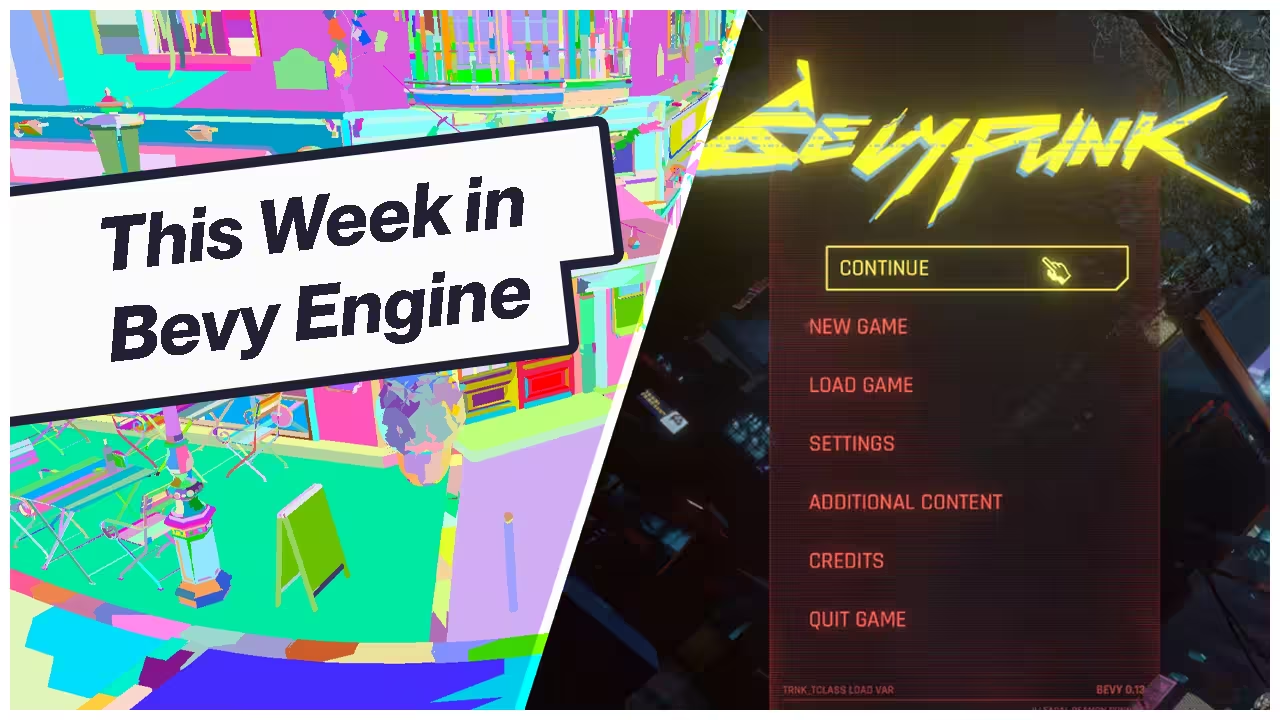
Cyberpunk UI, Minecraft simulation, and volumetric destruction
2024-05-27
This week in Bevy the ecosystem is going strong as the 0.14 release creeps closer.
The fourth unofficial Bevy meetup was livestreamed on May 24th including 3 talks.
- François gave a talk about Bevy's (and potentially your own) CI system titled Catching Rendering Regressions on all Platforms. This talk culminated in a call to test your own projects' targets, shaders, ui positioning, and more.
- Lorenz gave a talk about building your own
RenderPipelines
titled Hooking into the Bevy Rendering Pipeline. It came with a wonderful GitHub repo with working code. - Jos gave a talk about the project they had featured in the Bevy 0.12 release notes title Recreating Townscaper using Rust & Bevy. The talk covered a lot of what you would expect from a Townscaper-influenced topic including procedural generation and more. Demo of the project is available live at geofront.nl/project/spirit.
bevy_reflect custom attributes
Reflection in Bevy is used for a variety of applications. For example, bevy_inspector_egui
uses a custom macro to power inspector options.
#[derive(Reflect, Default, InspectorOptions)]
#[reflect(InspectorOptions)]
struct Slider {
#[inspector(min = 0.0, max = 1.0)]
value: f32,
}
Bevy could support this in its reflection capabilities and this PR does exactly that: enabling support for custom attributes. This reflected information is stored in type info and can be retrieved for use in editor or other code applications.
#[derive(Reflect)]
struct Slider {
#[reflect(@0.0..=1.0)]
value: f64,
}
let TypeInfo::Struct(info) = Slider::type_info() else {
panic!("expected struct info");
};
let field = info.field("value").unwrap();
let range = field.get_attribute::<RangeInclusive<f64>>().unwrap();
assert_eq!(*range, 0.0..=1.0);
Spherical linear interpolation
Dir3
and Dir2
got support for slerp
, aka "spherical linear interpolation", in #13451 which corresponds to interpolating between the two directions at a constant angular velocity.
Shapes
Sampling
Sampling was first introduced a couple months ago in #12484. This created the ShapeSample
trait which powers the uniform point sampling on bevy_math
's shapes. You can calculate randomized points contained by the shape's interior or on the surface boundary.
This week ShapeSample
got an implementation for sampling many points in a distribution instead of just one and the Annulus and Tetrahedron shapes got their sampling implementations.
Meshing
The Tetrahedron and ConicalFrustum got their mesh implementations.
New Primitives
Speaking of shapes, we also got some new primitives in Arc2d
, CircularSector
, and CircularSegment
.
and the Tetrahedron
(and the Triangle3d
) got added to the render_primitives
example.
Alice's Merge Train is a maintainer-level view into active PRs, both those that are merging and those that need work.
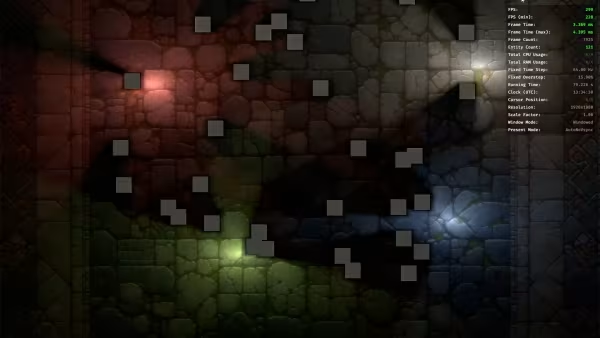
Showcase
Bevy work from the #showcase channel in Discord and around the internet. Use hashtag #bevyengine.
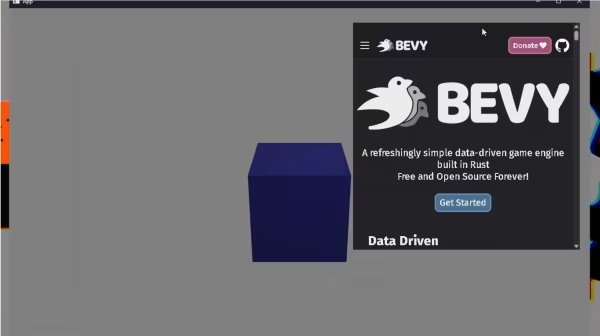
Bevy and Wry
showcase
Integrating Bevy and Wry to render webviews inside of Bevy apps.
This is an interesting approach that has been prototyped a few times going back at least 6 months since Tauri and Wry gained the ability to render multiple windows in one and interact via HasWindowHandle
. Tauri has an official example of this kind of approach and so does wry.
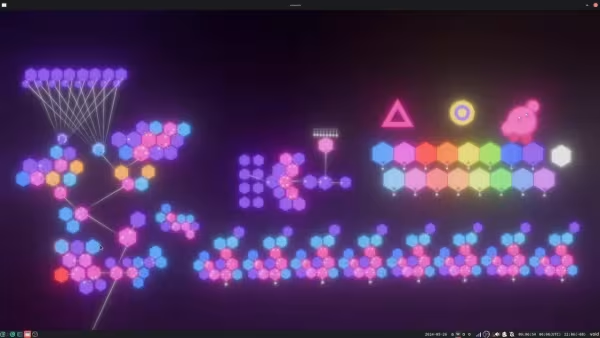
another quartz jam
showcase
quartz
is a visual programming and dsp playground. This demo is called "grace" and as usual, includes audio so click through to the YouTube video to hear it.
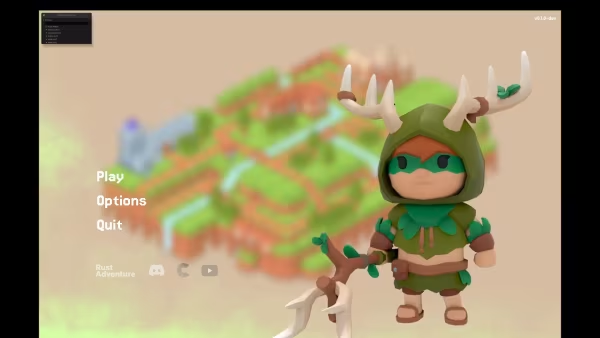
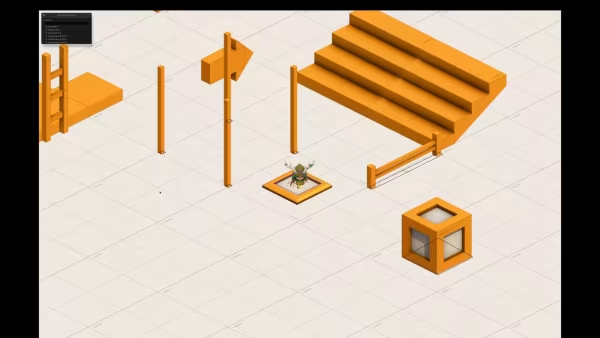
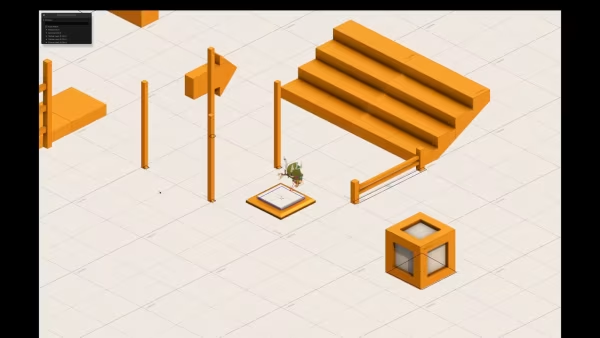
Isometric game blockout
showcase
This demo mimics the Hades 1 start menu using regular bevy_ui. Starting the game shows 3d rendered 8-directional character animations from Blender on top of an isometric level built with Tiled and integrated with bevy_ecs_tilemap
. Interaction in the world happens via sensors, colliders, and raycasting from bevy_xpbd_2d
. The world and character art are blockouts and will be replaced in the future.
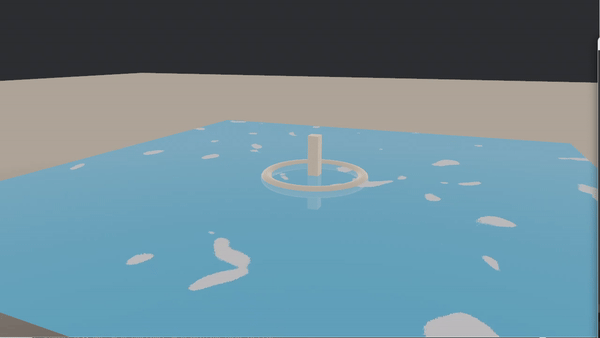
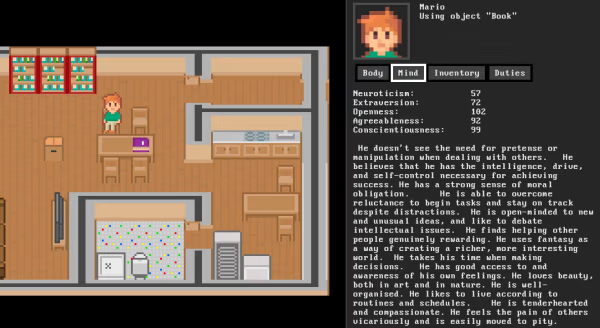
To Build a Home personality system
showcase
First version of the personality system of To Build a Home, based on the Big Five model of personality. Characters have five personality traits, subdivided in thirty sub-traits, that define their mood, decisions, wants, needs, etc. The text on the screenshot is generated dynamically based on the sub-traits of the character.
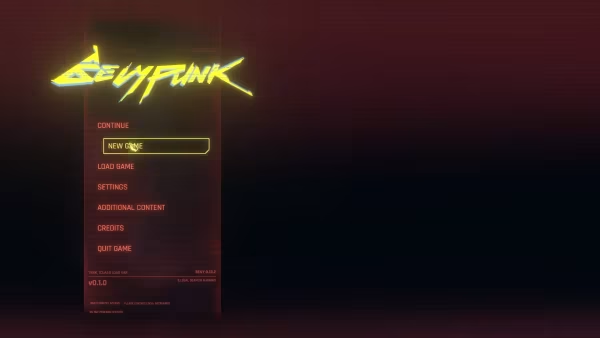
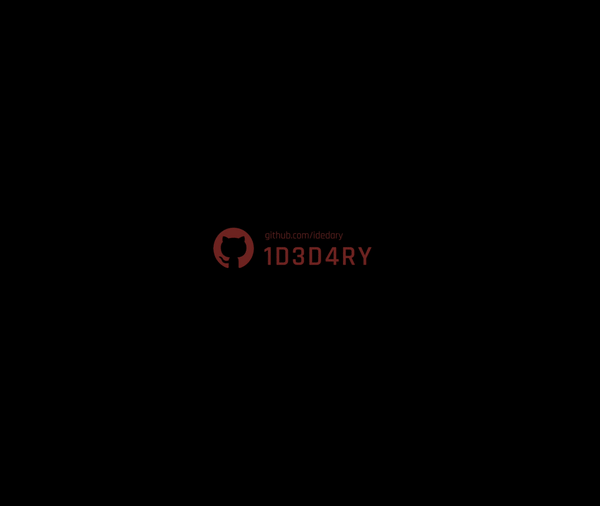
Bevypunk
showcase
bevypunk is a recreation of Cyberpunk UI in Bevy using bevy_lunex
: a path based retained layout engine.
This demo makes use of a brand new crate vleue_kinetoscope
which is responsible for loading and playing gifs.
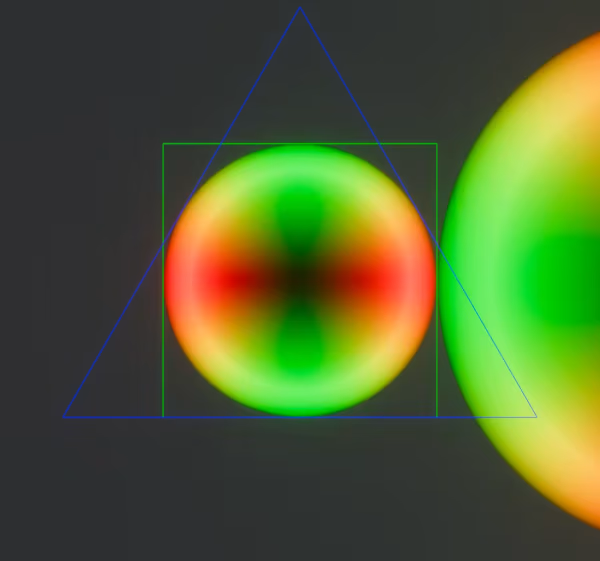
Infinitely scalable candy
showcase
An SDF fragment shader experiment. The code is fairly compact and more is included in the Discord thread
@fragment
fn fragment(mesh: VertexOutput)
-> @location(0) vec4<f32> {
let d = length(mesh.uv) - 0.5;
let a = abs(sin(d * 3.14159));
if (0.0 < d) {
discard;
} else {
return vec4f(
mesh.uv * mesh.uv * a * min(1/d * 1/d, 100.0),
0,
1
);
}
}
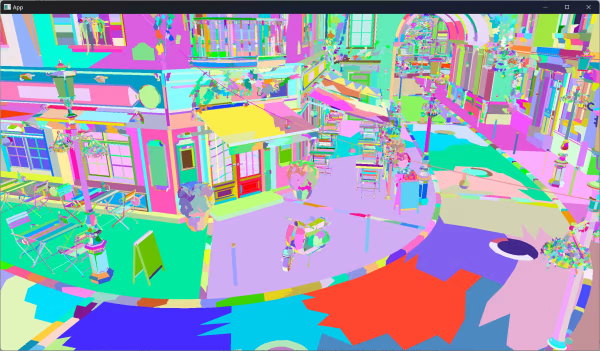
GLTF to MeshletMesh processing
showcase
Converting GLTF files, such as those exported from Blender, to Bevy MeshletMesh
s can make it easier to use the experimental meshlets feature. This demo is a demonstration of an early version of this processing work.
This demo is related to PR #13431
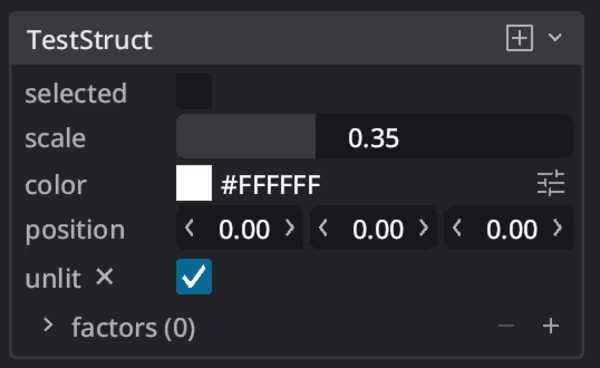
bevy_reactor min and max restrictions
showcase
bevy_reactor
's reflective property inspector widget now uses the new bevy_reflect
custom attributes feature to specify the min/max value for numeric fields, as well as the number of decimal digits shown and the step size. This enables building a slider widget for editing f32 fields. A new SpinBox widget can be used to edit Vec3 fields. Spinboxes are used when a numeric field has no min/max constraint, or where there isn't enough space to put a slider.
Available macro attributes include @ValueRange
, @Precision
, and @Step
with more to come and there's lots more information in the Discord thread.
#[derive(Resource, Debug, Reflect, Clone, Default)]
pub struct TestStruct {
pub selected: bool,
#[reflect(@ValueRange::<f32>(0.0..1.0))]
pub scale: f32,
pub color: Srgba,
pub position: Vec3,
pub unlit: Option<bool>,
#[reflect(@ValueRange::<f32>(0.0..10.0))]
pub roughness: Option<f32>,
#[reflect(@Precision(2))]
pub metalness: Option<f32>,
#[reflect(@ValueRange::<f32>(0.0..1000.0))]
pub factors: Vec<f32>,
}
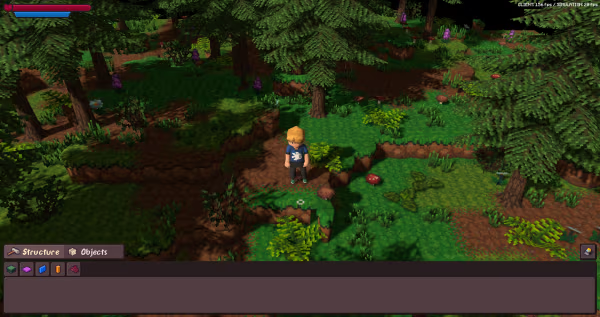
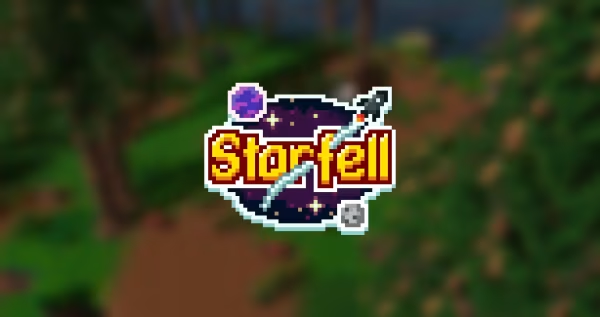
Starfell
showcase
Starfell is a game where you build your own community on a tiny planet. You will be able to construct houses, collect resources, interact with NPCs, and take on enemies and big bosses.
This update redesigns the pine trees, improves various aspects of foliage, and invests in some infrastructure and refactoring.
There's an older movement demo on YouTube.
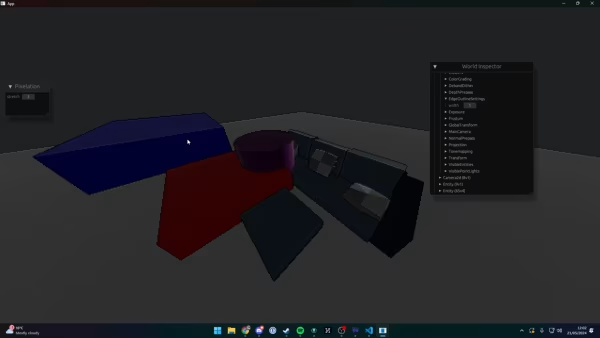
3d pixel art
showcase
3d pixel art generated by using a post-process shader to draw outlines and rendering at a low resolution then scaling up.
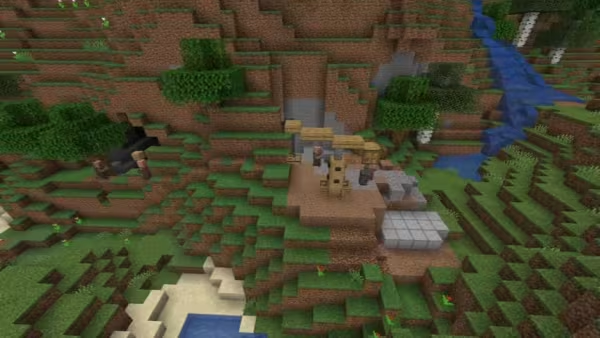
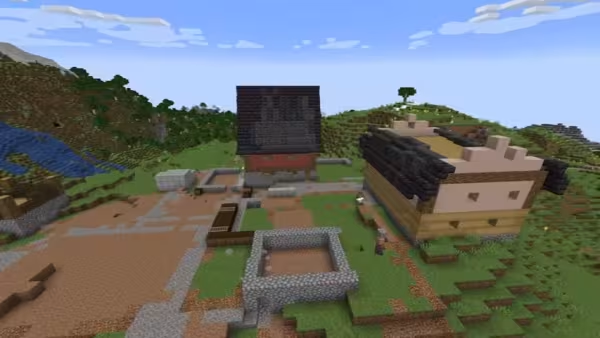
GenDesignMC 2024 Minecraft simulation in Bevy
showcase
This demo is simulating a Minecraft settlement in Bevy and then rendering it in Minecraft for GenDesignMC 2024.
As the simulation runs, each tick's changes get written to NBT, and at the end a datapack is generated that reads the NBT and replays it.
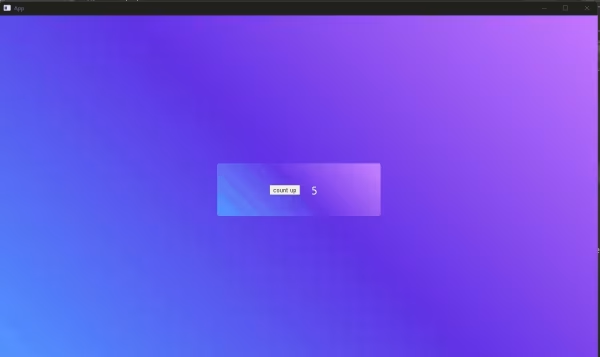
bevy_flurx and wry
showcase
bevy_flurx provides a mechanism for more sequential description of delays, character movement, waiting for user input, and other state waits.
wry is the cross-platform WebView rendering library used by Tauri.
This demo (and associated GitHub repo) is a simple prototype integrating Bevy and Wry using bevy_flurx
.
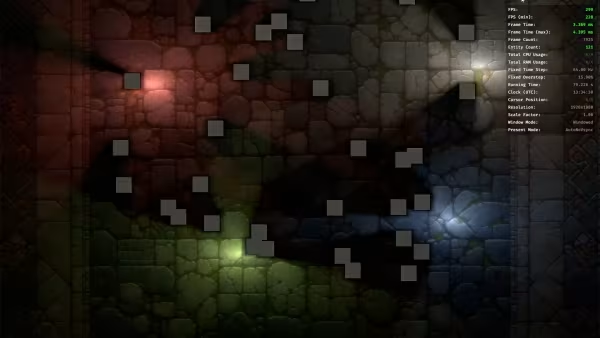
Crates
New releases to crates.io and substantial updates to existing projects
pyri_state v0.1.0
crate_release
Bevy States are getting ComputedStates
and Substates
in Bevy 0.14 but you can still use any alternative state implementation, like this crate: pyri_state
which has its own take on state management, including computed and sub states, and works with Bevy 0.13.
bevy_web_keepalive 0.1,0.2,0.3
crate_release
bevy_web_keepalive
is a collection of plugins that aim to help deal with the fact that web pages can stop running your Bevy app if the player backgrounds the game. It saw its 0.1, 0.2, and 0.3 releases this week.
bevy-histrion-packer v0.3.0
crate_release
bevy-histrion-packer
is a Bevy plugin that enables efficiently packing all game assets, such as textures, audio files, and other resources, into a single common PAK like file format.
0.3 updates the .hpak
file format to support multiple compression algorithms including deflate, gzip, zlib, and brotli.
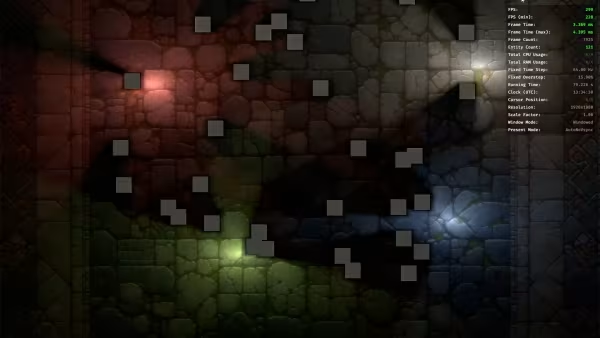
Devlogs
vlog style updates from long-term projects
Fixed incorrect behaviour of headless_renderer depending on image dimensions authored by bugsweeper
Remove another .view_layouts authored by IceSentry
Adds doc note that Timer and Stopwatch must be progressed manually authored by spooky-th-ghost
Fix lints on beta Rust authored by BD103
bevy_reflect: Custom attributes authored by MrGVSV
Deprecate dynamic plugins authored by BD103
Fix UI elements randomly not appearing after #13277. authored by pcwalton
Fix doc for Added, Changed authored by SpecificProtagonist
Add slerp function for Dir2, Dir3, Dir3A authored by bugsweeper
fix lava emissive strength in depth of field example authored by mockersf
Tetrahedron sampling authored by mweatherley
Inconsistent segments/resolution naming authored by solis-lumine-vorago
implement the full set of sort methods on QueryIter authored by Victoronz
Add more load_direct implementations authored by ricky26
Fix normals during mesh scaling authored by solis-lumine-vorago
Make render phases render world resources instead of components. authored by pcwalton
Rename `Rect` `inset()` method to `inflate()` authored by mnmaita
Annulus sampling authored by mweatherley
Optimize the values for `EMPTY` rect. authored by Olle-Lukowski
Tetrahedron mesh authored by mweatherley
Fix 2D looking blurry at odd window sizes authored by inodentry
Implement a SystemBuilder for building SystemParams authored by james-j-obrien
Further improve docs for component hooks authored by alice-i-cecile
Add `Distribution` access methods for `ShapeSample` trait authored by mweatherley
Make LoadContext use the builder pattern for loading dependent assets authored by ricky26
bevy_reflect: `enum_utility` cleanup authored by MrGVSV
simple Debug impls for query iterators authored by Victoronz
Mention of Vec normalization for Dir::new authored by bugsweeper
Add triangle_math tests and fix Triangle3d::bounding_sphere bug authored by aristaeus
Allow `AssetServer::load` to acquire a guard item. authored by mintlu8
Fix lighting example following emissive material changes in #13350 authored by jirisvd
New circular primitives: `Arc2d`, `CircularSector`, `CircularSegment` authored by aristaeus
Emissive is now LinearRgba on StandardMaterial authored by andristarr
Update render graph docs authored by cBournhonesque
add Debug for ptr types authored by cBournhonesque
Add meshing for `ConicalFrustum` authored by Jondolf
Add `Triangle3d` / `Tetrahedron` to render_primitives example authored by mweatherley
fix emissive value in StandardMaterial after swith to LinearRgba authored by mockersf
Want to contribute to Bevy?
Here you can find two types of potential contribution: Pull Requests that might need review and Issues that might need to be worked on.
Pull Requests Opened this week
Add App and SubApp resource methods authored by pietrosophya
implement the full set of sorts on QueryManyIter authored by Victoronz
Meshlet GLTF processor authored by JMS55
bevy_reflect: Reflection-based cloning authored by MrGVSV
constrain WorldQuery::init_state argument to ComponentInitializer authored by Victoronz
Remove SparseSets in Archetype/Table storage authored by cBournhonesque
Implement PBR anisotropy per `KHR_materials_anisotropy`. authored by pcwalton
add handling of all missing gltf extras: scene, mesh & materials authored by kaosat-dev
Added visibility bitmask as an alternative SSAO method authored by dragostis
Add a ComponentIndex and update QueryState creation/update to use it authored by cBournhonesque
Meshable extrusions authored by solis-lumine-vorago
add entities_all_unique to QueryManyIter authored by Victoronz
WIP: Try to normalise matrix naming authored by ricky26
improve texture slicing; support non-symmetrical slicing authored by JJJimbo1
fix: rename non send resources authored by pietrosophya
Implement percentage-closer soft shadows (PCSS). authored by pcwalton
Lower level `io` methods for `AssetServer`. authored by mintlu8
Avoid a panic when loading labelled assets authored by ricky26
Implement Rhombus 2D primitive. authored by salvadorcarvalhinho
Example for random sampling authored by mweatherley
Issues Opened this week
Gizmos primitives by ref authored by solis-lumine-vorago
Auto exposure breaks when object is too dark authored by GitGhillie
Very Large Material 2d Mesh diagonal artifact issue authored by miketwenty1
Bevy has too many rectangle / box types authored by alice-i-cecile
Move `bevy_math`'s implementations of `Reflect` into `bevy_math` authored by mweatherley
Document that zero-length reflection paths are allowed. authored by viridia
High performance sorted queries authored by iiYese
Add `World::resource_scope_by_id` authored by Shatur
Add additional names to GLTF entities for easier querying authored by MatrixDev
Image loading docs are not clear enough about required feature flags authored by cshenton-work
Squircle / Superellipse for UI / Gizmos / Mesh authored by Bcompartment
Bevy_ui outlines should respect BorderRadius. authored by viridia
Parent device is lost authored by theunrealtarik
Textures not displaying properly if normal/base texture transforms don't align authored by GitGhillie
`winit::TabLeft`/`Event::Suspended` doesn't function as intended on WASM authored by simbleau
Panic if changing browser zoom in WASM with an overridden scale factor authored by pietrosophya
Lack of Doc Comments in some Render Related Files authored by ua-kxie
Exit not correctly tearing down graphics stack?? authored by BobG1983
RenderAssetUsages::RENDER_WORLD assets are not cleaned when their handles are dropped authored by mockersf
Ui border is 1 pixel too large, or is getting aliased. authored by ImDanTheDev
Audio getting progressively worse. authored by redstonerti
Text styles should be inheritable authored by viridia