an in-progress wave function collapse implementation. Here its generating in a hexagonal grid.
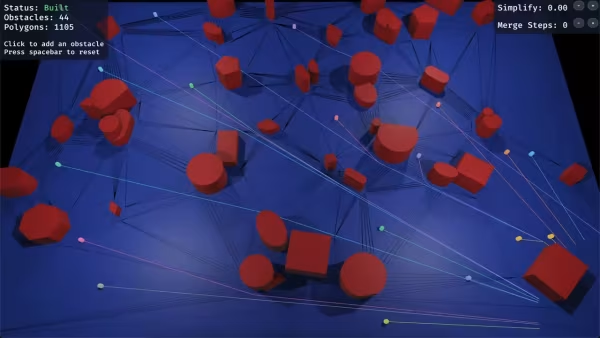
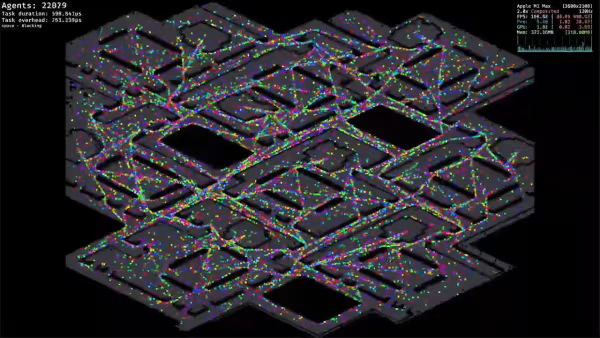
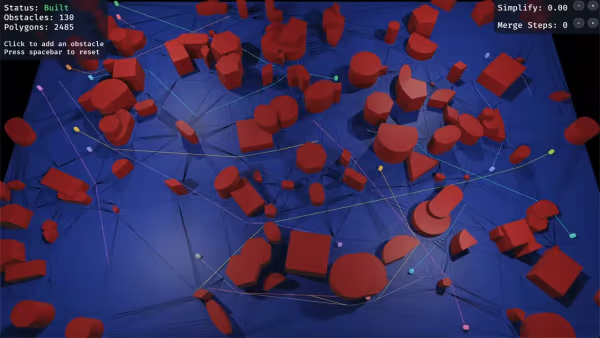
Navigation Meshes with Polyanya
showcase
This demo is preparation work for NavMesh pathfinding crate releases.
The first demo is spawning random obstacles made from extrude primitive shapes from Bevy, updating the NavMesh several times per second, and recomputing all paths at every update, all that running at 120fps. While the second demo shows off many different agent operating at once.
There is also a wasm-based demo you can run in the browser.
The code is on GitHub and is using a Polyanya implementation. Both repos have additional links to references should you want to understand what's happening at a deeper level.
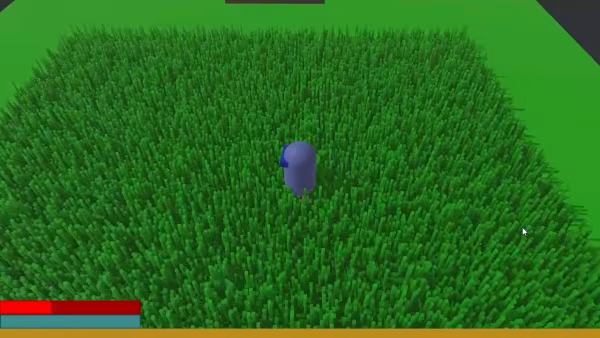
Grass/Fur
showcase
This is an implementation of grass inspired by the concepts in this Acerola video: "How Are Games Rendering Fur?".
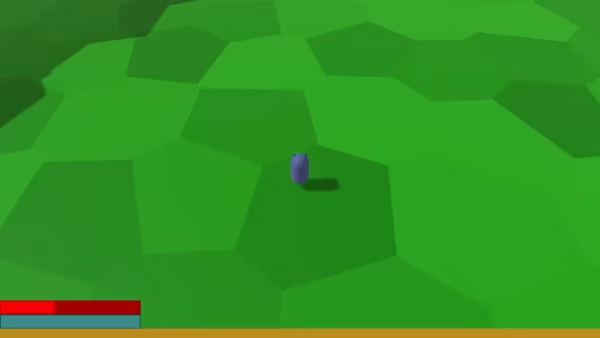
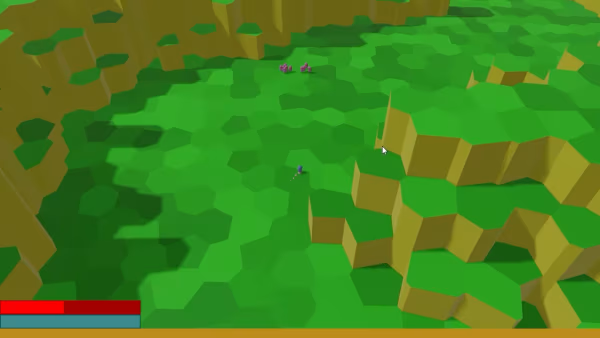
Map Generator
showcase
A map generator built from MarkovJunior grammar. The goal was to evoke walking through a big valley and the mesh generation and layout generation are separated. Layout and mesh generation as well as the MarkovJunior grammar are all covered in more depth in the Discord thread including some code samples.
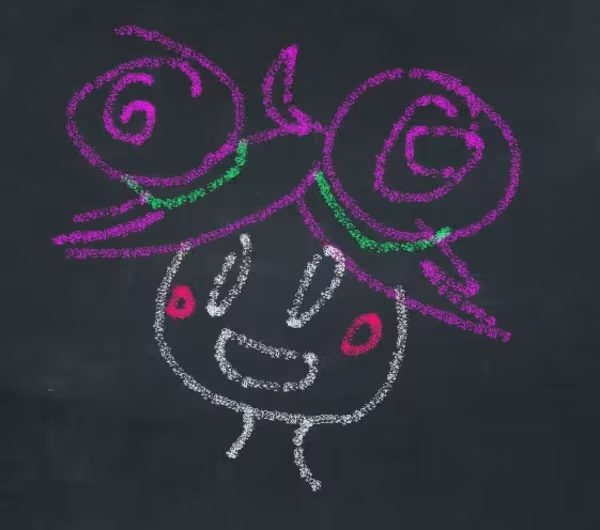
Chalkboard Audio
showcase
A chalkboard application that uses the mouse speed to adjust the sound of chalk hitting the chalkboard. It uses Bevy's native audio implementation.
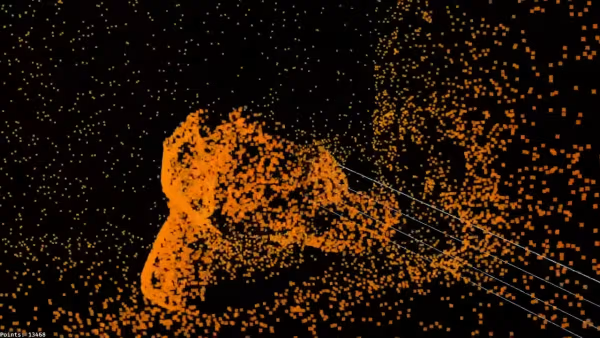
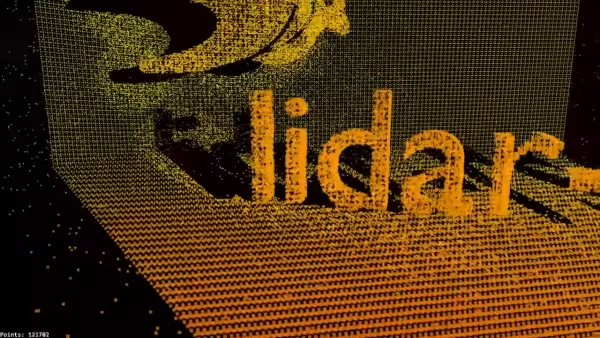
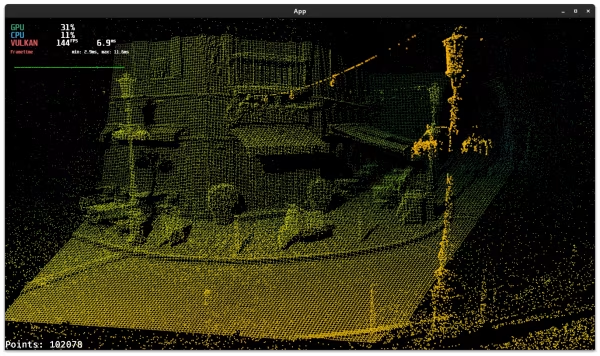
LIDAR
showcase
This LIDAR-style effect uses a custom render pipeline to render points and order-independent transparency to compose them which means no transparency sorting. The points are in a PointCloud
component and it respects culling.
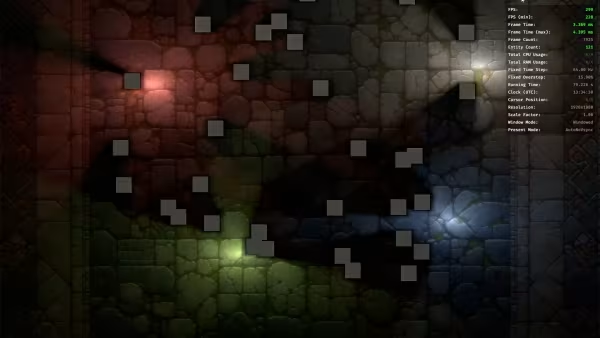
Crates
New releases to crates.io and substantial updates to existing projects
glam 0.28
crate_release
glam is a foundational crate when it comes to the Bevy ecosystem. This is probably most visible in the Vec types Bevy consumes and re-exports, like Vec3.
v0.28 brings AArch64 NEON SIMD support as well as a couple smaller breaking changes.
Its too late for this glam release to make it into Bevy 0.14 but the improvements should filter through the ecosystem (for example, encase) in time for 0.15.
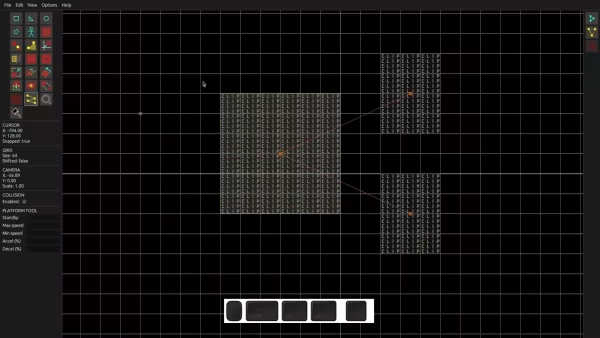
HillVacuum 0.2.5
crate_release
HillVacuum is a 2d map editor inspired by the TrenchBroom editor.
The releases add new documentation as well as the ability to edit texture animations independently of selected brushes.
bevy_svg_processor
crate_release
bevy_svg_processor enables working with .svg
s with the rendering simplicity and performance of raster images like .png
.
// Enable AssetMode::Processed
// and add the SvgProcessorPlugin
app.add_plugins((
DefaultPlugins.set(AssetPlugin {
mode: AssetMode::Processed,
..default()
}),
SvgProcessorPlugin::default(),
));
// Spawn your svg!
commands.spawn(SpriteBundle {
texture: asset_server.load("warrior.svg"),
..default()
});
bevy_scriptum 0.5
crate_release
bevy_scriptum adds scripting language support to Bevy, including Rhai and Lua.
0.5 brings the Lua support and introduces a documentation book
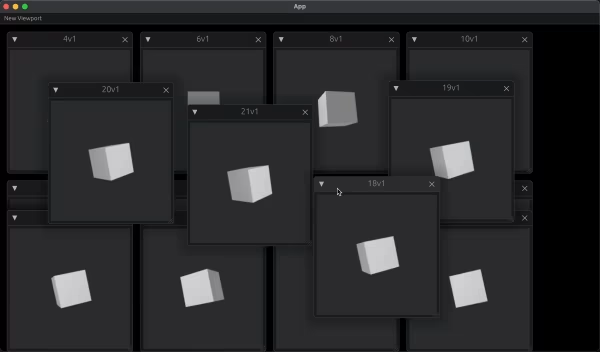
bevy_mod_picking 0.19
crate_release
bevy_mod_picking adds picking to Bevy. If you want to hover, drag, or click entities in your Bevy App then you're looking at the right crate. There are ongoing efforts to upstream some of this functionality into Bevy.
0.19 introduces a new render_to_texture
example to show how to render viewport textures that support picking and relaxes the requirements for egui and rapier, allowing the use of a wider range of compatible crates.
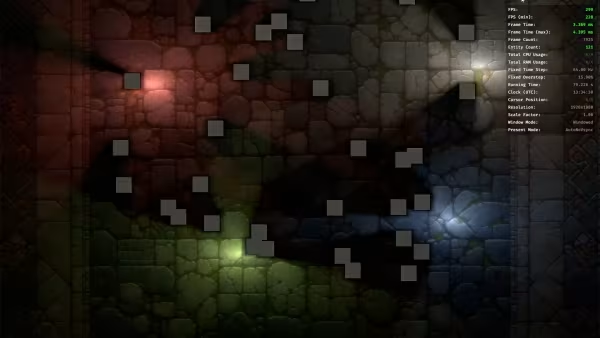
Educational
Tutorials, deep dives, and other information on developing Bevy applications, games, and plugins
Bevy Cheatbook updates
educational
A bunch of Bevy Cheatbook updates in various areas. New updates for Bevy 0.14 are under way as well.
- Pan/Orbit Camera
- Transform Interpolation/Extrapolation: How to get smooth-looking movement on-screen for things you simulate in
FixedUpdate
- Internal Parallelism: Multithreaded iteration
- One-Shot Systems
- Background Computation
Using tracing to profile a Bevy project
educational
This post covers Bevy's built-in capabilities around using tracing and outputting chrome-compatible traces.
Fix EntityCommands::despawn docs authored by thebluefish
view.inverse_clip_from_world should be world_from_clip authored by ChristopherBiscardi
Clear messed up feature flag on AppExitStates impl authored by bugsweeper
Re-name and Extend Run Conditions API authored by CupOfTeaJay
Added an illustration to the compass direction docs (issue 13664) authored by Wuketuke
Remove extra call to clear_trackers authored by chrisjuchem
Align `Scene::write_to_world_with` to match `DynamicScene::write_to_world_with` authored by dmyyy
Fix meshlet vertex attribute interpolation authored by JMS55
Poll system information in separate tasks authored by Brezak
Revert Align Scene::write_to_world_with to match DynamicScene::write_to_world_with authored by alice-i-cecile
Custom primitives example authored by lynn-lumen
Split event.rs into a full module. authored by BobG1983
Add `from_color` to `StandardMaterial` and `ColorMaterial` authored by Brezak
Revert "Make FOG_ENABLED a shader_def instead of material flag (#13783)" authored by alice-i-cecile
Improve error handling for `AssetServer::add_async` authored by JoJoJet
Update serialize flag for bevy_ecs authored by cBournhonesque
2D top-down camera example authored by theredfish
Clarify error message due to missing shader file authored by imrn99
fix docs around `StateTransition` and remove references to `apply_sta… authored by lee-orr
Add more granular system sets for state transition schedule ordering authored by MiniaczQ
Meshlet misc authored by JMS55
Stable interpolation and smooth following authored by mweatherley
Adds back in way to convert color to u8 array, implemented for the two RGB color types, also renames Color::linear to Color::to_linear. authored by gagnus
Remove unused mip_bias parameter from apply_normal_mapping authored by JMS55
Add segments to `ExtrusionBuilder` authored by lynn-lumen
Make FOG_ENABLED a shader_def instead of material flag authored by IceSentry
Let init_non_send_resource require FromWorld instead of Default authored by NiklasEi
Fix minor doc typo authored by janhohenheim
Revert "constrain WorldQuery::init_state argument to ComponentInitial… authored by hymm
Fix minor typos in query join docs authored by CatThingy
Ensure that events are updated even when using a bare-bones Bevy App authored by alice-i-cecile
Highlight dependency on shader files in examples authored by kornelski
run windows ci on rust 1.78 authored by mockersf
Ensure that events are updated even when using a bare-bones Bevy App (#13808) authored by mnmaita
Restore overwrite capabilities of `insert_state` authored by MiniaczQ
fix non-exact text h-alignment authored by robtfm
Mention updating Bevy book code validation for release candidates authored by BD103
Wgpu 0.20 authored by Elabajaba
reduce the antialias strength authored by hymm
Use dynamic uniform buffer in post processing example authored by jgayfer
Add missing plugins to doc of DefaultPlugins authored by MonaMayrhofer
Fix is_plugin_added::<Self>() being true during build authored by kristoff3r
Upstream `CorePlugin` from `bevy_mod_picking` authored by NthTensor
Generalised ECS reactivity with Observers authored by james-j-obrien
Want to contribute to Bevy?
Here you can find two types of potential contribution: Pull Requests that might need review and Issues that might need to be worked on.
Pull Requests Opened this week
Assetv2: Unprocessed asset meta defaults. authored by brandon-reinhart
Print warning when using llvmpipe authored by msvbg
Sample `Transform` from an `AnimationClip` authored by mintlu8
Add example enum Component usage to ecs_guide authored by theotherphil
add helper method Query::with_entity authored by Nathan-Fenner
Implement the infrastructure needed to support portals and mirrors. authored by pcwalton
derive(SystemParam) better lifetime param (#10331) authored by Wuketuke
Update to glam 0.28 authored by superdump
Use a unstable sort to sort component ids in `bevy_ecs` authored by Brezak
AssetPack syntax sugar for static assets authored by ecoskey
Created an EventMutator for when you want to mutate an event before reading authored by BobG1983
Fix meshlet interactions with regular shading passes authored by JMS55
Added peeking to EventReader authored by BobG1983
Checking points when creating CubicBezier (#13726) authored by Wuketuke
Add Mac OS tracy info to profiling docs authored by mweatherley
add debug logging to ascertain the base path the asset server is using authored by alphastrata
Fix to events par_read bug 13821 authored by BobG1983
Made some things in bevy_render `Debug`. authored by mintlu8
Add first person view model example authored by janhohenheim
Update accesskit and accesskit_winit authored by jdm
Implement omnidirectional cameras for real-time reflection probes. authored by pcwalton
Use a well defined type for sides in RegularPolygon authored by NiseVoid
Change `SceneInstanceReady` to trigger an observer. authored by komadori
text position: use size instead of bounds authored by mockersf
Adds `serde` derives to `CubicCurve` behind feature flag authored by mnmaita
Windows CI example runner: back to using rust stable authored by mockersf
remove inaccurate warning from `in_state` authored by lee-orr
Fix regression in #13714 authored by dmyyy
Issues Opened this week
First-party parallax backgrounds authored by alice-i-cecile
First-party tile maps authored by alice-i-cecile
Fog toggling shader flag is suboptimal authored by alice-i-cecile
Incorrect normals of ConicalFrustum authored by lynn-lumen
Tracking: `bevy-trait-query` breakage after changes to `WorldQuery` API authored by RobWalt
Add non-short-circuiting run conditions and re-rename short-circuiting run conditions to be explicit authored by alice-i-cecile
Add Boolean Operations on `Mesh`es authored by snailtomatoes
MeshletMesh not writing to the depth prepass authored by JMS55
MeshletMesh material textures not rendering sometimes authored by JMS55
MeshletMesh incorrect depth buffer handling authored by JMS55
Remake PRs targeting 0.14 branch authored by alice-i-cecile
AssetServer load docs should state that assets don't get loaded multiple times authored by torsteingrindvik
I hope to support iOS and Android input methods. authored by mzdk100
EventReader:par_read doesn’t mark events as read authored by BobG1983
Additive blending of Quats looks suspect authored by mweatherley
AlphaMode::Blend not depth testing authored by bananaturtlesandwich
Links to schedules and conditions are broken in various state docs authored by rparrett
SSAO does not work with orthographic projections authored by aevyrie
Incorrect WARN when using sub states authored by jonathandw743
Gamepad not giving back correct data when connected while app is running authored by SenneDeVocht
`CubicCurve` is missing serde support authored by torsteingrindvik
Doing `#[reflect(ignore)]` on fields in a struct still requires `Default` on that field authored by torsteingrindvik
Panic when log filters are incorrectly configured authored by rparrett
Update `QueryData` docs to mention that `Mut` implements it authored by Adamkob12
Add MainScheduleOrder::insert_before() authored by JMS55
Simple gizmo usage might trigger vulkan validation errors authored by torsteingrindvik
Observe should state that `Trigger<_>` must be first arg authored by torsteingrindvik